我一直在开发一个画布游戏,遇到了一些内存泄漏的问题。我以为问题与渲染和删除实体有关,但我在不渲染任何内容的情况下运行代码,发现音频调度对象本身就会导致泄漏。这个问题会导致音频在一段时间后开始发出噼啪声并停止播放。游戏仍然可以渲染,但音频停止播放——我还注意到角色的射击速度变得更慢(射击时间与调度器函数中的音符一起进行调度)。
游戏链接:Link to game 我用来处理音频的代码来自于A Tale of Two Clocks' tutorial。当我在Chrome上运行节拍器的代码并进行时间轴记录时,堆分配会增加。 为什么这段代码会导致内存泄漏?在我看来它很好。计时器ID设置为空值? 图1:节拍器本身的堆时间轴
图2:我的应用程序仅运行节拍器函数的时间轴(没有游戏实体)
图3:我的应用程序正常运行
这是代码:
游戏链接:Link to game 我用来处理音频的代码来自于A Tale of Two Clocks' tutorial。当我在Chrome上运行节拍器的代码并进行时间轴记录时,堆分配会增加。 为什么这段代码会导致内存泄漏?在我看来它很好。计时器ID设置为空值? 图1:节拍器本身的堆时间轴

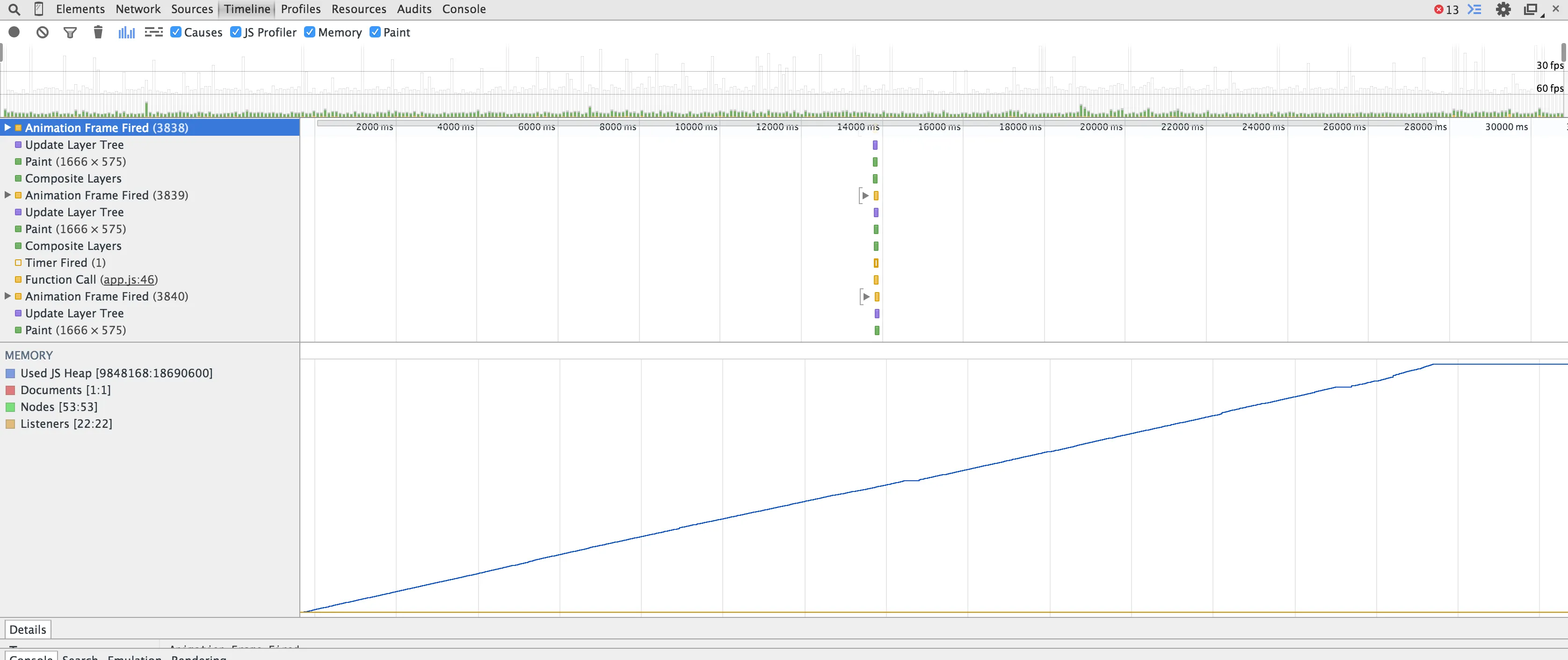

function init(){
var container = document.createElement( 'div' );
// CREATE CANVAS ... ...
