Python和Java之间的进程间通信
在Python应用程序中实现一个自包含、系统无关的JRE。
为了使Python应用程序独立于整个系统,必须在Python应用程序内部创建一个JRE。如果希望应用程序不依赖于系统,并且所有内容都包含在应用程序中,则必须执行此操作。如果整个应用程序必须准备好在任何用户的计算机上运行而无需进行任何其他资源和安装程序,则这非常有帮助。
前往
https://learn.microsoft.com/en-us/java/openjdk/download,下载所需硬件架构和操作系统的JDK,并将其加载到Python应用程序的环境中。然后将Java应用程序项目作为整体或从Java应用程序制作的
.jar
文件放置在Python应用程序的目录中。
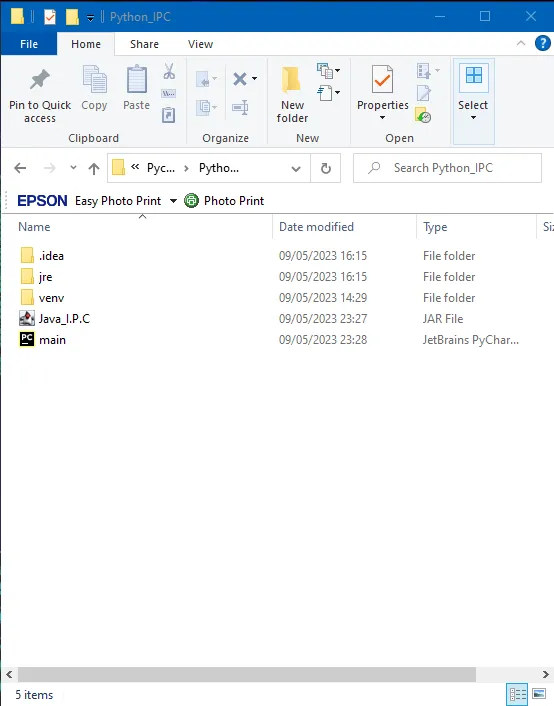
您可以在 Python 应用程序中启动 Java 应用程序作为子进程。
进程间通信方法
使用 stdin、stout 和 stderr 进行实时进程间通信
stdin
、stdout
和 stderr
流是操作系统内核用于应用程序和进程的主要 I/O 系统。通过修改这些流从子进程到操作系统,再到子进程到父进程的流动,这些流将被绕过父进程,并使父进程能够直接与子进程执行输入和输出操作。
[ Java 代码 ]
public class Main {
public static void main(String[] args)
{
System.out.println("Message from Java application");
System.out.flush();
Scanner s = new Scanner(System.in);
String input = s.nextLine();
s.close();
System.out.println(input);
System.out.flush();
}
}
[ Python 代码 ]
import subprocess
path_to_java_exe = "C:\\Users\\Teodor Mihail\\PycharmProjects\\Python_IPC\\jre\\bin\\java.exe"
java_exe_arguments = "-jar"
java_jar_file_path = "C:\\Users\\Teodor Mihail\\PycharmProjects\\Python_IPC\\Java_I.P.C.jar"
process = None
def subprocess_startup():
global process
process = subprocess.Popen([path_to_java_exe, java_exe_arguments, java_jar_file_path], stdin=subprocess.PIPE,
stdout=subprocess.PIPE, stderr=subprocess.PIPE)
def subprocess_read_stdout():
received = process.stdout.readline()
print(received)
def subprocess_write_stdin(data):
process.stdin.write(data.encode("utf-8"))
process.stdin.flush()
def main():
subprocess_startup()
subprocess_read_stdout()
subprocess_write_stdin("Message from Python application\n")
subprocess_read_stdout()
if __name__ == '__main__':
main()
在每次调用
process.stdin.write
方法时,发送到子进程的数据必须以换行符("
\n
")结尾。这是因为Java扫描器将扫描
stdin
流以查找换行符,并且由于流被缓冲,这将导致在执行此操作的线程上出现锁定。