这是一张完美的样本图片,包含了各种黑色物体。
而这段代码应该可以找到所有的黑色植物。
但结果令人失望,因为这
包含了118个轮廓。请注意,我需要找到并取出14个物体。当轮廓实际上不正确时,如何切割每个植物?或者至少将非常接近的轮廓连接到每个更大的轮廓,以单独保存对象?谢谢。
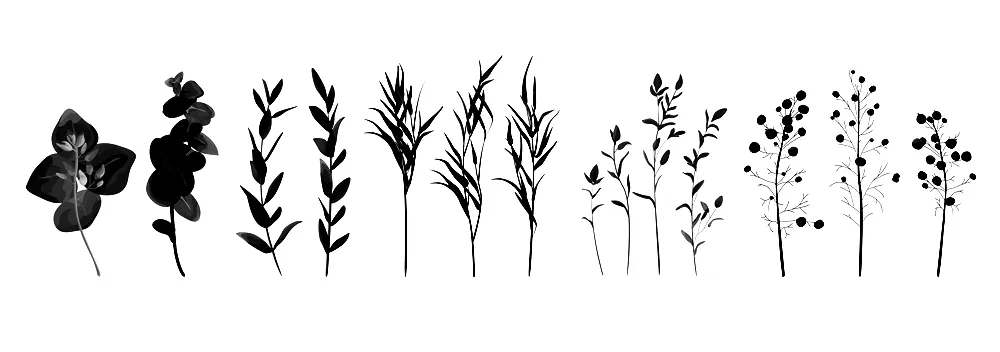
import cv2 as cv
import numpy as np
img = cv.imread("12.jpg")
tresh_min= 200
tresh_max=255
gray = cv.cvtColor(img, cv.COLOR_BGR2GRAY)
blurred = cv.GaussianBlur(gray, (5, 5), 0)
_, threshold = cv.threshold(blurred, tresh_min, tresh_max, 0)
(contours, _)= cv.findContours(threshold, cv.RETR_TREE, cv.CHAIN_APPROX_SIMPLE)
print(f'Number of countours: {len(contours)}')
mask = np.ones(img.shape[:2], dtype="uint8") * 255
# Draw the contours on the mask
cv.drawContours(mask, contours=contours, contourIdx=-1, color=(0, 255, 255), thickness=2)
但结果令人失望,因为这
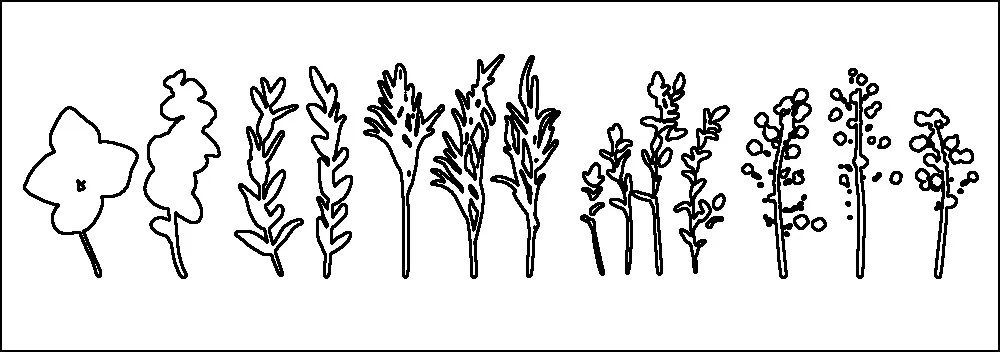