我该如何在两个圆之间绘制带箭头的线条,已知以下信息:
如果我将箭头绘制到圆的“中心”处,则箭头将不可见。 如果我将箭头向后移动太远,则线条会显示并隐藏箭头的尖端(这里为了更好的可见性而夸张):
按照要求,这是我的代码的相关部分(使用LiveScript编写):
- 圆心位置
- 圆的半径
如果我将箭头绘制到圆的“中心”处,则箭头将不可见。 如果我将箭头向后移动太远,则线条会显示并隐藏箭头的尖端(这里为了更好的可见性而夸张):
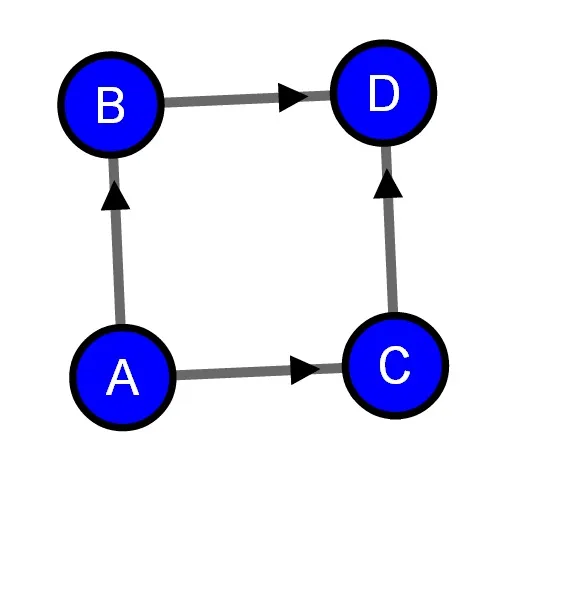
# Draw an arrow to use for lines
svg.append("svg:defs")
.append("svg:marker")
.attr("id", "arrow")
.attr("viewBox", "0 0 10 10")
.attr("refX", 27)
.attr("refY", 5)
.attr("markerUnits", "strokeWidth")
.attr("markerWidth", 8)
.attr("markerHeight", 6)
.attr("orient", "auto")
.append("svg:path")
.attr("d", "M 0 0 L 10 5 L 0 10 z")
svg.append("line")
.attr "x1" 5
.attr "x2" 50
.attr "y1" 5
.attr "y2" 50
.style "stroke" "black"
.attr "stroke-width" 2
.attr "marker-end" "url(\#arrow)"
另外,这里是工作示例的JSFiddle(请注意箭头已经被“捏”成合适的样子):http://jsfiddle.net/yeQS2/
markerWidth
以及markerHeight
,似乎不会出现问题。我已经在此示例中使用滚动条进行了尝试:http://jsfiddle.net/mccannf/ethh8/3/ - mccannf