David Dale 问道:
更新. 根据文档,可以得知gz
文件不能在a
模式下打开。那么,现有归档中添加或更新文件的最佳方法是什么?
简短回答:
- 解压 / 解包归档
- 替换 / 添加文件
- 重新打包 / 压缩归档
我尝试使用gzip
和tarfile
以及文件/流接口在内存中执行此操作,但未能成功运行 - 由于替换文件显然不可能,因此必须重写tarball。 因此,最好只是解包整个归档。
维基百科关于tar,gzip。
如果直接运行脚本,还会尝试生成测试图像"a.png、b.png、c.png、new.png"(需要Pillow)和初始存档"test.tar.gz"(如果它们不存在)。 然后,将归档解压缩到临时目录中,将"a.png"覆盖为"new.png"的内容,并打包所有文件,覆盖原始归档。以下是各个文件:
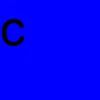
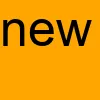
当然,也可以按顺序运行脚本函数进行交互式模式,以便有机会查看文件。假设脚本文件名为“t.py”:
>>> from t import *
>>> make_images()
>>> make_archive()
>>> replace_file()
Workaround
下面开始(最重要的部分在replace_file()
函数中):
"""
Replace a file in a .tar.gz archive via temporary files
https://dev59.com/u14c5IYBdhLWcg3wEGp_
"""
import sys
import pathlib
import tempfile
import tarfile
gfn = "test.tar.gz"
iext = ".png"
replace = "a"+iext
replacement = "new"+iext
def make_images():
"""Generate 4 test images with Pillow (PIL fork, http://pillow.readthedocs.io/)"""
try:
from PIL import Image, ImageDraw, ImageFont
font = ImageFont.truetype("arial.ttf", 50)
for k,v in {"a":"red", "b":"green", "c":"blue", "new":"orange"}.items():
img = Image.new('RGB', (100, 100), color=v)
d = ImageDraw.Draw(img)
d.text((0, 0), k, fill=(0, 0, 0), font=font)
img.save(k+iext)
except Exception as e:
print(e, file=sys.stderr)
print("Could not create image files", file=sys.stderr)
print("(pip install pillow)", file=sys.stderr)
def make_archive():
"""Create gzip compressed tar file with the three images"""
try:
t = tarfile.open(gfn, 'w:gz')
for f in 'abc':
t.add(f+iext)
t.close()
except Exception as e:
print(e, file=sys.stderr)
print("Could not create archive", file=sys.stderr)
def make_files():
"""Generate sample images and archive"""
mi = False
for f in ['a','b','c','new']:
p = pathlib.Path(f+iext)
if not p.is_file():
mi = True
if mi:
make_images()
if not pathlib.Path(gfn).is_file():
make_archive()
def add_file_not():
"""Might even corrupt the existing file?"""
print("Not possible: tarfile with \"a:gz\" - failing now:", file=sys.stderr)
try:
a = tarfile.open(gfn, 'a:gz')
a.add(replacement, arcname=replace)
a.close()
except Exception as e:
print(e, file=sys.stderr)
def replace_file():
"""Extract archive to temporary directory, replace file, replace archive """
print("Workaround", file=sys.stderr)
with tempfile.TemporaryDirectory() as td:
tdp = pathlib.Path(td)
with tarfile.open(gfn) as r:
r.extractall(td)
(tdp/replace).write_bytes( pathlib.Path(replacement).read_bytes() )
with tarfile.open(gfn, "w:gz") as w:
for f in tdp.iterdir():
w.add(f, arcname=f.name)
def test():
"""as the name suggests, this just runs some tests ;-)"""
make_files()
replace_file()
if __name__ == "__main__":
test()
如果您想添加文件而不是替换它们,显然只需省略替换临时文件的行,并将其他文件复制到临时目录中。确保
pathlib.Path.iterdir
也“看到”要添加到新存档中的新文件。
我已经将其放入一个更有用的函数中:
def targz_add(targz=None, src=None, dst=None, replace=False):
"""Add <src> file(s) to <targz> file, optionally replacing existing file(s).
