这个问题一开始对我来说似乎很简单,我的想法是使用blob分析来检测不同的斑点,按大小分组,并使用floodfill算法进行着色。
但是,我遇到了一些默认值的问题,这些默认值我没有修改,这浪费了一些时间。此外,我没有找到任何关于使用OpenCV进行洪水填充或给斑点着色的Python代码片段,而且针对SimpleBlobDetection的语法与旧版本相比发生了一些变化,我只能找到很少的文档和示例代码。所以,也许所有这些代码对其他用户也有用。
希望我正确地识别了您想要查找的部分。如果您不想包括大的黑色外叶,可以注释掉一行代码。
为了可视化,您可以调整图像的大小(目前已被注释,但请记得按4*4=16的系数相应调整大小阈值)。
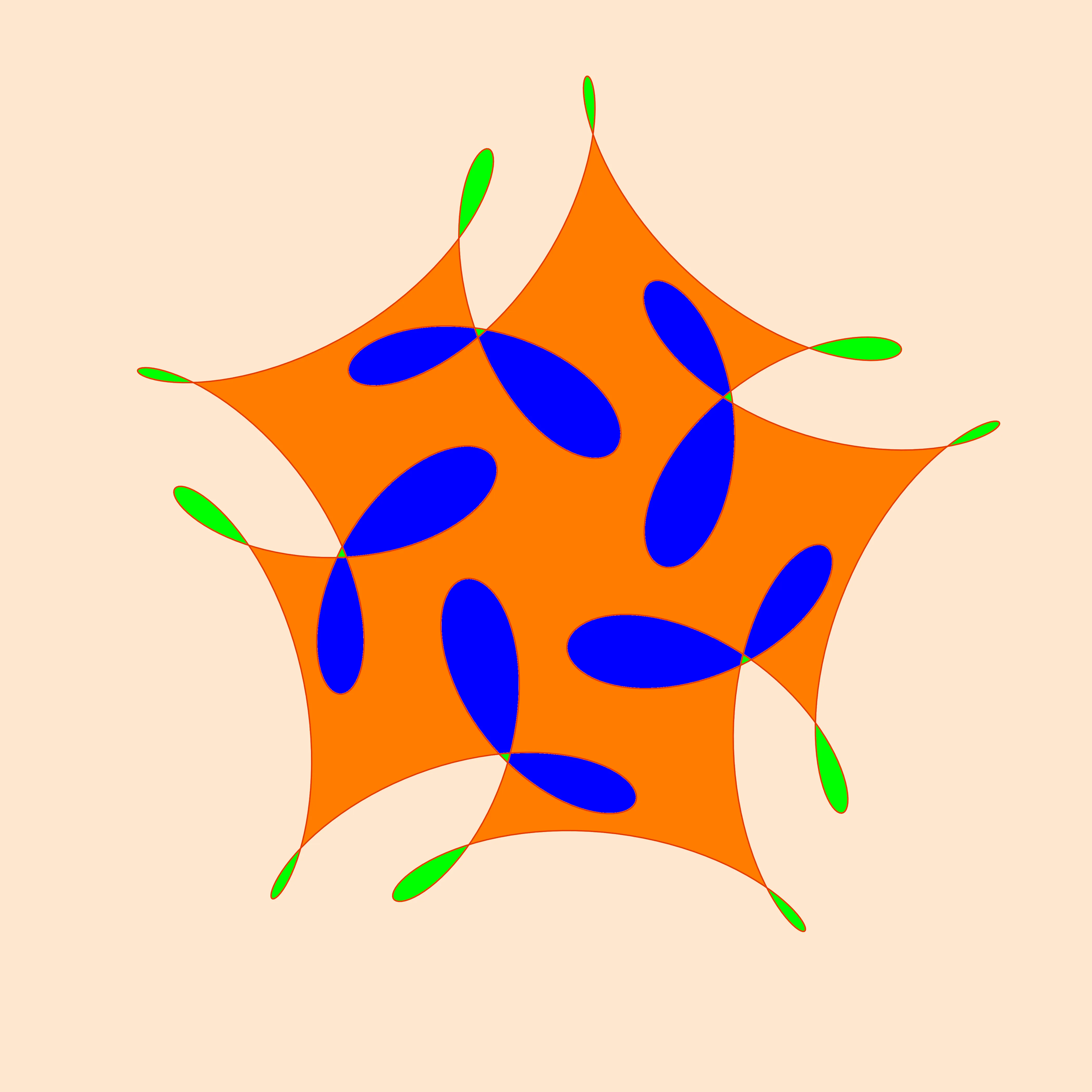
尽管这个代码有点长,但希望易于阅读。在解决这个问题时,我学到了很多与OpenCV相关的知识,谢谢!
