我正在尝试在屏幕上随机位置画x个不相交的圆。
我的期望结果:在屏幕上绘制x个圆到随机位置,如我在paint中绘制的美丽图表所示:
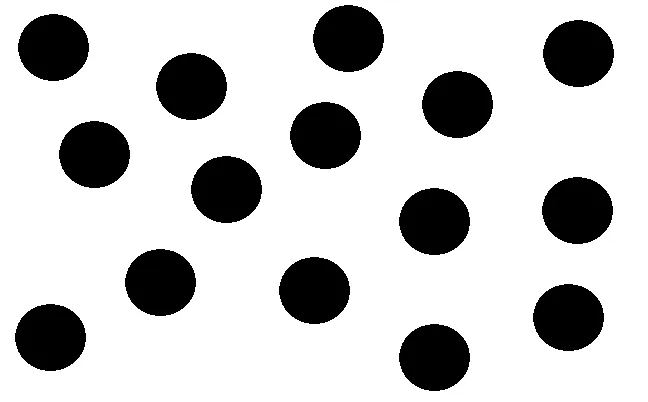
实际结果:每次程序循环时,在新的随机位置只画了一个圆。
我尝试过的代码:
import pygame
import random
def draw_food(screen,color,x,y,radius):
'''This function draws/creates the food sprite, The screen variable tells the food what screen to be drawn to. the color variable sets the color the radius variable sets how large of a circle the food will be.'''
pygame.draw.circle(screen,green,(x,y),radius)
pygame.init()
width = 800
height = 600
screen = pygame.display.set_mode((width,height))
white = (255, 255, 255)
green = (0, 255, 0)
running = True
# main program loop
while running:
# Did the user click the window close button?
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Fill the background with white
screen.fill((white))
# Draw the food
draw_food(screen,green,random.randint(0,width),random.randint(0,height),20)
# Flip the display
pygame.display.flip()
我查看了其他类似的问题,但我能找到的所有问题都是针对不同的编程语言或者与本题无关。同时我也是 Python 和 Pygame 的初学者。