背景:我正在尝试创建一个用于三个矩阵相乘的行为文件。我试图通过首先查看是否可以读取输入矩阵,然后输出中间矩阵来调试它。
行为文件:
作为我的模拟图中所示,我获得了一些值,但是信号变成了一些奇怪的大值。
我有一些疑问,din(0), din(1), din(2)...din(n) 是否与 inputblock(0,0),inputblock(0,1),inputblock(0,2) 等相对应。但是我仔细检查了我的行为文件,并没有发现任何问题。我的测试台设计有什么问题吗?
编辑:我需要在此输出结果。
我原以为这段代码与答案中的代码类似,但我遇到了完全相同的问题。如何解决?这是当前输出的图片。正确的值都在那里,只是被一个时钟周期向后移动了一个位置。
最终编辑:我自己解决了这个问题。问题出在循环边界上。
行为文件:
LIBRARY ieee;
USE ieee.std_logic_1164.ALL;
entity DCT_beh is
port (
Clk : in std_logic;
Start : in std_logic;
Din : in INTEGER;
Done : out std_logic;
Dout : out INTEGER
);
end DCT_beh;
architecture behavioral of DCT_beh is
begin
process
type RF is array ( 0 to 7, 0 to 7 ) of INTEGER;
variable i, j, k : INTEGER;
variable InBlock : RF;
variable COSBlock : RF;
variable TempBlock : RF;
variable OutBlock : RF;
variable A, B, P, Sum : INTEGER;
begin
COSBlock := (
( 125, 122, 115, 103, 88, 69, 47, 24 ),
( 125, 103, 47, -24, -88, -122, -115, -69 ),
( 125, 69, -47, -122, -88, 24, 115, 103 ),
( 125, 24, -115, -69, 88, 103, -47, -122 ),
( 125, -24, -115, 69, 88, -103, -47, 122 ),
( 125, -69, -47, 122, -88, -24, 115, -103 ),
( 125, -103, 47, 24, -88, 122, -115, 69 ),
( 125, -122, 115, -103, 88, -69, 47, -24 )
);
--Starting
wait until Start = '1';
Done <= '0';
--Read Input Data
for i in 0 to 7 loop
for j in 0 to 7 loop
wait until Clk = '1' and clk'event;
InBlock(i,j) := Din;
end loop;
end loop;
--TempBlock = COSBLOCK * InBlock
for i in 0 to 7 loop
for j in 0 to 7 loop
Sum := 0;
for k in 0 to 7 loop
A := COSBlock( i, k );
B := InBlock( k, j );
P := A * B;
Sum := Sum + P;
if( k = 7 ) then
TempBlock( i, j ) := Sum;
end if;
end loop;
end loop;
end loop;
--Finishing
wait until Clk = '1' and Clk'event;
Done <= '1';
--Output Data
for i in 0 to 7 loop
for j in 0 to 7 loop
wait until Clk = '1' and Clk'event;
Done <= '0';
Dout <= tempblock(i,j);
end loop;
end loop;
end process;
end behavioral;
测试台文件:
LIBRARY ieee;
USE ieee.std_logic_1164.ALL;
-- Uncomment the following library declaration if using
-- arithmetic functions with Signed or Unsigned values
--USE ieee.numeric_std.ALL;
ENTITY lab4b_tb IS
END lab4b_tb;
ARCHITECTURE behavior OF lab4b_tb IS
-- Component Declaration for the Unit Under Test (UUT)
COMPONENT DCT_beh
PORT(
Clk : IN std_logic;
Start : IN std_logic;
Din : IN INTEGER;
Done : OUT std_logic;
Dout : OUT INTEGER
);
END COMPONENT;
--Inputs
signal Clk : std_logic := '0';
signal Start : std_logic := '0';
signal Din : INTEGER;
--Outputs
signal Done : std_logic;
signal Dout : INTEGER;
-- Clock period definitions
constant Clk_period : time := 10 ns;
BEGIN
-- Instantiate the Unit Under Test (UUT)
uut: DCT_beh PORT MAP (
Clk => Clk,
Start => Start,
Din => Din,
Done => Done,
Dout => Dout
);
-- Clock process definitions
Clk_process :process
begin
Clk <= '0';
wait for Clk_period/2;
Clk <= '1';
wait for Clk_period/2;
end process;
-- Stimulus process
stim_proc: process
variable i, j : INTEGER;
variable cnt : INTEGER;
begin
-- hold reset state for 100 ns.
wait for 100 ns;
start <= '1';
wait for clk_period;
start <= '0';
for cnt in 0 to 63 loop
wait until clk = '1' and clk'event;
din <= cnt;
end loop;
--wait for 100 ns;
--start <= '1';
--wait for clk_period;
--start <= '0';
--for i in 0 to 63 loop
-- wait for clk_period;
--if (i < 24) then
--din <= 255;
--elsif (i > 40) then
--din <= 255;
--else
--din <= 0;
--end if;
--end loop;
wait;
end process;
END;
当start=1时,矩阵被读入inputblock。在这种情况下,矩阵仅填充了从0到63的唯一递增值。然后,当done=1时,输出乘积矩阵outblock。问题在于,在我的模拟中,我收到了一些应该在最终矩阵中但顺序不正确的值。例如,下面的行包含乘积矩阵tempblock中的第一行:
14464.000 15157.000 15850.000 16543.000 17236.000 17929.000 18622.000 19315.000
作为我的模拟图中所示,我获得了一些值,但是信号变成了一些奇怪的大值。
我有一些疑问,din(0), din(1), din(2)...din(n) 是否与 inputblock(0,0),inputblock(0,1),inputblock(0,2) 等相对应。但是我仔细检查了我的行为文件,并没有发现任何问题。我的测试台设计有什么问题吗?