顺便说一句,这张图片很棒。
import numpy as np
import cv2
im = cv2.imread('tricky.png')
imgray= cv2.cvtColor(im,cv2.COLOR_BGR2GRAY)
ret,imthresh = cv2.threshold(imgray,160, 255,type=cv2.THRESH_BINARY_INV)
params = cv2.SimpleBlobDetector_Params()
params.filterByArea = True
params.minArea = 15000
params.maxArea = 150000
detector = cv2.SimpleBlobDetector_create(params)
keypoints = detector.detect(imthresh)
im_with_keypoints = cv2.drawKeypoints(imthresh, keypoints, np.array([]), (0,0,255), cv2.DRAW_MATCHES_FLAGS_DRAW_RICH_KEYPOINTS)
cv2.imshow("Keypoints", im_with_keypoints)
maskborder=imgray.copy()
maskborder[:] = 0
bordersize=1
maskborder=cv2.copyMakeBorder(maskborder, top=bordersize, bottom=bordersize, left=bordersize, right=bordersize, borderType= cv2.BORDER_CONSTANT, value=[255,255,255] )
print imgray.shape[:2]
print maskborder.shape[:2]
result = im.copy()
for k in keypoints:
print int(k.pt[0]),int(k.pt[1])
seed_pt = int(k.pt[0]),int(k.pt[1])
cv2.floodFill(result, maskborder, seed_pt, (255,0, 0))
ret,imthresh2 = cv2.threshold(imgray,150, 255,type=cv2.THRESH_BINARY)
ret,imthresh3 = cv2.threshold(imgray,140, 255,type=cv2.THRESH_BINARY_INV)
imthresh4 = cv2.add(imthresh2,imthresh3)
params = cv2.SimpleBlobDetector_Params()
params.filterByArea = True
params.minArea = 20
params.maxArea = 1000
params.maxArea = 50000
params.filterByCircularity = False
params.filterByConvexity = False
params.filterByInertia = False
detector = cv2.SimpleBlobDetector_create(params)
keypoints = detector.detect(imthresh4)
im_with_keypoints2 = cv2.drawKeypoints(imthresh4, keypoints, np.array([]), (0,0,255), cv2.DRAW_MATCHES_FLAGS_DRAW_RICH_KEYPOINTS)
cv2.imshow("Keypoints2", im_with_keypoints2)
maskborder=imgray.copy()
maskborder[:] = 0
bordersize=1
maskborder=cv2.copyMakeBorder(maskborder, top=bordersize, bottom=bordersize, left=bordersize, right=bordersize, borderType= cv2.BORDER_CONSTANT, value=[255,255,255] )
print imgray.shape[:2]
print maskborder.shape[:2]
result2 = result.copy()
for k in keypoints:
print int(k.pt[0]),int(k.pt[1])
seed_pt = int(k.pt[0]),int(k.pt[1])
cv2.floodFill(result2, maskborder, seed_pt, (0,255, 0))
cv2.imshow("Result", result2)
cv2.imwrite("result.png",result2)
cv2.waitKey(0)
cv2.destroyAllWindows()