框架: fabricjs
我的第一个问题是如何在两条线之间画角度。我的代码可以运行,但我对结果不满意。
我的第二个问题是在两个点之间画一条曲线。
关于第一个问题的代码。 我有三个点: A,B,C
2条线: AB,BC
利用这些信息,我计算出距离为10的距离点。
let angle = this.calcAngle(A,B,C);
let distanceAB = this.calcCornerPoint(A, B, 10);
let distanceBC = this.calcCornerPoint(C, B, 10);
计算角度:
calcAngle(A, B, C, final, finalAddon = "°") {
var nx1 = A.x - B.x;
var ny1 = A.y - B.y;
var nx2 = C.x - B.x;
var ny2 = C.y - B.y;
this.lineAngle1 = Math.atan2(ny1, nx1);
this.lineAngle2 = Math.atan2(ny2, nx2);
if (nx1 * ny2 - ny1 * nx2 < 0) {
const t = lineAngle2;
this.lineAngle2 = this.lineAngle1;
this.lineAngle1 = t;
}
// if angle 1 is behind then move ahead
if (lineAngle1 < lineAngle2) {
this.lineAngle1 += Math.PI * 2;
}
}
使用以下步骤绘制路径:
this.drawAngleTrapez(distanceAB, distanceBC, B);
drawAngleTrapez(AB, BC, B) {
let points = [AB, BC, B];
let path = "";
if (this.trapezObjs[this.iterator]) {
this.canvas.remove(this.trapezObjs[this.iterator]);
}
path += "M " + Math.round(points[0].x) + " " + Math.round(points[0].y) + "";
for (let i = 1; i < points.length; i++) {
path += " L " + Math.round(points[i].x) + " " + Math.round(points[i].y) + "";
}
this.currentTrapez = this.trapezObjs[this.iterator] = new fabric.Path(path, {
selectable: false,
hasControls: false,
hasBorders: false,
hoverCursor: 'default',
fill: '#ccc',
strokeWidth: this.strokeWidth,
});
this.canvas.add(this.trapezObjs[this.iterator]);
}
然后我画了一个圆形:
drawAnglePoint(B,d = 10) {
this.currentCorner = new fabric.Circle({
left: B.x,
top: B.y,
startAngle: this.lineAngle1,
endAngle: this.lineAngle2,
radius: 10,
fill: '#ccc',
selectable: false,
hasControls: false,
hasBorders: false,
hoverCursor: 'default',
});
this.canvas.add(this.currentCorner);
}
但是结果并不美观:
并且蓝点不在线的末端,或许还需要一些修正。
this.startPoint.set({ left: C.x, top: C.y });
第二个问题已解决:我的计算有误。
问题是,这不是一个漂亮的曲线:
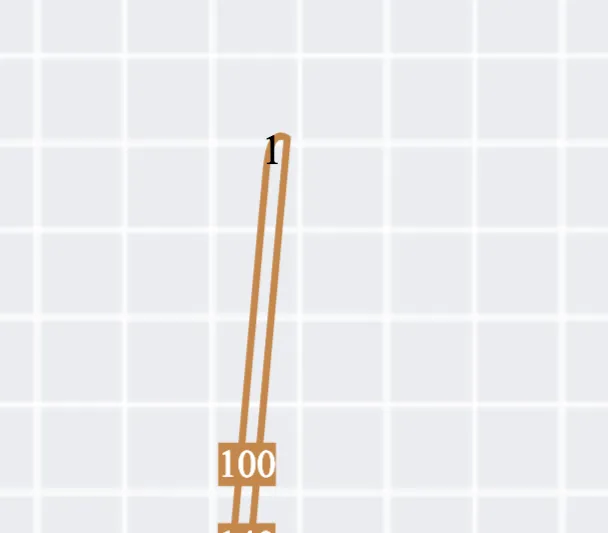