编辑:我需要在此输出结果。
din<=0;
for i in 0 to 63 loop
wait until clk = '1' and clk'event;
if i = 0 then
Start <= '1','0' after clk_period;
end if;
if (i < 24) then
din <= 255;
elsif (i > 40) then
din <= 255;
else
din <= 0;
end if;
end loop;
我原以为这段代码与答案中的代码类似,但我遇到了完全相同的问题。如何解决?这是当前输出的图片。正确的值都在那里,只是被一个时钟周期向后移动了一个位置。
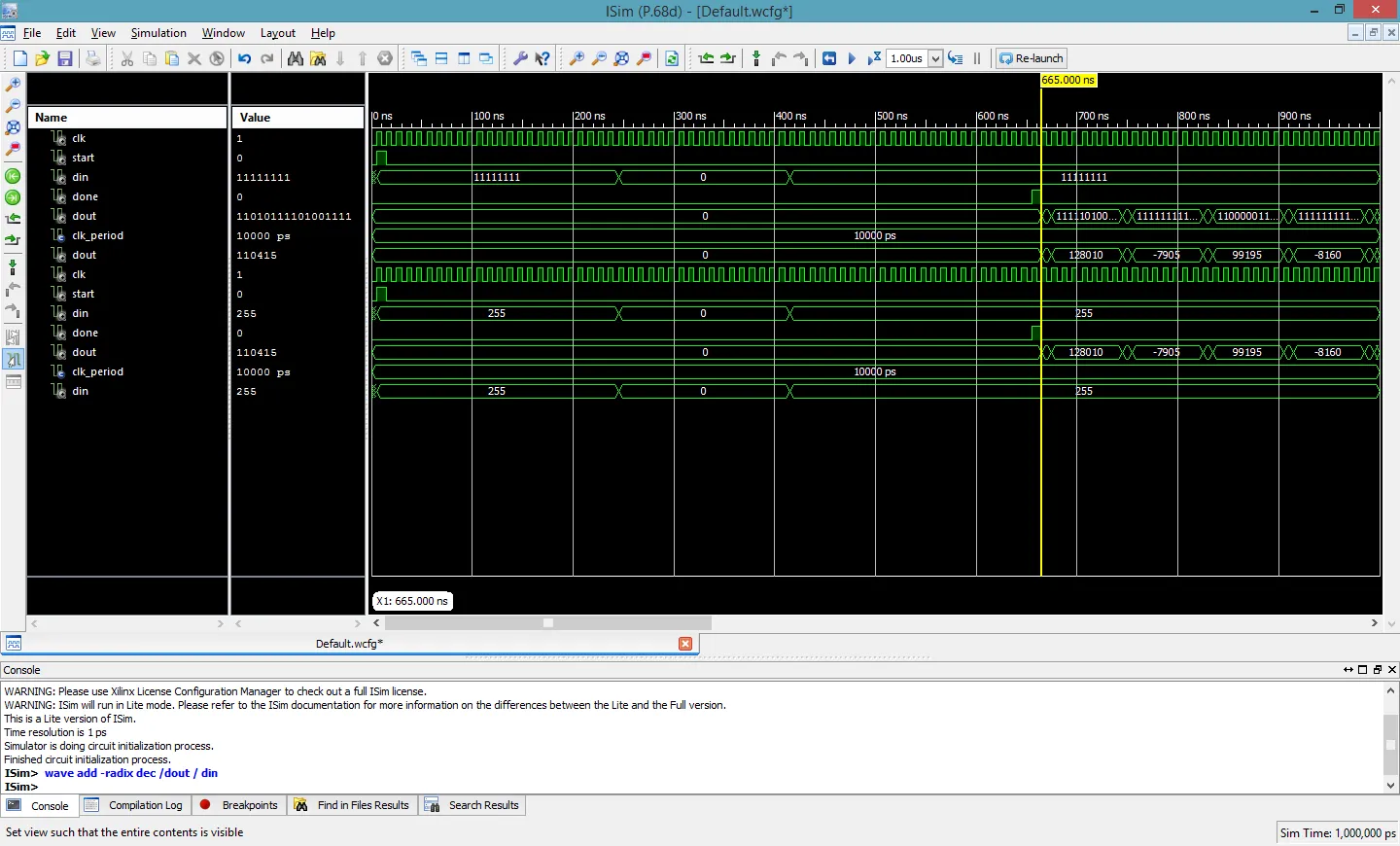