我在使用Android动画API时遇到了很多问题。
我需要将一个尺寸为400px*600px的图像从屏幕左下角向上移动,屏幕尺寸为600px*1024px。此外,在动画开始时,图像的大部分应位于屏幕外。
以下是我的布局:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="@drawable/common_background">
<ImageView android:id="@+id/hand"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:scaleType="matrix"
android:src="@drawable/howto_throw_hand" />
</RelativeLayout>
活动的逻辑:
public class MyActivity extends Activity
{
ImageView hand;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
hand = (ImageView)findViewById(R.id.hand);
}
@Override
public void onWindowFocusChanged(boolean hasFocus) {
//initial positioning
RelativeLayout.LayoutParams layoutParams = new RelativeLayout.LayoutParams(
new ViewGroup.MarginLayoutParams(
RelativeLayout.LayoutParams.WRAP_CONTENT,
RelativeLayout.LayoutParams.WRAP_CONTENT
)
);
layoutParams.setMargins(400, 600, 0, 0);
hand.setLayoutParams(layoutParams);
//making animation
TranslateAnimation animation = new TranslateAnimation(
Animation.RELATIVE_TO_SELF, 0, Animation.ABSOLUTE, -100,
Animation.RELATIVE_TO_SELF, 0, Animation.ABSOLUTE, -1000
);
animation.setInterpolator(new LinearInterpolator());
animation.setDuration(3000);
//applying animation
hand.startAnimation(animation);
}
}
带有初始状态的截图:
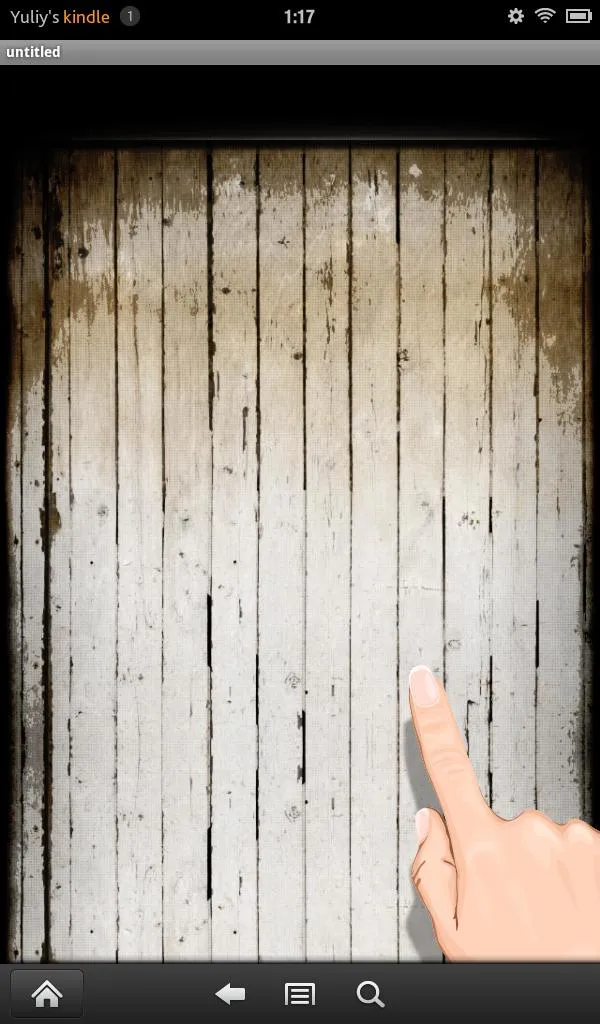
问题在于在动画过程中,图像被裁剪,就像这个视频中演示的那样:带有动画的视频
我花了很多时间,但还是没有能够解决这个问题 =(
有什么方法可以解决这个问题吗?
提前感谢您!
~~~~~~~~~~~~~~~~~~ 已解决 ~~~~~~~~~~~~~~~~~~
正如ben75所说:“...问题在于初始位置。当您进行TranslateAnimation时:图像不会移动:它会以不同的平移重新绘制”,“动画只是转换了第一个裁剪的图像”。
我是如何解决这个问题的:
布局:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="@drawable/common_background">
<ImageView android:id="@+id/hand"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:visibility="invisible"
android:src="@drawable/howto_throw_hand" />
</RelativeLayout>
活动的逻辑:
public class MyActivity extends Activity
{
ImageView hand;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
hand = (ImageView)findViewById(R.id.hand);
}
@Override
public void onWindowFocusChanged(boolean hasFocus) {
//initial positioning — removed
//making animation
TranslateAnimation animation = new TranslateAnimation(400, 300, 600, 0);
animation.setInterpolator(new LinearInterpolator());
animation.setDuration(3000);
//applying animation
hand.startAnimation(animation);
}
}
轰!