考虑到嵌套列表长这样
L = [['John','Sayyed'],['John','Simon'],['bush','trump'],['Sam','Suri','NewYork'],['Suri','Orlando','Canada']]
以下是我分享的两个潜在选项来解决 OP 的问题。
选项1
使用(注释使其自我解释):
L = [set(l) for l in L]
for i in range(len(L)):
for j in range(i+1,len(L)):
if len(L[i].intersection(L[j])) > 0:
L[i] = L[i].union(L[j])
L[j] = set()
L = [list(l) for l in L if len(l) > 0]
这将给我们
[['John', 'Sayyed', 'Simon'], ['bush', 'trump'], ['NewYork', 'Sam', 'Canada', 'Suri', 'Orlando']]
为方便起见,你可能想要进行重构。
def union(L):
L = [set(l) for l in L]
for i in range(len(L)):
for j in range(i+1,len(L)):
if len(L[i].intersection(L[j])) > 0:
L[i] = L[i].union(L[j])
L[j] = set()
L = [list(l) for l in L if len(l) > 0]
return L
然后简单地
L_new = union(L)
[Out]: [['John', 'Sayyed', 'Simon'], ['bush', 'trump'], ['NewYork', 'Sam', 'Canada', 'Suri', 'Orlando']]
选项 2
使用 NetworkX
。
首先创建一个没有节点和边的空图。
G = nx.Graph()
然后,为了添加节点,有各种选项,比如使用{{link1:Graph.add_nodes_from
}}和{{link2:Graph.add_edge
}}。
for l in L:
G.add_nodes_from(l)
for i in range(len(l)):
for j in range(i+1,len(l)):
G.add_edge(l[i],l[j])
for l in L:
G.add_nodes_from(l)
for i in range(len(l)-1):
G.add_edge(l[i],l[i+1])
最后,我们可以使用 connected_components
获取连接组件的列表。
L = list(nx.connected_components(G))
[Out]: [{'John', 'Sayyed', 'Simon'}, {'bush', 'trump'}, {'NewYork', 'Sam', 'Canada', 'Suri', 'Orlando'}]
作为额外的步骤,您可能想要绘制图形。为此,
NetworkX
提供了可视化图形的基本功能。
在这里查看有关使用 NetworkX
绘制图形的更多信息。
一种方法是使用
matplotlib
。
有各种布局可供使用,例如:
spring_layout
import matplotlib.pyplot as plt
pos = nx.spring_layout(G, k=0.5, iterations=20, scale=10)
nx.draw(G, pos, with_labels=True, font_weight='bold', font_color='white', node_size=2500, node_color='blue')
plt.show()
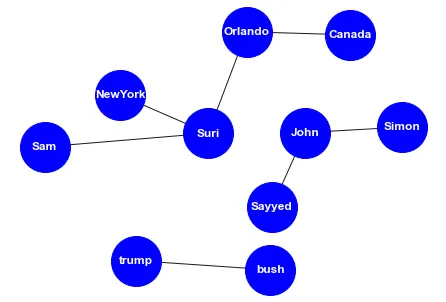
spiral_layout
import matplotlib.pyplot as plt
pos = nx.spiral_layout(G, scale=5)
nx.draw(G, pos, with_labels=True, font_weight='bold', font_color='white', node_size=2500, node_color='blue')
plt.show()
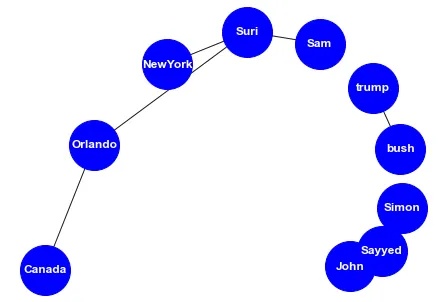
shell_layout
import matplotlib.pyplot as plt
pos = nx.shell_layout(G, scale=10)
nx.draw(G, pos, with_labels=True, font_weight='bold', font_color='white', node_size=2500, node_color='blue')
plt.show()
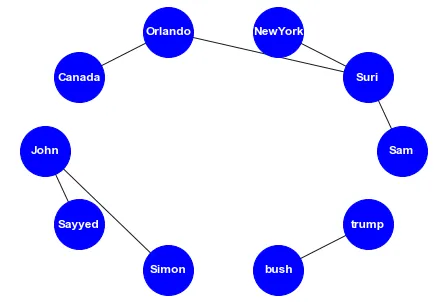
还有更多,取决于使用情况和目标。在此处查看所有可用的布局。