3种解决透视投影中视图空间位置恢复的方案
投影矩阵描述了场景中3D点到视口中2D点的映射关系。它将从视图(眼睛)空间转换为裁剪空间,而裁剪空间中的坐标会通过裁剪坐标的w分量除以得到归一化设备坐标(NDC)。NDC位于范围内(-1,-1,-1)到(1,1,1)。
在透视投影中,投影矩阵描述了从针孔相机所看到的世界中的3D点到视口中2D点的映射关系。
相机截锥体中的视图空间坐标被映射到一个立方体(归一化设备坐标)中。
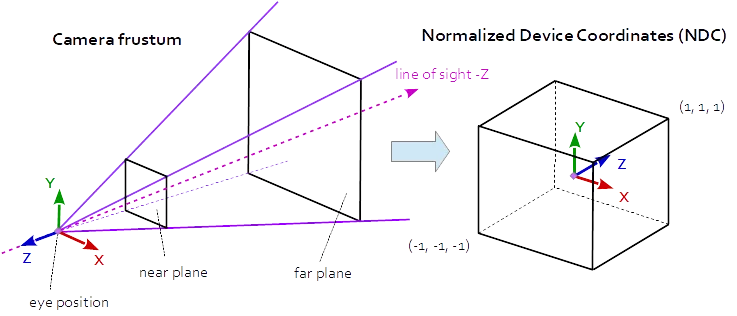
透视投影矩阵:
r = right, l = left, b = bottom, t = top, n = near, f = far
2*n/(r-l) 0 0 0
0 2*n/(t-b) 0 0
(r+l)/(r-l) (t+b)/(t-b) -(f+n)/(f-n) -1
0 0 -2*f*n/(f-n) 0
it follows:
aspect = w / h
tanFov = tan( fov_y * 0.5 );
prjMat[0][0] = 2*n/(r-l) = 1.0 / (tanFov * aspect)
prjMat[1][1] = 2*n/(t-b) = 1.0 / tanFov
在透视投影中,Z分量是通过有理函数计算得出的:
z_ndc = ( -z_eye * (f+n)/(f-n) - 2*f*n/(f-n) ) / -z_eye
深度(gl_FragCoord.z
和gl_FragDepth
)的计算如下:
z_ndc = clip_space_pos.z / clip_space_pos.w;
depth = (((farZ-nearZ) * z_ndc) + nearZ + farZ) / 2.0;
1. 视野和纵横比
由于投影矩阵是由视野和纵横比定义的,因此可以通过视野和纵横比来恢复视口位置。前提是它是对称透视投影,并且已知归一化设备坐标、深度和近远平面。
恢复视图空间中的Z距离:
z_ndc = 2.0 * depth - 1.0;
z_eye = 2.0 * n * f / (f + n - z_ndc * (f - n));
通过XY归一化设备坐标恢复视图空间位置:
ndc_x, ndc_y = xy normalized device coordinates in range from (-1, -1) to (1, 1):
viewPos.x = z_eye * ndc_x * aspect * tanFov;
viewPos.y = z_eye * ndc_y * tanFov;
viewPos.z = -z_eye;
2. 投影矩阵
投影矩阵中存储了由视野和长宽比定义的投影参数。因此,可以通过对称透视投影的投影矩阵值来恢复视口位置。
请注意投影矩阵、视野和长宽比之间的关系:
prjMat[0][0] = 2*n/(r-l) = 1.0 / (tanFov * aspect);
prjMat[1][1] = 2*n/(t-b) = 1.0 / tanFov;
prjMat[2][2] = -(f+n)/(f-n)
prjMat[3][2] = -2*f*n/(f-n)
在视图空间中恢复Z距离:
A = prj_mat[2][2];
B = prj_mat[3][2];
z_ndc = 2.0 * depth - 1.0;
z_eye = B / (A + z_ndc);
通过XY归一化设备坐标恢复视图空间位置:
viewPos.x = z_eye * ndc_x / prjMat[0][0];
viewPos.y = z_eye * ndc_y / prjMat[1][1];
viewPos.z = -z_eye;
3. 逆投影矩阵
当然,视口位置可以通过逆投影矩阵得到。
mat4 inversePrjMat = inverse( prjMat );
vec4 viewPosH = inversePrjMat * vec3( ndc_x, ndc_y, 2.0 * depth - 1.0, 1.0 )
vec3 viewPos = viewPos.xyz / viewPos.w;
请参考以下问题的答案: