如何将当前代码转换为类似于这个压力图的形式 - 矩形按弧形排列 - 然后突出显示最佳情况刻度以表示数值。
因此,我们将添加矩形并在创建骨架时更改角度。
我有一个演示,可以创建一系列矩形并更改角度 - 但我不确定如何稳定这个演示并从左侧创建弧形 - https://jsfiddle.net/smo6kqzw/3/
我目前有一个看起来像这样的拨号图表
因此,我们将添加矩形并在创建骨架时更改角度。
我有一个演示,可以创建一系列矩形并更改角度 - 但我不确定如何稳定这个演示并从左侧创建弧形 - https://jsfiddle.net/smo6kqzw/3/
var svg = d3.select('body').append('svg').attr("height", 500).attr("width", 500).attr("class", "svger")
var rectbuf = 50;
var padd = 1;
for (let i = 0; i < 20; i++) {
svg.append("rect")
.attr("width", 30)
.attr("height", 20)
.attr("fill", "red")
.attr("y", 20)
.attr("x", rectbuf * 5)
.attr("transform", "rotate("+((5+padd) *i)+")")
}
// create svg element:
var svg = d3.select("#rect").append("svg").attr("width", 800).attr("height", 200)
// Add the path using this helper function
svg.append('rect')
.attr('x', 10)
.attr('y', 120)
.attr('width', 600)
.attr('height', 40)
.attr('stroke', 'black')
.attr('fill', '#69a3b2');
我目前有一个看起来像这样的拨号图表
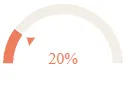
import React from 'react'
import * as d3 from 'd3'
class GaugeChart extends React.Component {
constructor(props) {
super(props)
this.myRef = React.createRef()
this.state = {
data: [],
theme: this.props.theme
? this.props.theme
: ['#bde0fe', '#2698f9', '#71bcfd', '#f1f8fe'],
}
}
componentDidMount() {
var $this = this.myRef.current
d3.select($this).selectAll('svg').remove()
const value = this.props.value
const data = [
{
label: 'Completed',
value: value,
},
{
label: 'Remaining',
value: 100 - value,
},
]
const width = parseInt(this.props.width, 10),
height = parseInt(this.props.height, 10),
radius = parseInt(this.props.r, 10),
innerradius = parseInt(this.props.ir, 10)
var color = d3.scaleOrdinal().range(this.state.theme)
var arc = d3.arc().outerRadius(radius).innerRadius(innerradius)
data.forEach(function (d) {
d.total = +d.value
})
var pie = d3
.pie()
.startAngle(-90 * (Math.PI / 180))
.endAngle(90 * (Math.PI / 180))
.padAngle(0.02) // some space between slices
.sort(null)
.value(function (d) {
return d.total
})
var svg = d3
.select($this)
.append('svg')
.attr('width', width)
.attr('height', height + 5)
.append('g')
.attr('class', 'piechart')
.attr('transform', 'translate(' + width / 2 + ',' + height + ')')
var segments = svg.append('g').attr('class', 'segments')
var slices = segments
.selectAll('.arc')
.data(pie(data))
.enter()
.append('g')
.attr('class', 'arc')
slices
.append('path')
.attr('d', arc)
.attr('fill', function (d, i) {
return color(i)
})
/*.transition()
.attrTween('d', function(d) {
var i = d3.interpolate(d.startAngle + 0.1, d.endAngle);
return function(t) {
d.endAngle = i(t);
return arc(d);
}
} )*/
var arrow = svg.append('g').attr('transform', 'rotate( 0 )')
arrow
.append('path')
.attr('fill', '#ef7e60')
.attr(
'd',
`M -${innerradius - 5},0 -${innerradius - 12},-5 -${
innerradius - 12
},5`,
) // draw triangle
var text = svg
.append('text')
.attr('text-anchor', 'middle')
.attr('dy', 0)
.attr('fill', '#ef7e60')
function update(data) {
text.text(`${Math.round(data[0].value)}%`) // set % text
arrow.transition().attr('transform', `rotate( ${data[0].value * 1.8} )`) //rotate to desired qantity ( i put to 60% of 180 deg )
}
update(data)
}
render() {
return <div ref={this.myRef} className="GaugeChart" />
}
}
export default GaugeChart
-- 最新的fiddle是12月2日 https://jsfiddle.net/gp4wL80t/
最新的fiddle是12月3日 https://jsfiddle.net/0k7zvdxn/