我不知道Q-table是什么,但我花了很多时间试图可视化不同的东西。
根据我对您问题的理解,您需要10个表格,我在下面的代码中将它们排列成2行5列的格子。也就是说,这段代码应该可以按您需要的任何数量进行扩展,希望如此。
我创建了一个字典,其中包含我认为可能出现在Q表格中的代表性值。希望我的假设足够接近,您可以使用以下代码来解决问题。
from matplotlib import pyplot as plt
import numpy as np
n_row = 2
n_col = 5
Q = {}
for m in range(n_row * n_col):
Q[(m, 'U')] = 2 * np.random.random() - 1
Q[(m, 'D')] = 2 * np.random.random() - 1
Q[(m, 'L')] = 2 * np.random.random() - 1
Q[(m, 'R')] = 2 * np.random.random() - 1
boxsize = 0.5
fontcol = 'k'
centerfontcol = [0.3, 0.3, 0.3]
fontsize = 4
maxalpha = 0.3
fig = plt.figure(figsize = (n_col * boxsize, n_row * boxsize))
ax = plt.axes([0, 0, 1, 1])
xspace = 0.2 / n_col
yspace = 0.15 / n_row
m = 0
for r in range(n_row):
y1 = 1 - (r + 1) / n_row
y2 = 1 - r / n_row
for c in range(n_col):
x1 = c / n_col
x2 = (c+1) / n_col
plt.plot([x1, x1, x2, x2, x1], [y1, y2, y2, y1, y1], 'k')
fillalpha = maxalpha * np.random.random()
plt.fill([x1, x1, x2, x2, x1], [y1, y2, y2, y1, y1], 'k', alpha = fillalpha)
plt.text((x1 + x2) / 2, (y1 + y2) / 2, "%i" % m,
color = centerfontcol, fontsize = fontsize, va = 'center', ha = 'center')
plt.text(x1 + xspace, (y1 + y2) / 2, "%.2f" % Q[(m, 'L')],
color = fontcol, fontsize = fontsize, va = 'center', ha = 'center')
plt.text(x2 - xspace, (y1 + y2) / 2, "%.2f " % Q[(m, 'R')],
color = fontcol, fontsize = fontsize, va = 'center', ha = 'center')
plt.text((x1 + x2) / 2, y2 - yspace, "%.2f" % Q[(m, 'U')],
color = fontcol, fontsize = fontsize, va = 'center', ha = 'center')
plt.text((x1 + x2) / 2, y1 + yspace, "%.2f" % Q[(m, 'D')],
color = fontcol, fontsize = fontsize, va = 'center', ha = 'center')
m += 1
ax.set_axis_off()
plt.savefig("q-table.png", bbox_inches = "tight")
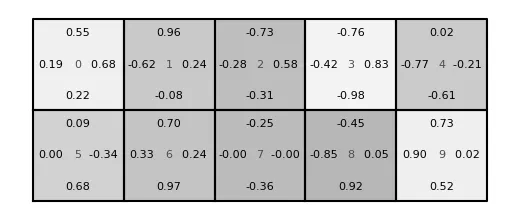
fill_color = None
),然后在函数中,如果fill_color
不是None
,则使用plt.fill(....., color = fill_color)
。 - ramzeekm
跟踪字典的索引。我只想着色来自holes
的索引框。我尝试过使用if (r*n_row+c*n_col) in holes
,但是错误的索引框不知何故被着色了。 - waffledood