我决定写一篇medium.com文章来回答这个问题,并已经完成了。以下是解决方案的样子,以及代码。这是文章和解决方案。
https://marklucking.medium.com/a-real-world-alignment-challenges-in-swiftui-2-0-ff440dceae5a
简而言之,我设置了四个标签的正确对齐方式,然后将这四个容器相互对齐。
在此代码中,我包含了一个滑块,以便您更好地理解它的工作原理。
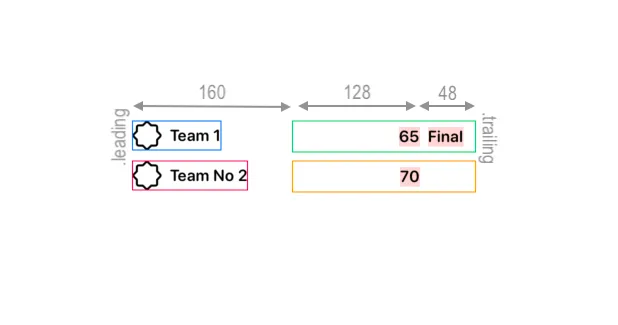
import SwiftUI
struct ContentView: View {
private var newAlignment1H: HorizontalAlignment = .leading
private var newAlignment1V: VerticalAlignment = .top
private var newAlignment2H: HorizontalAlignment = .trailing
private var newAlignment2V: VerticalAlignment = .top
@State private var zeroX: CGFloat = 160
var body: some View {
VStack {
ZStack(alignment: .theAlignment) {
HStack {
Label {
Text("Team 1")
.font(.system(size: 16, weight: .semibold, design: .rounded))
} icon: {
Image(systemName:"seal")
.resizable()
.scaledToFit()
.frame(width: 30)
}.labelStyle(HorizontalLabelStyle())
}
.border(Color.blue)
.alignmentGuide(.theHorizontalAlignment, computeValue: {d in zeroX})
HStack {
Text("65")
.font(.system(size: 16, weight: .semibold, design: .rounded))
.background(Color.red.opacity(0.2))
.frame(width: 128, height: 32, alignment: .trailing)
Text("Final")
.font(.system(size: 16, weight: .semibold, design: .rounded))
.background(Color.red.opacity(0.2))
.frame(width: 48, height: 32, alignment: .leading)
}
.border(Color.green)
}
ZStack(alignment: .theAlignment) {
HStack {
Label {
Text("Team No 2")
.font(.system(size: 16, weight: .semibold, design: .rounded))
} icon: {
Image(systemName:"seal")
.resizable()
.scaledToFit()
.frame(width: 30)
}.labelStyle(HorizontalLabelStyle())
}
.alignmentGuide(.theHorizontalAlignment, computeValue: {d in zeroX})
.border(Color.pink)
HStack {
Text("70")
.font(.system(size: 16, weight: .semibold, design: .rounded))
.background(Color.red.opacity(0.2))
.frame(width: 128, height: 32, alignment: .trailing)
Text("")
.background(Color.red.opacity(0.2))
.frame(width: 48, height: 32, alignment: .leading)
}
.border(Color.orange)
}
VStack {
Slider(value: $zeroX, in: 0...200, step: 10)
Text("\(zeroX)")
}
}
}
}
struct VerticalLabelStyle: LabelStyle {
func makeBody(configuration: Configuration) -> some View {
VStack(alignment: .center, spacing: 8) {
configuration.icon
configuration.title
}
}
}
struct HorizontalLabelStyle: LabelStyle {
func makeBody(configuration: Configuration) -> some View {
HStack(alignment: .center, spacing: 8) {
configuration.icon
configuration.title
}
}
}
extension VerticalAlignment {
private enum TheVerticalAlignment : AlignmentID {
static func defaultValue(in d: ViewDimensions) -> CGFloat {
return d[VerticalAlignment.top]
}
}
static let theVerticalAlignment = VerticalAlignment(TheVerticalAlignment.self)
}
extension HorizontalAlignment {
private enum TheHorizontalAlignment : AlignmentID {
static func defaultValue(in d: ViewDimensions) -> CGFloat {
return d[HorizontalAlignment.leading]
}
}
static let theHorizontalAlignment = HorizontalAlignment(TheHorizontalAlignment.self)
}
extension Alignment {
static let theAlignment = Alignment(horizontal: .theHorizontalAlignment, vertical: .theVerticalAlignment)
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}