我有一个错误列表
我想根据“状态”和“严重性”对这些错误进行分组。我使用了以下代码来实现它。
这个Linq查询会输出以下内容。
然而,我希望得到以下输出
能否通过对
数据网格中的输出将如下所示:
MyBugList
,它是使用以下类创建的。internal class BugDetails
{
public int Id { get; set; }
public string State { get; set; }
public string Severity { get; set; }
}
我想根据“状态”和“严重性”对这些错误进行分组。我使用了以下代码来实现它。
var BugListGroup = (from bug in MyBugList
group bug by new
{
bug.State,
bug.Severity
} into grp
select new
{
BugState = grp.Key.State,
BugSeverity = grp.Key.Severity,
BugCount = grp.Count()
}).OrderBy(x=> x.BugState).ToList();
这个Linq查询会输出以下内容。
Closed Critical 40
Active Critical 167
Closed Medium 819
Closed Low 323
Resolved Medium 61
Resolved Low 11
Closed High 132
Active Low 17
Active Medium 88
Active High 38
Resolved High 4
Resolved Critical 22
Deferred High 11
然而,我希望得到以下输出
Critical High Medium Total
Closed 3 4 5 12
Active 5 4 5 14
Resolved 6 4 5 15
Deferred 1 4 5 10
Total 15 16 20 51
能否通过对
MyBugList
或 BugListGroup
进行 LINQ 查询来获得此内容?
我想要将输出作为列表获取,以便可以将其用作数据网格的源。
注意:状态和严重性值是动态的,不能硬编码。
以下是我根据Dmitriy Zapevalov提供的答案实现的。
private void button1_Click(object sender, EventArgs e)
{
var grouped = MyBugList.GroupBy(b => b.State).Select(stateGrp => stateGrp.GroupBy(b => b.Severity));
//Setting DataGrid properties
dataGridBug.Rows.Clear();
dataGridBug.Columns.Clear();
dataGridBug.DefaultCellStyle.NullValue = "0";
dataGridBug.DefaultCellStyle.Alignment = DataGridViewContentAlignment.MiddleCenter;
//Declaring DataGrid Styles
var gridBackColor = Color.AliceBlue;
var gridFontStyle = new Font(Font, FontStyle.Bold | FontStyle.Italic);
//Declaring column and row Ids
const string stateColumnId = "State";
const string totalColumnId = "Total";
const string totalRowId = "Total";
//Adding first column
dataGridBug.Columns.Add(stateColumnId, stateColumnId);
dataGridBug.Columns[0].DefaultCellStyle.Alignment = DataGridViewContentAlignment.MiddleLeft;
//Adding other columns
foreach (var strSeverity in MyBugList.Select(b => b.Severity).Distinct())
{
dataGridBug.Columns.Add(strSeverity, strSeverity);
}
//Adding Total Column
var totColPos = dataGridBug.Columns.Add(totalColumnId, totalColumnId);
var totCol = dataGridBug.Columns[totColPos];
//Adding data to grid
foreach (var state in grouped)
{
var nRow = dataGridBug.Rows.Add();
var severities = state as IList<IGrouping<string, BugDetails>> ?? state.ToList();
dataGridBug.Rows[nRow].Cells[0].Value = severities.First().First().State;
var sevCount = 0;
foreach (var severity in severities)
{
dataGridBug.Rows[nRow].Cells[severity.Key].Value = severity.Count();
sevCount += severity.Count();
}
dataGridBug.Rows[nRow].Cells[totalColumnId].Value = sevCount;
}
//Adding total row
var totRowPos = dataGridBug.Rows.Add(totalRowId);
var totRow = dataGridBug.Rows[totRowPos];
//Adding data to total row
for (var c = 1; c < dataGridBug.ColumnCount; c++)
{
var sum = 0;
for (var i = 0; i < dataGridBug.Rows.Count; ++i)
{
sum += Convert.ToInt32(dataGridBug.Rows[i].Cells[c].Value);
}
dataGridBug.Rows[totRowPos].Cells[c].Value = sum;
}
//Styling total column
totCol.DefaultCellStyle.BackColor = gridBackColor;
totCol.DefaultCellStyle.Font = gridFontStyle;
//Styling total row
totRow.DefaultCellStyle.BackColor = gridBackColor;
totRow.DefaultCellStyle.Font = gridFontStyle;
}
数据网格中的输出将如下所示:
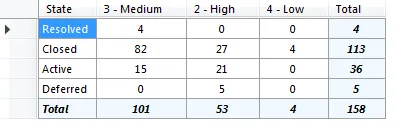