假设您有一个分割地图,每个对象都由唯一的索引标识,看起来类似于这样:
。对于每个对象,我想保存它覆盖的像素,但是到目前为止我只能想到标准的
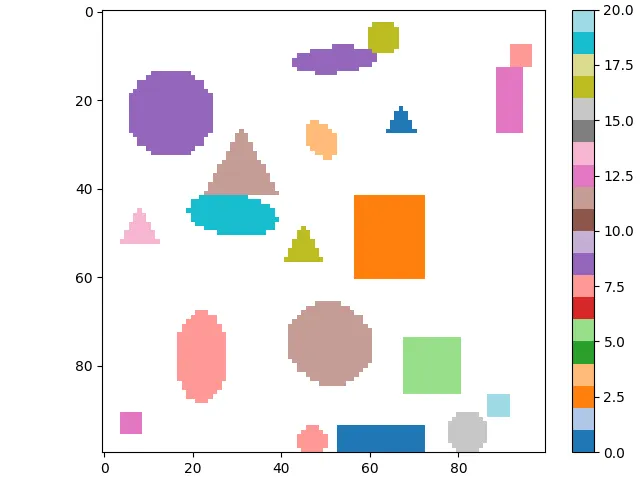
for
循环。不幸的是,对于具有数千个单独对象的大型图像,这种方法非常缓慢--至少对于我的真实数据是这样。我该如何加速处理呢?import numpy as np
import matplotlib as mpl
import matplotlib.pyplot as plt
from skimage.draw import random_shapes
# please ignore that this does not always produce 20 objects each with a
# unique color. it is simply a quick way to produce data that is similar to
# my problem that can also be visualized.
segmap, labels = random_shapes(
(100, 100), 20, min_size=6, max_size=20, multichannel=False,
intensity_range=(0, 20), num_trials=100,
)
segmap = np.ma.masked_where(segmap == 255, segmap)
object_idxs = np.unique(segmap)[:-1]
objects = np.empty(object_idxs.size, dtype=[('idx', 'i4'), ('pixels', 'O')])
# important bit here:
# this I can vectorize
objects['idx'] = object_idxs
# but this I cannot. and it takes forever.
for i in range(object_idxs.size):
objects[i]['pixels'] = np.where(segmap == i)
# just plotting here
fig, ax = plt.subplots(constrained_layout=True)
image = ax.imshow(
segmap, cmap='tab20', norm=mpl.colors.Normalize(vmin=0, vmax=20)
)
fig.colorbar(image)
fig.show()
for
循环,直接使用objects ['pixels'] = indicesPerObject
吗? - mapf