我正在尝试使用新的Google地图Android API v2开发一个适用于Android 2.3.3或更高版本的应用程序。 这是一个非常简单的应用程序: 它获取用户当前位置(使用GPS或网络信号) 从数据库中获取POI 使用方向API,将用户驾驶到POI。
我的问题是如何获取用户当前位置。感谢这篇文章 How to get the current location in Google Maps Android API v2? 我了解到我不能使用新的Google API更新当前位置。
另一个问题是我可以使用GoogleMap setMyLocationEnabled(boolean enabled)
设置自己的位置,但无法使用getMyLocation()
知道用户在哪里。
我使用了这个指南http://www.vogella.com/articles/AndroidLocationAPI/article.html#maps_mylocation来获取我的位置,并尝试将其集成到我的Activity中以绘制用户位置。
这是我的代码:
清单
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="it.mappe"
android:versionCode="1"
android:versionName="1.0" >
<uses-permission android:name="it.mappe.permission.MAPS_RECEIVE" />
<uses-sdk
android:minSdkVersion="10"
android:targetSdkVersion="16" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="com.google.android.providers.gsf.permission.READ_GSERVICES" />
<uses-feature
android:glEsVersion="0x00020000"
android:required="true" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<meta-data
android:name="com.google.android.maps.v2.API_KEY"
android:value="MYGMAPSKEY" />
<activity
android:name="it.mappe.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
主要活动
package it.mappe;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.model.BitmapDescriptorFactory;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.Marker;
import com.google.android.gms.maps.model.MarkerOptions;
import android.content.Context;
import android.content.Intent;
import android.location.Criteria;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.os.Bundle;
import android.provider.Settings;
import android.support.v4.app.FragmentActivity;
import android.widget.Toast;
public class MainActivity extends FragmentActivity implements LocationListener {
private GoogleMap map;
private static final LatLng ROMA = new LatLng(42.093230818037,11.7971813678741);
private LocationManager locationManager;
private String provider;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
map = ((SupportMapFragment) getSupportFragmentManager().findFragmentById(R.id.map)).getMap();
LocationManager service = (LocationManager) getSystemService(LOCATION_SERVICE);
boolean enabledGPS = service
.isProviderEnabled(LocationManager.GPS_PROVIDER);
boolean enabledWiFi = service
.isProviderEnabled(LocationManager.NETWORK_PROVIDER);
// Check if enabled and if not send user to the GSP settings
// Better solution would be to display a dialog and suggesting to
// go to the settings
if (!enabledGPS) {
Toast.makeText(this, "GPS signal not found", Toast.LENGTH_LONG).show();
Intent intent = new Intent(Settings.ACTION_LOCATION_SOURCE_SETTINGS);
startActivity(intent);
}
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
// Define the criteria how to select the locatioin provider -> use
// default
Criteria criteria = new Criteria();
provider = locationManager.getBestProvider(criteria, false);
Location location = locationManager.getLastKnownLocation(provider);
// Initialize the location fields
if (location != null) {
Toast.makeText(this, "Selected Provider " + provider,
Toast.LENGTH_SHORT).show();
onLocationChanged(location);
} else {
//do something
}
}
/* Request updates at startup */
@Override
protected void onResume() {
super.onResume();
locationManager.requestLocationUpdates(provider, 400, 1, this);
}
/* Remove the locationlistener updates when Activity is paused */
@Override
protected void onPause() {
super.onPause();
locationManager.removeUpdates(this);
}
@Override
public void onLocationChanged(Location location) {
double lat = location.getLatitude();
double lng = location.getLongitude();
Toast.makeText(this, "Location " + lat+","+lng,
Toast.LENGTH_LONG).show();
LatLng coordinate = new LatLng(lat, lng);
Toast.makeText(this, "Location " + coordinate.latitude+","+coordinate.longitude,
Toast.LENGTH_LONG).show();
Marker startPerc = map.addMarker(new MarkerOptions()
.position(coordinate)
.title("Start")
.snippet("Inizio del percorso")
.icon(BitmapDescriptorFactory.fromResource(R.drawable.ic_launcher)));
}
@Override
public void onProviderDisabled(String provider) {
Toast.makeText(this, "Enabled new provider " + provider,
Toast.LENGTH_SHORT).show();
}
@Override
public void onProviderEnabled(String provider) {
Toast.makeText(this, "Disabled provider " + provider,
Toast.LENGTH_SHORT).show();
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
// TODO Auto-generated method stub
}
}
主要布局
<?xml version="1.0" encoding="utf-8"?>
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/map"
android:layout_width="match_parent"
android:layout_height="match_parent"
class="com.google.android.gms.maps.SupportMapFragment" />
它能工作,但不够好,正如你可以在图片中看到的,每当有新位置时,它都会添加一个新标记(我认为是覆盖层...我从未使用过旧版的谷歌地图 API)。
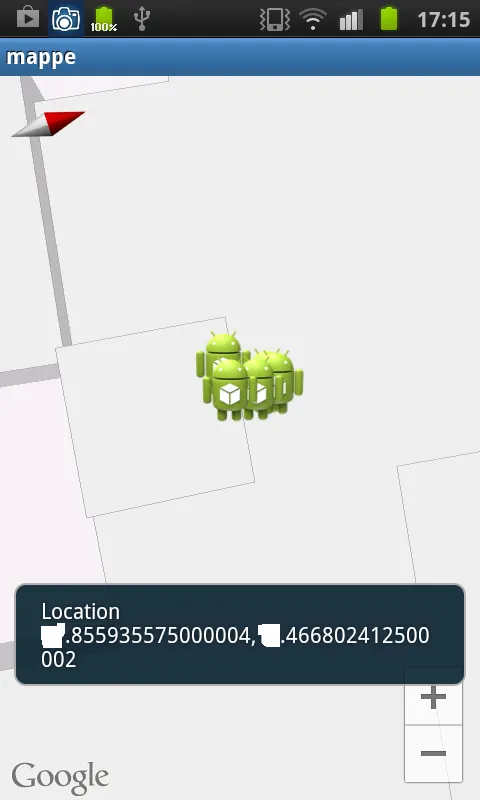
我该如何只显示一个标记?
此外,在我的地图上,我需要显示 POI 标记,所以我认为删除所有标记并在地图上重新绘制它不是一个好的解决方案。有另一种更好的方法来获得用户当前位置,每次更新并仅显示一个自定义标记吗?
Criteria criteria = Criteria() { Accuracy = Accuracy.Fine };
- Adrián Rodríguez