我想实现一个像 FB 或 G+ 应用程序一样的滑动菜单,我从 FB 菜单演示 和 https://github.com/jfeinstein10/SlidingMenu 找到了一些示例代码,这些可以作为初始代码使用,但我需要更多的功能。比如说目前只能通过点击菜单按钮才能工作,但我希望也可以通过手势来移动它。我希望有这样一种行为:有一个中心视图,在将其向右移动时,会出现一个视图,在将其向左移动时,菜单会出现。比方说有三个视图 A、B、C,当我将 C 向左滑动时,A 就会出现;当我将 C 向右滑动时,B 就会出现。C 位于 A 和 B 的中间。
1.中间视图向右移动
向右移动
2.将中间视图向左侧移动
向左移动
现在我的问题是:开发这种视图的最佳实践是什么?我听说应该使用片段和视图分页器。那么我该如何开发呢?有没有任何人已经实现了样例?任何帮助和建议都会被赞赏。
请参考使用这种滑动视图的应用程序Skout app。
1.中间视图向右移动
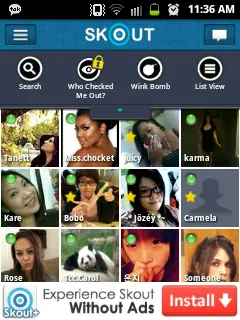
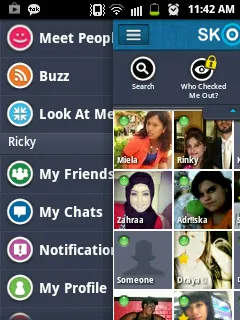
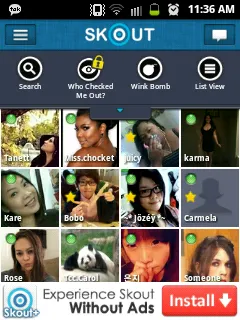
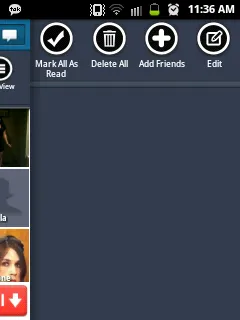
请参考使用这种滑动视图的应用程序Skout app。