为什么渲染顺序与我的预期不同?
这样做,
最小可重现的示例: {{link1:
}}
window.draw(cell);
window.draw(player);
这样做,
player
应该渲染在任何cell
的上方。但是我得到的结果是(标记线下方的cell
被渲染在player
的上方)- 为什么呢?
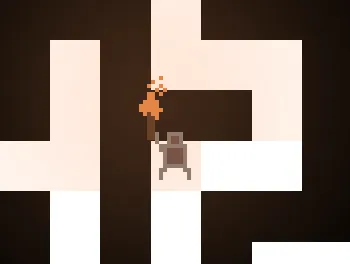
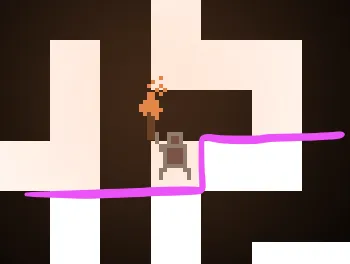
最小可重现的示例: {{link1:
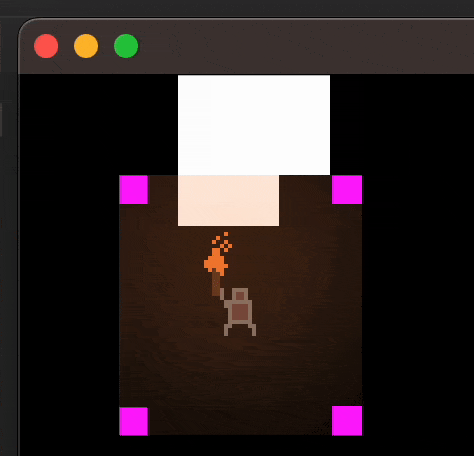
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(1280, 960), "Sample");
sf::Texture texture; texture.loadFromFile("assets/images/player.png");
sf::Sprite sprite(texture);
int **map = new int *[3]{new int[3]{0, 0, 0}, new int[3]{0, 0, 0}, new int[3]{0, 0, 7}};
struct Entity { int x, y; } entity{};
while (window.isOpen()) {
for (auto event = sf::Event(); window.pollEvent(event);) {
std::swap(map[entity.y][entity.x],map[entity.y - (event.type == sf::Event::KeyPressed && event.key.code == sf::Keyboard::Up ? 1 : 0)][entity.x - (event.type == sf::Event::KeyPressed && event.key.code == sf::Keyboard::Left ? 1 : 0)]);
}
window.clear(sf::Color::Black);
float cell_size = 960.f / 19;
for (int y = 0; y < 19; ++y) {
for (int x = 0; x < 19; ++x) {
sf::RectangleShape cell(sf::Vector2f(cell_size, cell_size));
if (x >= 3 || y >= 3) {
continue;
}
switch (map[y][x]) {
case 0:
cell.setPosition(float(x) * cell_size + float(1280 - 19 * cell_size) / 2,float(y) * cell_size);
window.draw(cell);
break;
case 7:
sprite.setPosition(float(x) * cell_size, float(y) * cell_size);
entity = {x, y};
window.draw(sprite);
break;
}
}
}
window.display();
}
}