你可以使用
prompt_toolkit
来实现这个功能。
如果需要,这里有相关文档:
你可以按照示例添加语法高亮到输入中。
Adding syntax highlighting is as simple as adding a lexer. All of the Pygments lexers can be used after wrapping them in a PygmentsLexer. It is also possible to create a custom lexer by implementing the Lexer abstract base class.
from pygments.lexers.html import HtmlLexer
from prompt_toolkit.shortcuts import prompt
from prompt_toolkit.lexers import PygmentsLexer
text = prompt('Enter HTML: ', lexer=PygmentsLexer(HtmlLexer))
print('You said: %s' % text)
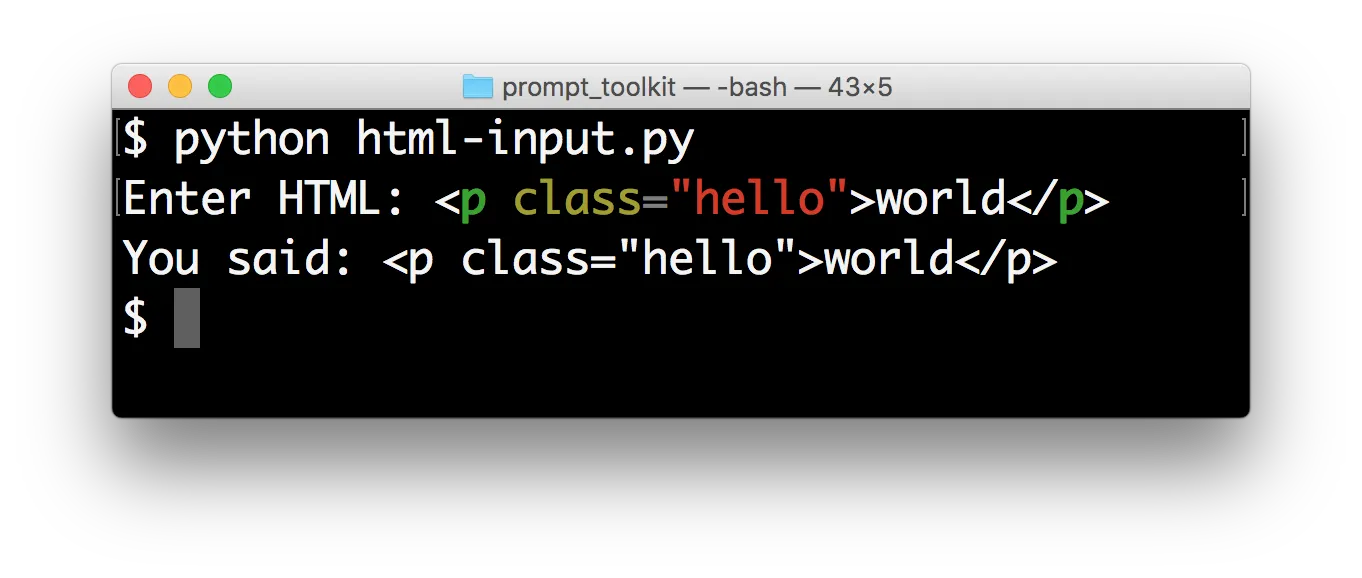
与上面类似,您可以创建定制的
prompt_toolkit.lexers.Lexer
来进行计算器高亮,就像以下示例一样。在这里,我创建了一个自定义帮助类:
from typing import Callable
from prompt_toolkit.document import Document
from prompt_toolkit.formatted_text.base import StyleAndTextTuples
from prompt_toolkit.formatted_text import FormattedText
from prompt_toolkit.shortcuts import prompt
import prompt_toolkit.lexers
import re
class CustomRegexLexer(prompt_toolkit.lexers.Lexer):
def __init__(self, regex_mapping):
super().__init__()
self.regex_mapping = regex_mapping
def lex_document(self, document: Document) -> Callable[[int], StyleAndTextTuples]:
def lex(_: int):
line = document.text
tokens = []
while len(line) != 0:
for pattern, style_string in self.regex_mapping.items():
match: re.Match = pattern.search(line)
if not match:
continue
else:
pass
match_string = line[:match.span()[1]]
line = line[match.span()[1]:]
tokens.append((style_string, match_string))
break
return tokens
return lex
现在我们已经实现了上述帮助类,可以创建我们的正则表达式模式及其相应的样式。要了解有关样式字符串中可以包含哪些内容,请转到
此页面。
operators_allowed = ["+", "-", "/", "*", "(", ")", "=", "^"]
operators = re.compile("^["+''.join([f"\\{x}" for x in operators_allowed])+"]")
numbers = re.compile(r"^\d+(\.\d+)?")
text = re.compile(r"^.")
regex_mapping = {
operators: "#ff70e5",
numbers: "#ffa500",
text: "#2ef5ff",
}
MyCalculatorLexer = CustomRegexLexer(regex_mapping)
有了创建的词法分析器,现在您可以在函数提示中使用该词法分析器:
text = prompt("Enter Equation: ", lexer=MyCalculatorLexer)
def input_maths(message):
return prompt(message, lexer=MyCalculatorLexer)
text = input_maths("Enter Equation: ")
这里是一些示例输出:

现在一切都正常了。还要检查一下
prompt_toolkit
,你可以像
gallery中展示的那样创建大量自定义内容。
rich
吗?它可能适合你 https://github.com/willmcgugan/rich - Prayson W. Daniel