我正在使用numpy和scipy做一个项目,需要填充nan值。目前我使用scipy.interpolate.rbf,但它会导致python崩溃得如此严重,即使try/except也无法保存。然而,运行几次后,似乎在周围全部是nan的情况下中间有数据时可能会不断失败,就像一个孤岛一样。有没有更好的解决方案可以避免这种频繁崩溃?
顺便说一句,我需要外推的数据量很大。有时候甚至达到了图像的一半(70x70,灰度),但它并不需要完美。它是图像拼接程序的一部分,只要与实际数据相似,就能工作。我尝试过最近邻来填充nans,但结果太不同了。
编辑:
总是似乎在失败的图像上。将该图像隔离出来后,它才能通过一次图像再次崩溃。
我至少使用的是NumPy 1.8.0和SciPy 0.13.2版本。
顺便说一句,我需要外推的数据量很大。有时候甚至达到了图像的一半(70x70,灰度),但它并不需要完美。它是图像拼接程序的一部分,只要与实际数据相似,就能工作。我尝试过最近邻来填充nans,但结果太不同了。
编辑:
总是似乎在失败的图像上。将该图像隔离出来后,它才能通过一次图像再次崩溃。
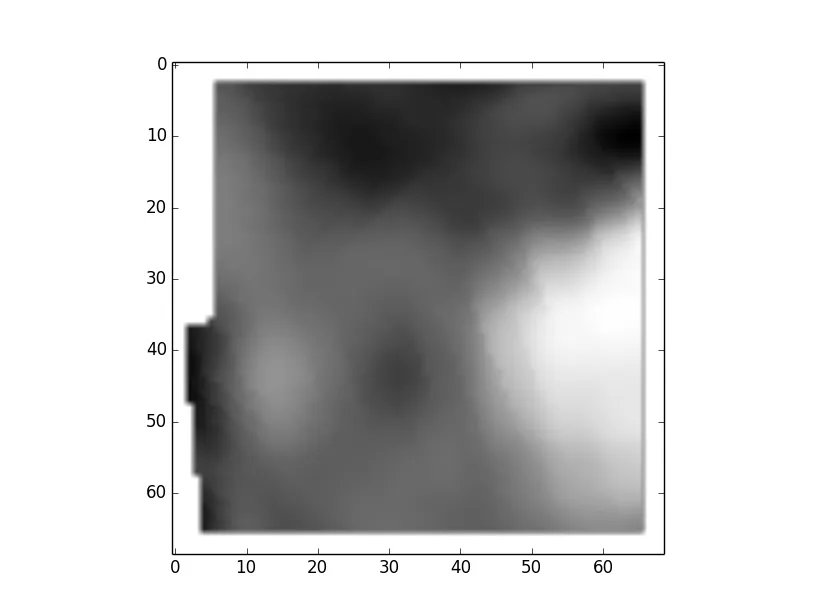