我想制作一个非常灵活的复选框,它可以这样工作:
https://css-tricks.com/indeterminate-checkboxes/
:
我希望我的复选框有三个选项:未选中、已选中和不确定。不确定:如果与此不确定复选框连接的所有复选框都被选中,则此不确定复选框将被选中。
我的复选框看起来像这样:
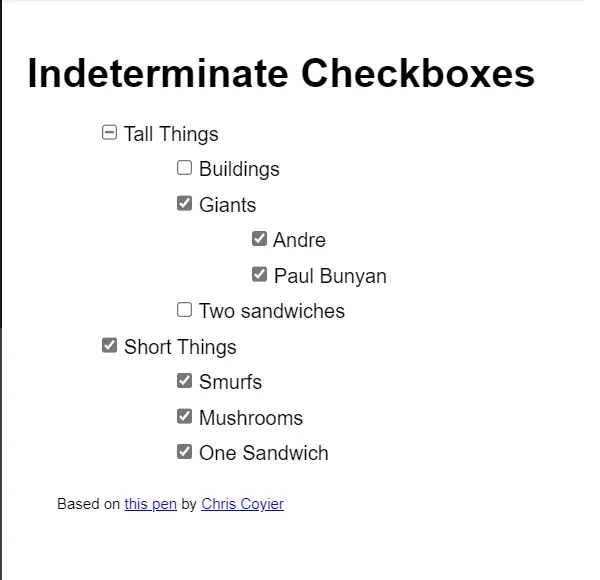
import classNames from 'classnames';
import React from 'react';
interface IProps {
name?: string;
className?: string;
style?: React.CSSProperties;
label?: string;
color?: 'primary' | 'success' | 'secondary' | 'danger' | 'warning' | 'info' | 'light' | 'dark';
value?: string;
disabled?: boolean;
checked?: boolean;
onChange?: () => void;
indeterminate?: boolean;
}
type DefaultProps = Partial<IProps>;
const defaultProps: DefaultProps = {
color: 'primary',
disabled: false,
};
const Checkbox: React.FC<IProps> = (props) => {
const {label, color, disabled, name, value, indeterminate, onChange, checked, ...nextProps} = props;
return (
<div className="checkbox-content">
<label className={classNames(
{...nextProps},
'checkbox',
`checkbox-${color}`,
disabled && 'checkbox-disabled',
indeterminate && `checkbox-${color}-indeterminate`
)}
>
<input
type="checkbox"
name={name && name}
value={value && value}
checked={checked}
onChange={onChange}
/>
<span>{label}</span>
</label>
</div>
);
};
Checkbox.defaultProps = defaultProps;
Checkbox.displayName = 'CheckBox';
export default Checkbox;
function Application () {
const [isChecked, setChecked] = React.useState(false);
const handleChange = () => {
setChecked(!isChecked);
};
return (
<div>
<Checkbox label="initial" onChange={handleChange} checked={isChecked} />
</div>
);
}
I dont have any idea, how to do it properly because I want to my component be fully reusable.
name && name
еТМvalue && value
зЪДжДПдєЙжШѓдїАдєИпЉЯ - zenlyname
呢? - Felix