我需要设计一个三角形并在其中显示一些文本,角度为45度,然后我需要在三角形边界之外放置一个TextView来显示其他文本,就像横幅一样。但是当我使用相对布局并设置三角形背景时,它仍然表现为矩形,遮挡了我的TextView。以下是我使用的代码:
<RelativeLayout
android:id="@+id/relative"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_alignParentTop="true"
android:background="@drawable/image_sticker" >
<com.example.AngledTextView
android:id="@+id/textViewx"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:rotation="52"
android:textColor="#FFFFFF" />
</RelativeLayout>
我的AngledTextView类:
public class AngledTextView extends TextView {
private int mWidth;
private int mHeight;
public AngledTextView(Context context, AttributeSet attrs) {
super(context, attrs);
}
@Override
protected void onDraw(Canvas canvas) {
canvas.save();
/*Paint textPaint = new Paint();
int xPos = (canvas.getWidth() / 2);
int yPos = (int) ((canvas.getHeight() / 2) - ((textPaint.descent() + textPaint.ascent()) / 2)) ;
canvas.rotate(45, xPos,yPos); */
super.onDraw(canvas);
canvas.restore();
}
}
问题:
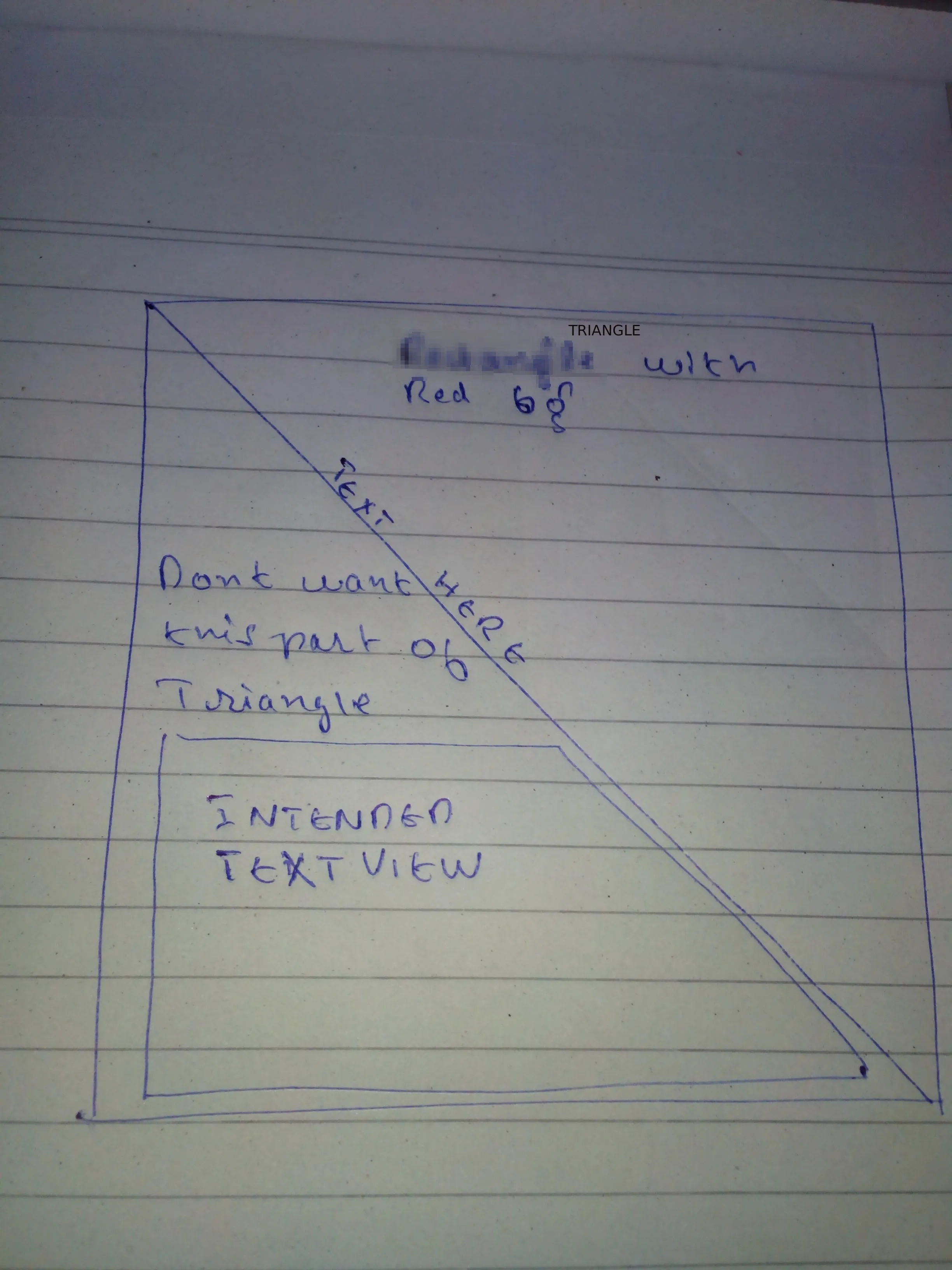