我复制了您提供的代码,只是在最后面添加了4行代码。
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
import numpy as np
plt.close()
fig = plt.figure(figsize=(5, 2.5))
ax = fig.add_subplot(projection='3d')
ax.get_proj = lambda: np.dot(Axes3D.get_proj(ax), np.diag([1, 1, 1, 0.5]))
u = v = np.linspace(0, 2 * np.pi, 50)
u, v = np.meshgrid(u, v)
X = np.cos(v) * (6 - (5/4 + np.sin(3 * u)) * np.sin(u - 3 * v))
Y = (6 - (5/4 + np.sin(3 * u)) * np.sin(u - 3 * v)) * np.sin(v)
Z = -np.cos(u - 3 * v) * (5/4 + np.sin(3 * u))
ax.plot_surface(X, Y, Z, rstride=1, cstride=1, color=[0.7] * 3, linewidth=0.25, edgecolor="k")
left, right = plt.xlim()
ax.set_zlim(left, right)
ax.set_ylim(left, right)
plt.tight_layout()
我注释掉了
ax.set_box_aspect
这行代码,因为它会报错。以上的输出结果是:
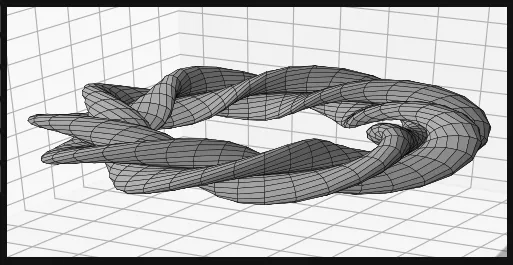
--- 编辑 ---
我有一个解决办法可以让它在matplotlib v3.4.2中正常工作:
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
import numpy as np
import os
plt.close()
fig = plt.figure(figsize=(5,5))
ax = fig.add_subplot(projection='3d')
u = v = np.linspace(0, 2 * np.pi, 50)
u, v = np.meshgrid(u, v)
X = np.cos(v) * (6 - (5/4 + np.sin(3 * u)) * np.sin(u - 3 * v))
Y = (6 - (5/4 + np.sin(3 * u)) * np.sin(u - 3 * v)) * np.sin(v)
Z = -np.cos(u - 3 * v) * (5/4 + np.sin(3 * u))
ax.plot_surface(X, Y, Z, rstride=1, cstride=1, color=[0.7] * 3, linewidth=0.25, edgecolor="k")
left, right = plt.xlim()
ax.set_zlim(left, right)
ax.set_ylim(left, right)
ax.dist = 6
fig.patch.set_alpha(0)
ax.patch.set_alpha(0)
ax.axis('off')
plt.tight_layout()
plt.savefig('plotted.png')
ax.remove()
fig = matplotlib.pyplot.gcf()
fig.set_size_inches(5, 2.5)
ax_bg = fig.add_subplot(111, projection='3d')
ax_bg.dist = 3
ax = fig.add_subplot(111)
fig.patch.set_alpha(1)
fig.subplots_adjust(left=0, bottom=0, right=1, top=1, wspace=0, hspace=0)
im = plt.imread('plotted.png')
h, w, dc = im.shape
im_cropped = im[120:390, :, :]
ax.axis('off')
ax.imshow(im_cropped)
os.remove('plotted.png')
输出结果为:
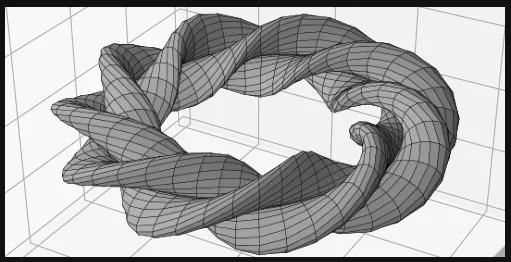
我不知道它是否适用于您的特定情况,但它可以满足您的问题。
如果有不清楚的地方,请让我知道。