将绘图区域拆分成单独的小区域
我们按以下步骤构建一个函数:
- 通过遍历对象的结构,获取用于分面的变量名称(在这里是
'z'
)。
- 我们用空的
ggplot
对象中的facet
元素覆盖绘图对象的facet
元素(因此如果我们在此阶段打印它,则分面将被删除)。
- 我们提取数据并沿着我们在第1步中确定的变量进行拆分。
- 我们用每个子集(这里是
12
次)覆盖原始数据并将所有输出存储在列表中。
代码:
splitFacet <- function(x){
facet_vars <- names(x$facet$params$facets)
x$facet <- ggplot2::ggplot()$facet
datasets <- split(x$data, x$data[facet_vars])
new_plots <- lapply(datasets,function(new_data) {
x$data <- new_data
x})
}
new_plots <- splitFacet(myplot)
length(new_plots)
new_plots[[3]]
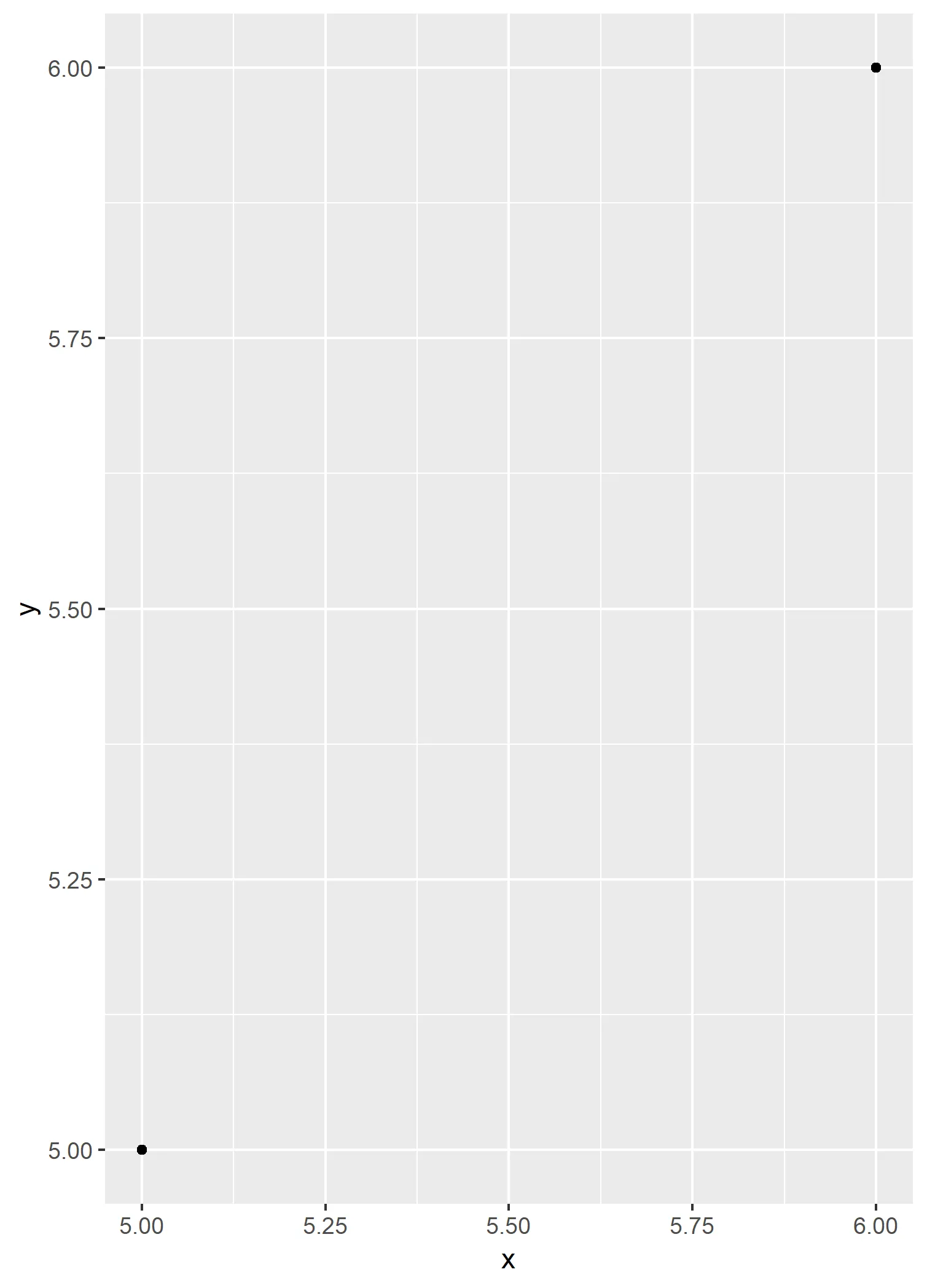
将图案分割成多个子图,每个子图最多有n个
如果我们想保留这些子图,但想要更少的子图,可以跳过第2步,重新调整我们的分割方式,使其包括用于分面的多个变量值。
我们不需要创建一个单独的函数,而是将第1个函数通用化,其中n
表示每个图中的子图数量。
n = NULL
表示获得先前输出的结果,这与n = 1
(每个图中仅包含一个子图)稍有不同。
splitFacet <- function(x, n = NULL){
facet_vars <- names(x$facet$params$facets)
if(is.null(n)){
x$facet <- ggplot2::ggplot()$facet
datasets <- split(x$data, x$data[facet_vars])
} else {
inter0 <- interaction(x$data[facet_vars], drop = TRUE)
inter <- ceiling(as.numeric(inter0)/n)
datasets <- split(x$data, inter)
}
new_plots <- lapply(datasets,function(new_data) {
x$data <- new_data
x})
}
new_plots2 <- splitFacet(myplot,4)
length(new_plots2)
new_plots2[[2]]

这也可能会很有用:
unfacet <- function(x){
x$facet <- ggplot2::ggplot()$facet
x
}
整洁的方式
如果代码可用,就不需要费这么大劲了,我们可以在将数据提供给 ggplot
之前对其进行拆分:
library(tidyverse)
myplots3 <-
df %>%
split(ceiling(group_indices(.,z)/n_facets)) %>%
map(~ggplot(.,aes(x =x, y=y))+geom_point()+facet_wrap(~z))
myplots3[[3]]

myplots <- lapply(unique(df$z), function(id) ggplot(subset(df, z == id), aes(x=x, y=y))+geom_point() + ylim(range(df$y)) + xlim(range(df$x)) ); do.call(gridExtra::grid.arrange, myplots[3:6])
这样。 - lukeAfacet_wrap(~z)
。 - eipi10