嗨,
您需要创建一个新的 UIViewControllerAnimatedTransitioning
。
然后在 animateTransition(using transitionContext: UIViewControllerContextTransitioning)
中编写您的动画代码。
现在在 iOS 10 中,您可以使用 UIViewPropertyAnimator
来动画显示 UIVisualBlurEffect
的 BlurRadius
。
结果: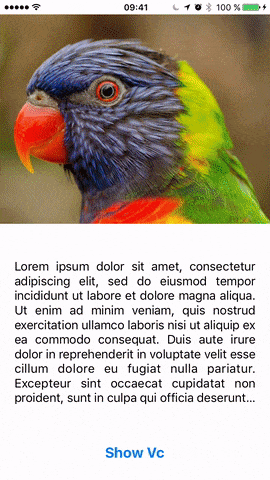
这里有一个用法示例:https://github.com/PierrePerrin/PPBlurModalPresentation
第一步
您需要创建模糊过渡效果。
class BlurModalPresentation: NSObject,UIViewControllerAnimatedTransitioning {
func transitionDuration(using transitionContext: UIViewControllerContextTransitioning?) -> TimeInterval{
return 0.5
}
var blurView = UIVisualEffectView(effect: UIBlurEffect(style: UIBlurEffectStyle.light))
var destinationView : UIView!
var animator: UIViewPropertyAnimator?
func animateTransition(using transitionContext: UIViewControllerContextTransitioning){
let containerView = transitionContext.containerView
_ = transitionContext.viewController(forKey: UITransitionContextViewControllerKey.from)
let toVc = transitionContext.viewController(forKey: UITransitionContextViewControllerKey.to)
destinationView = toVc!.view
destinationView.alpha = 0.0
blurView.effect = nil
blurView.frame = containerView.bounds
self.blurTransition(transitionContext) {
self.unBlur(transitionContext, completion: {
self.blurView.removeFromSuperview()
transitionContext.completeTransition(true)
})
}
containerView.addSubview(toVc!.view)
containerView.addSubview(blurView)
}
func blurTransition(_ context : UIViewControllerContextTransitioning,completion: @escaping () -> Void){
UIViewPropertyAnimator.runningPropertyAnimator(withDuration: self.transitionDuration(using: context)/2, delay: 0, options: UIViewAnimationOptions.curveLinear, animations: {
self.destinationView.alpha = 0.5
self.blurView.effect = UIBlurEffect(style: UIBlurEffectStyle.light)
}, completion: { (position) in
completion()
})
}
func unBlur(_ context : UIViewControllerContextTransitioning,completion: @escaping () -> Void){
UIViewPropertyAnimator.runningPropertyAnimator(withDuration: self.transitionDuration(using: context) / 2, delay:0, options: UIViewAnimationOptions.curveLinear, animations: {
self.destinationView.alpha = 1.0
self.blurView.effect = nil
}, completion: { (position) in
completion()
})
}
}
然后
您需要在ViewController
中设置转场代理:
import UIKit
class ViewController: UIViewController,UIViewControllerTransitioningDelegate {
let blurModalPresentation = BlurModalPresentation()
override func viewDidLoad() {
super.viewDidLoad()
}
func showVC(){
let str = self.storyboard!
let vc = str.instantiateViewController(withIdentifier: "YourViewControllerIdentifier")
vc.transitioningDelegate = self
self.present(vc, animated: true, completion: nil)
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
}
func animationController(forPresented presented: UIViewController, presenting: UIViewController, source: UIViewController) -> UIViewControllerAnimatedTransitioning?{
return blurModalPresentation
}
func animationController(forDismissed dismissed: UIViewController) -> UIViewControllerAnimatedTransitioning?{
return blurModalPresentation
}
}