我正在使用Swing编写一个简单的输入框。我使用boxLayout创建了一个用户输入的简单GUI。问题是,在所有标签的JPanel和JTextFields的JPanel之间创建水平支撑会导致整个面板向下移动(奇怪)。这是整个面板的代码:
private JPanel secondCard() {
//main panel. set the boxlayout
secondCard = new JPanel();
secondCard.setLayout(new BoxLayout(secondCard,BoxLayout.Y_AXIS));
// create vertical strut for looks
secondCard.add(Box.createVerticalStrut(20));
// create title. center it.
JLabel title = new JLabel("Configure main network parameters ");
title.setAlignmentX(CENTER_ALIGNMENT);
secondCard.add(title);
// create vertical strut for looks
secondCard.add(Box.createVerticalStrut(20));
// create panel for the description labels
JPanel labelPanel = new JPanel();
labelPanel.setLayout(new BoxLayout(labelPanel,BoxLayout.Y_AXIS));
labelPanel.setAlignmentX(LEFT_ALIGNMENT);
JLabel inPut =new JLabel("number of inputs");
inPut.setAlignmentX(LEFT_ALIGNMENT);
labelPanel.add(inPut);
inPut =new JLabel("number of outputs");
inPut.setAlignmentX(LEFT_ALIGNMENT);
labelPanel.add(inPut);
inPut =new JLabel("number of layers");
inPut.setAlignmentX(LEFT_ALIGNMENT);
labelPanel.add(inPut);
JPanel textFieldPanel = new JPanel();
textFieldPanel.setLayout(new BoxLayout(textFieldPanel,BoxLayout.Y_AXIS));
textFieldPanel.setAlignmentX(LEFT_ALIGNMENT);
JTextField inputTextField = new JTextField();
inputTextField.setAlignmentX(LEFT_ALIGNMENT);
textFieldPanel.add(inputTextField);
inputTextField.setMinimumSize(new Dimension(0,0));
inputTextField = new JTextField();
inputTextField.setAlignmentX(LEFT_ALIGNMENT);
textFieldPanel.add(inputTextField);
inputTextField.setMinimumSize(new Dimension(0,0));
inputTextField = new JTextField();
inputTextField.setAlignmentX(LEFT_ALIGNMENT);
textFieldPanel.add(inputTextField);
inputTextField.setMinimumSize(new Dimension(0,0));
textFieldPanel.setMaximumSize(new Dimension(50, labelPanel.getMaximumSize().height));
JPanel inputPanel = new JPanel();
inputPanel.setLayout(new BoxLayout(inputPanel,BoxLayout.X_AXIS));
inputPanel.setAlignmentX(CENTER_ALIGNMENT);
inputPanel.add(labelPanel);
//this is the problem strut!! it causes inputPanel to shift downwards
inputPanel.add(Box.createHorizontalStrut(20));
inputPanel.add(textFieldPanel);
secondCard.add(inputPanel);
return secondCard;
}
没有支撑杆时它看起来是这样的:
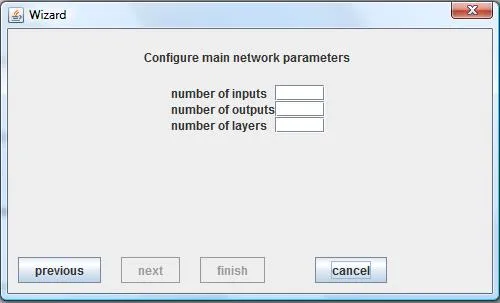
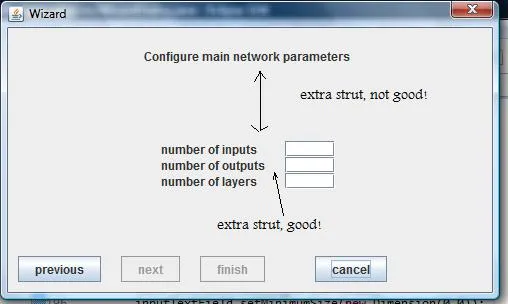
javax.swing.Box
是一个“使用 BoxLayout 对象作为布局管理器的轻量级容器”。Box.Filler
是一个静态嵌套类,与Box
或BoxLayout
配合使用效果非常好。 - trashgod