audioContext = new AudioContext();
requestAnimFrame(draw);
timerWorker = new Worker("js/metronomeworker.js");
timerWorker.onmessage = function(e) {
if (e.data == "tick") {
// console.log("tick!");
scheduler();
}
else {
console.log("message: " + e.data);
}
};
timerWorker.postMessage({"interval":lookahead});
}
function nextNote() {
// Advance current note and time by a 16th note...
var secondsPerBeat = 60.0 / tempo; // Notice this picks up the CURRENT
// tempo value to calculate beat length.
nextNoteTime += 0.25 * secondsPerBeat; // Add beat length to last beat time
current16thNote++; // Advance the beat number, wrap to zero
if (current16thNote == 16) {
current16thNote = 0;
}
}
function scheduleNote( beatNumber, time ) {
// push the note on the queue, even if we're not playing.
notesInQueue.push( { note: beatNumber, time: time } );
if ( (noteResolution==1) && (beatNumber%2))
return; // we're not playing non-8th 16th notes
if ( (noteResolution==2) && (beatNumber%4))
return; // we're not playing non-quarter 8th notes
// create an oscillator // create sample
var osc = audioContext.createOscillator();
osc.connect( audioContext.destination );
if (beatNumber % 16 === 0) // beat 0 == low pitch
osc.frequency.value = 880.0;
else if (beatNumber % 4 === 0 ) // quarter notes = medium pitch
osc.frequency.value = 440.0;
else // other 16th notes = high pitch
osc.frequency.value = 220.0;
osc.start( time ); //sound.play(time)
osc.stop( time + noteLength ); // " "
}
function scheduler() {
// while there are notes that will need to play before the next interval,
// schedule them and advance the pointer.
while (nextNoteTime < audioContext.currentTime + scheduleAheadTime ) {
scheduleNote( current16thNote, nextNoteTime );
nextNote();
}
}
function play() {
isPlaying = !isPlaying;
if (isPlaying) { // start playing
current16thNote = 0;
nextNoteTime = audioContext.currentTime;
timerWorker.postMessage("start");
return "stop";
} else {
timerWorker.postMessage("stop");
return "play";
}
}
Metronome.js:
var timerID=null;
var interval=100;
self.onmessage=function(e){
if (e.data=="start") {
console.log("starting");
timerID=setInterval(function(){postMessage("tick");},interval)
}
else if (e.data.interval) {
console.log("setting interval");
interval=e.data.interval;
console.log("interval="+interval);
if (timerID) {
clearInterval(timerID);
timerID=setInterval(function(){postMessage("tick");},interval)
}
}
else if (e.data=="stop") {
console.log("stopping");
clearInterval(timerID);
timerID=null;
}
};
如何在scheduleNote()中安排声音(和拍摄):
if (beatNumber % 4 === 0) {
playSound(samplebb[0], gainNode1);
}
if (planet1play === true) {
if (barNumber % 4 === 0)
if (current16thNote % 1 === 0) {
playSound(samplebb[26], planet1gain);
}
}
if (shootx) {
// Weapon 1
if (gun === 0) {
if (beatNumber === 2 || beatNumber === 6 || beatNumber === 10 || beatNumber === 14) {
shoot(bulletX, bulletY);
playSound(samplebb[3], gainNode2);
}
}
更新
即使我在游戏中不渲染或更新任何内容,音频仍然存在问题。在较慢的机器上问题更加严重。您可以通过此处链接查看该游戏。
不知道为什么会出现这种情况,可能是某种音频缓存问题?有人有什么想法吗?