我猜你需要的几乎就是以下内容:
我想这几乎是你所需要的:
fun showSortDialog(context: Activity) {
val options = arrayOf(
"Relevance",
"Price - Low to High",
"Price - High to Low",
"Newest"
)
val builder = AlertDialog.Builder(context, R.style.MultiChoiceAlertDialog)
val view = context.layoutInflater.inflate(R.layout.dialog_custom, null, false)
val radioGroup = view.findViewById<RadioGroup>(R.id.radiogroup)
val purpleColor = ContextCompat.getColor(context, R.color.purple)
val radioStyle = ContextThemeWrapper(radioGroup.context, R.style.MyRadioButton)
for (option in options) {
val radioButton = RadioButton(radioStyle)
radioButton.setText(option)
radioGroup.addView(radioButton)
}
radioGroup.setOnCheckedChangeListener { group, checkedId ->
for (child in radioGroup.children) {
child as RadioButton
if (child.id == checkedId) {
child.setTextColor(purpleColor)
} else {
child.setTextColor(Color.BLACK)
}
}
}
builder.setView(view)
builder.show()
}
样式:
<style name="YourAlertDialog.Button" parent="Widget.MaterialComponents.Button.TextButton">
<item name="android:textColor">@color/colorPrimary</item>
<item name="android:textSize">20sp</item>
<item name="android:textAllCaps">false</item>
<item name="android:gravity">left</item>
<item name="android:letterSpacing">0</item>
</style>
<style name="MultiChoiceAlertDialog" parent="Theme.MaterialComponents.Light.Dialog.Alert">
<item name="buttonBarPositiveButtonStyle">@style/YourAlertDialog.Button</item>
<item name="buttonBarNegativeButtonStyle">@style/YourAlertDialog.Button</item>
<item name="buttonBarNeutralButtonStyle">@style/YourAlertDialog.Button</item>
<item name="android:background">#fff</item>
<item name="android:textColorPrimary">#000</item>
<item name="android:textColor">@drawable/selector_custom</item>
<item name="android:colorActivatedHighlight">#0f0</item>
<item name="android:colorControlActivated">#00f</item>
<item name="colorControlNormal">@color/gray</item>
<item name="colorControlActivated">@color/purple</item>
<item name="dialogCornerRadius">8dp</item>
</style>
<style name="MyRadioButton" parent="Theme.AppCompat.Light">
<item name="colorControlNormal">@color/gray</item>
<item name="colorControlActivated">@color/purple</item>
</style>
以及自定义布局(dialog_custom.xml):
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:orientation="vertical">
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:text="Sort by"
android:textColor="#000"
android:textSize="20sp" />
<RadioGroup
android:id="@+id/radiogroup"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</RadioGroup>
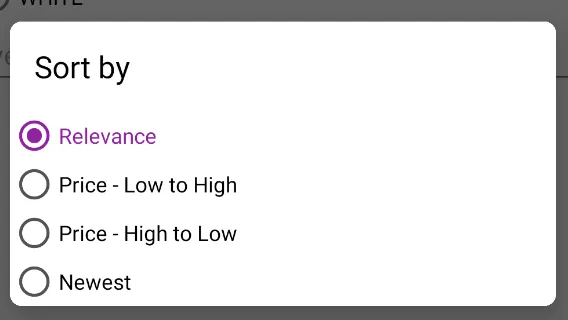