我一直在对200多张连续拍摄的太阳图片进行对齐,以便以后进行分析。
我以为我已经成功了,但是当我播放所有图片的视频时,我注意到太阳向下漂移。我认为这是因为在某些图片中,没有完整的太阳盘,因此我通过“质心”对齐它们的方式有些错误。
以下是我所说的漂移的一些示例:
我认为这种漂移是由于我如何找到每个圆盘的质心 - 由于每个FITS图像中都没有完整的圆盘,质心会慢慢移动到不同的位置,从而导致对齐出现偏斜。
我用的代码在这里:
我意识到只有当完整的圆圈存在时,这才有效 - 在我的一些图像中,太阳盘的部分被切断了。
我正在努力编辑我的函数,以便如果x轴或y轴中的一个较短(即如果圆圈的一部分被切断),我可以调整索引,使其好像完整的圆圈在那里 - 这样我的质心就真正成为圆圈的中心,我可以继续对齐。
提前感谢。
我以为我已经成功了,但是当我播放所有图片的视频时,我注意到太阳向下漂移。我认为这是因为在某些图片中,没有完整的太阳盘,因此我通过“质心”对齐它们的方式有些错误。
以下是我所说的漂移的一些示例:
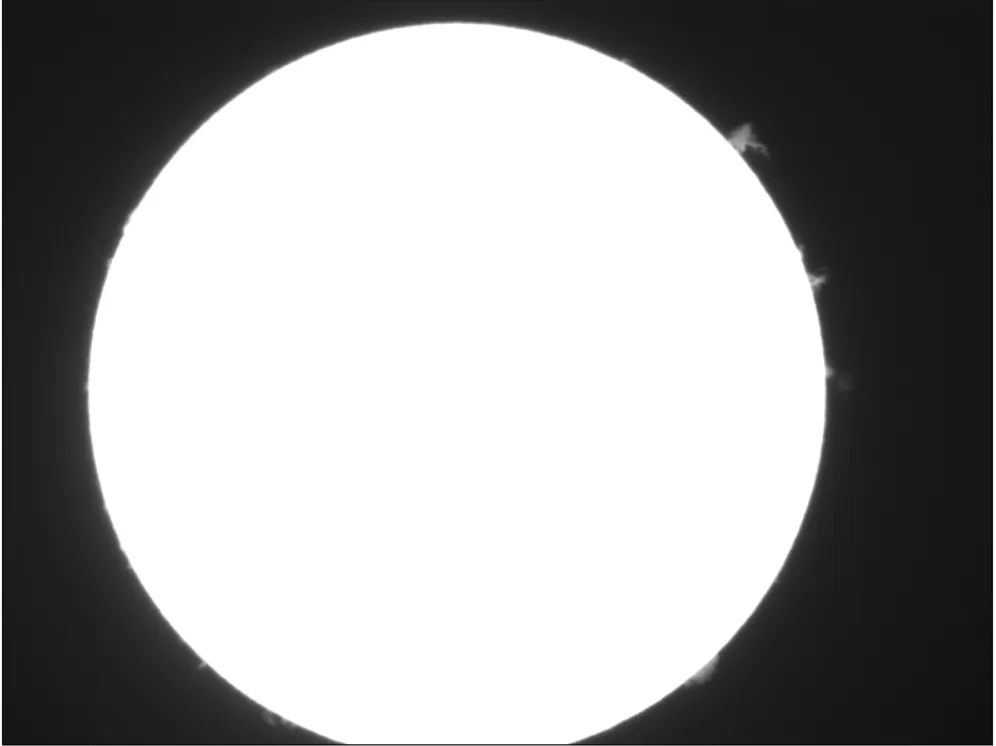
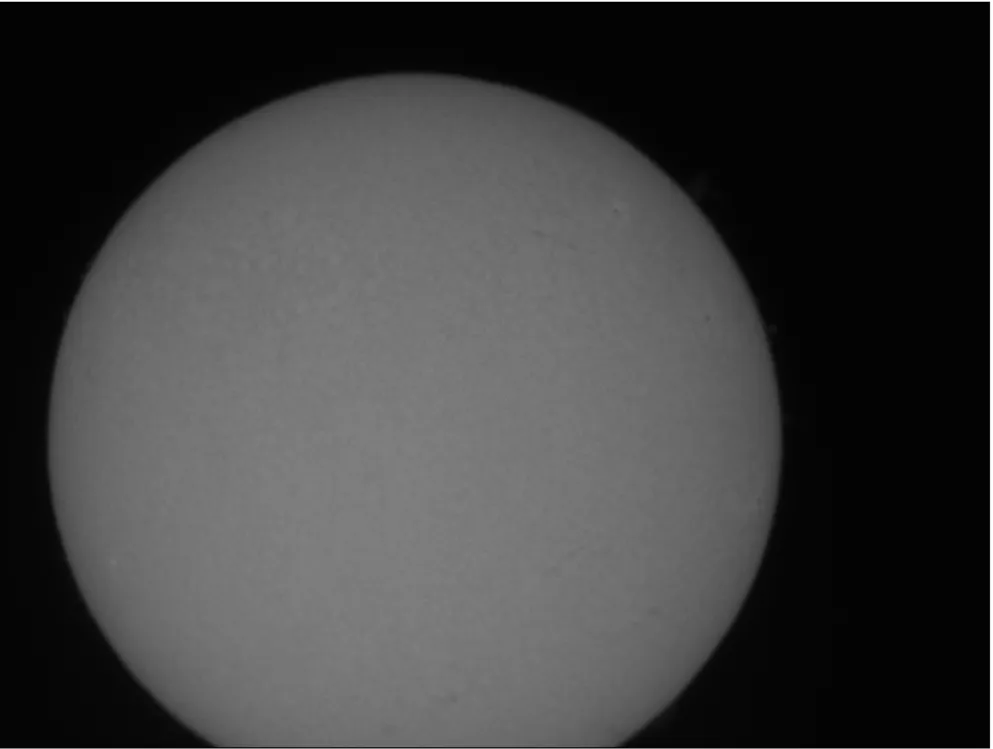
我用的代码在这里:
def get_centroid(data):
#data is extracted from a FITS file
#getting data centroid
y_index, x_index = np.where(data >= 1e4)
#threshold is set to only include bright circle (sun) and not background
#calculate the centroid
centroid_y = np.mean(y_index)
centroid_x = np.mean(x_index)
return(centroid_y, centroid_x)
我意识到只有当完整的圆圈存在时,这才有效 - 在我的一些图像中,太阳盘的部分被切断了。
我正在努力编辑我的函数,以便如果x轴或y轴中的一个较短(即如果圆圈的一部分被切断),我可以调整索引,使其好像完整的圆圈在那里 - 这样我的质心就真正成为圆圈的中心,我可以继续对齐。
提前感谢。