我想创建一个自定义的
它应该看起来像这样:
以下是我尝试创建此自定义
UIView
,例如TestLabelView
,其中包含一个标签,其边距为16px。它应该看起来像这样:
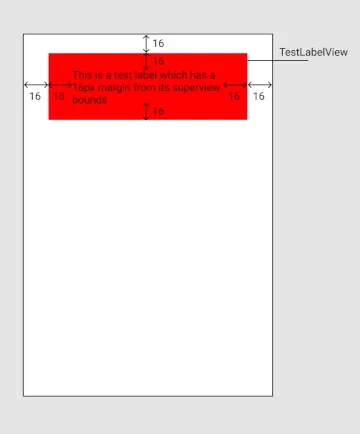
UIView
并在视图控制器中实例化它的方法:
TestLabelView
class TestLabelView: UIView {
private var label = UILabel()
var text: String = "" { didSet { updateUI() } }
override init(frame: CGRect) {
super.init(frame: frame)
internalInit()
}
required init?(coder: NSCoder) {
super.init(coder: coder)
internalInit()
}
private func internalInit() {
backgroundColor = .red
label.numberOfLines = 0
translatesAutoresizingMaskIntoConstraints = false
addSubview(label)
}
private func updateUI() {
label.text = text
NSLayoutConstraint(item: label,
attribute: .leading,
relatedBy: .equal,
toItem: self,
attribute: .leading,
multiplier: 1,
constant: 24).isActive = true
NSLayoutConstraint(item: self,
attribute: .trailing,
relatedBy: .equal,
toItem: label,
attribute: .trailing,
multiplier: 1,
constant: 24).isActive = true
NSLayoutConstraint(item: label,
attribute: .top,
relatedBy: .equal,
toItem: self,
attribute: .top,
multiplier: 1,
constant: 24).isActive = true
NSLayoutConstraint(item: self,
attribute: .bottom,
relatedBy: .equal,
toItem: label,
attribute: .bottom,
multiplier: 1,
constant: 24).isActive = true
setNeedsUpdateConstraints()
}
}
视图控制器
class ViewController: UIViewController {
@IBOutlet weak var testLabelView: TestLabelView?
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = .white
testLabelView?.text = "This is a test view with a test label, contained in multiple lines"
}
}
在故事板中
我设置了一个占位符内在大小来消除故事板错误,并且底部边距大于或等于24px,因此视图高度应根据内容大小设置
但是这样做后,我看不到标签出现。 我做错了什么?
感谢您的帮助
label.translatesAutoresizingMaskIntoConstraints = false
? - Shawn Frank