只需将字典类型转换为json_str,使用 json.dumps(adict)
。
import pandas as pd
import json
import psycopg2
from sqlalchemy import create_engine
engine_nf = create_engine('postgresql+psycopg2://user:password@192.168.100.120:5432/database')
sql_read = lambda sql: pd.read_sql(sql, engine_nf)
sql_execute = lambda sql: pd.io.sql.execute(sql, engine_nf)
sql = '''
CREATE TABLE if not exists product (
store_id int
, url text
, price text
, charecteristics json
, color text
, dimensions text
)
'''
_ = sql_execute(sql)
thedictionary = {'price money': '$1', 'name': 'Google',
'color': '', 'imgurl': 'http://www.google.com/images/nav_logo225.png',
'charateristics': 'No Description',
'store': 'google'}
sql = '''
INSERT INTO product(store_id, url, price, charecteristics, color, dimensions)
VALUES (%d, '%s', '%s', '%s', '%s', '%s')
''' % (1, 'http://www.google.com', '$20',
json.dumps(thedictionary), 'red', '8.5x11')
sql_execute(sql)
sql = '''
select *
from product
'''
df = sql_read(sql)
df
charecteristics = df['charecteristics'].iloc[0]
type(charecteristics)
事实上,我喜欢另一种将数据转储到Postgres的方式。
import io
import csv
def df2db(df_a, table_name, engine):
output = io.StringIO()
df_a.to_csv(output, sep='\t', index = False, header = False, quoting=csv.QUOTE_NONE)
output.getvalue()
output.seek(0)
connection = engine.raw_connection()
cursor = connection.cursor()
cursor.copy_from(output,table_name,null='')
connection.commit()
cursor.close()
df = sql_read('select * from product')
type(df.charecteristics.iloc[0])
df.charecteristics = df.charecteristics.map(json.dumps)
df2db(df, 'product', engine_nf)
df_end = sql_read('select * from product')
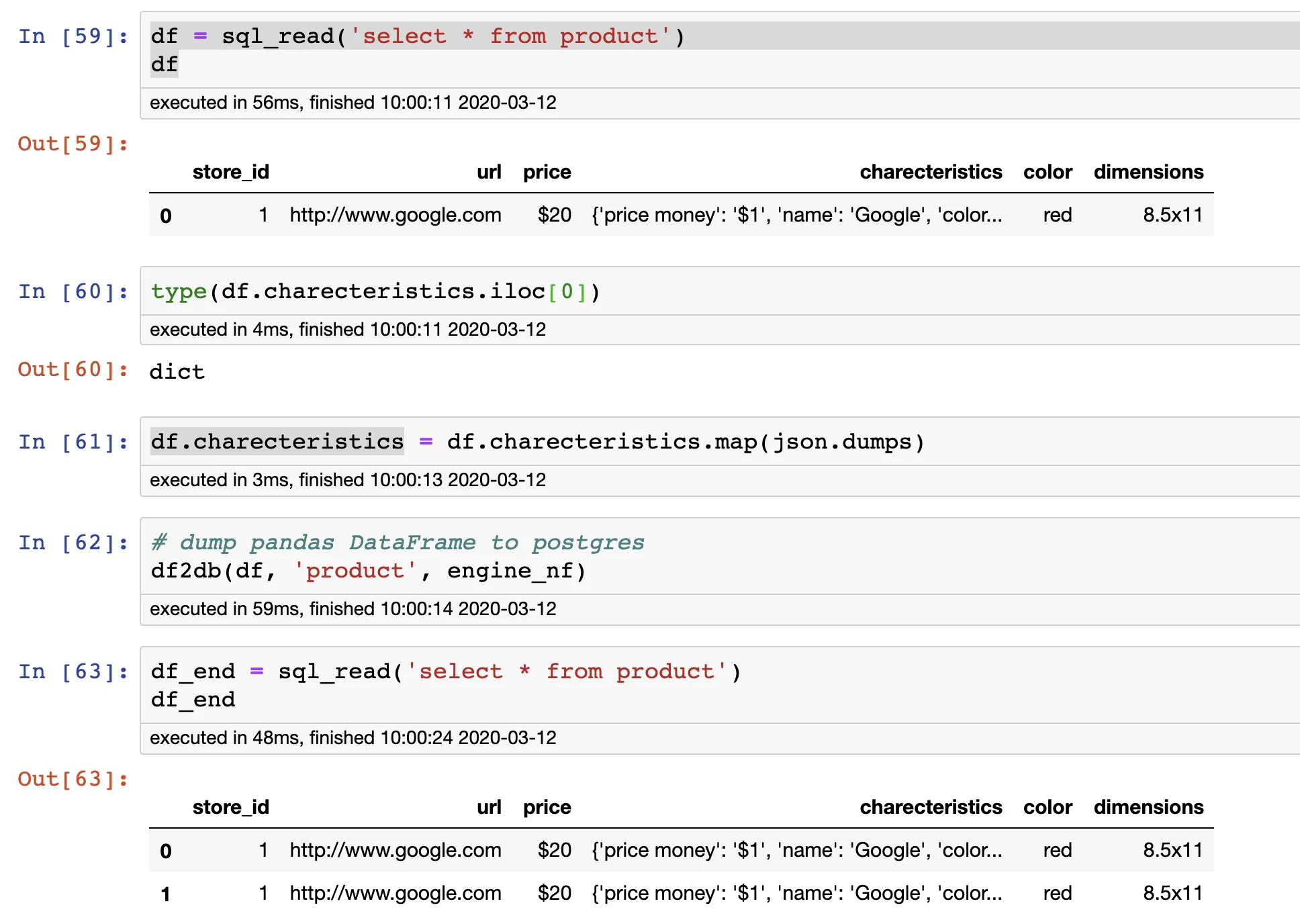