Uses temporary directory to modify archive contents.
TODO: complete error handling...
"""
import sys, pathlib, tempfile, tarfile
tp = pathlib.Path(targz)
if not tp.is_file():
sys.stderr.write("Target '{}' does not exist!\n".format(tp) )
return 1
if not src:
sys.stderr.write("No files given.\n")
return 1
if not isinstance(src, (tuple, list, set)):
src = [src]
srcp = []
for s in src:
sp = pathlib.Path(s)
if not sp.is_file():
sys.stderr.write("Source '{}' does not exist.\n".format(sp) )
else:
srcp.append(sp)
if not srcp:
sys.stderr.write("None of the files exist.\n")
return 1
dstp = []
if not dst:
dstp = [sp.name for sp in srcp]
else:
if callable(dst):
dstp = [pathlib.Path(c) for c in map(dst, srcp)]
elif not isinstance(dst, (tuple, list, set)):
dstp = [pathlib.Path(dst).name]
elif isinstance(dst, (tuple, list, set)):
dstp = [pathlib.Path(d) for d in dst]
else:
sys.stderr.write("Please fix me, I cannot handle the destination(s) '{}'\n".format(dst) )
return 1
if not dstp:
sys.stderr.write("None of the files exist.\n")
return 1
sdp = zip(srcp, dstp)
with tempfile.TemporaryDirectory() as tempdir:
tempdirp = pathlib.Path(tempdir)
with tarfile.open(tp) as r:
r.extractall(tempdirp)
for s,d in sdp:
dp = tempdirp/d
if not dp.is_file or replace:
sys.stderr.write("Writing '{1}' (from '{0}')\n".format(s,d) )
dp.write_bytes( s.read_bytes() )
else:
sys.stderr.write("Skipping '{1}' (from '{0}')\n".format(s,d) )
with tarfile.open(tp, "w:gz") as w:
for f in tempdirp.iterdir():
w.add(f, arcname=f.name)
return None
以下是一些示例“测试”:
targz_add("test.tar.gz", "new.png", lambda x:str(x).replace("new","a"), replace=True)
shutil
同样支持压缩文件,但不支持向已有压缩文件中添加文件:
https://docs.python.org/zh-cn/3/library/shutil.html#archiving-operations
自版本3.2起新增。
自版本3.5起更改:增加对 xztar 格式的支持。
还提供了高级实用工具来创建和读取压缩和归档文件。它们依赖于 zipfile
和 tarfile
模块。
Here's adding a file by extracting to memory using io.BytesIO, adding, and compressing:
import io
import gzip
import tarfile
gfn = "test.tar.gz"
replace = "a.png"
replacement = "new.png"
print("reading {}".format(gfn))
m = io.BytesIO()
with gzip.open(gfn) as g:
m.write(g.read())
print("opening tar in memory")
m.seek(0)
with tarfile.open(fileobj=m, mode="a") as t:
t.list()
print("adding {} as {}".format(replacement, replace))
t.add(replacement, arcname=replace)
t.list()
print("writing {}".format(gfn))
m.seek(0)
with gzip.open(gfn, "wb") as g:
g.write(m.read())
it prints
reading test.tar.gz
opening tar in memory
?rw-rw-rw- 0/0 877 2018-04-11 07:38:57 a.png
?rw-rw-rw- 0/0 827 2018-04-11 07:38:57 b.png
?rw-rw-rw- 0/0 787 2018-04-11 07:38:57 c.png
adding new.png as a.png
?rw-rw-rw- 0/0 877 2018-04-11 07:38:57 a.png
?rw-rw-rw- 0/0 827 2018-04-11 07:38:57 b.png
?rw-rw-rw- 0/0 787 2018-04-11 07:38:57 c.png
-rw-rw-rw- 0/0 2108 2018-04-11 07:38:57 a.png
writing test.tar.gz
Optimizations are welcome!