我正在开发一款类似于iPhone上的Amaziograph应用程序,也被称为万花筒或曼陀罗。目前为止,我已经尝试并制作了一个特定的应用程序布局
。我已经扩展了画布并制作了一个自定义画布,在绘制方法中,我将画布分成了9个部分,并在循环中旋转画布并复制其内容。这是我用于上述圆形分割形状的画布类代码。
我无法实现下图所示的功能:
我想在一个框中绘制,然后它应该被复制到其他框中。如何实现这一点?一个小指南将非常有帮助。
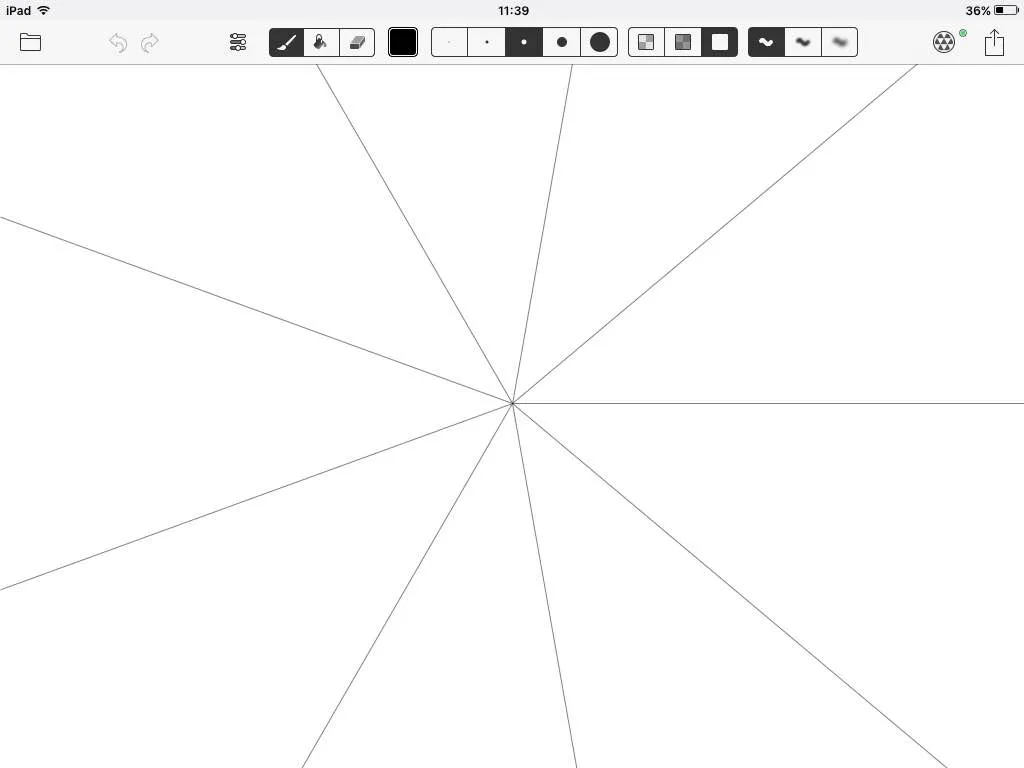
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.graphics.Path;
import android.graphics.PorterDuff;
import android.util.AttributeSet;
import android.view.MotionEvent;
import android.view.View;
import com.madala.mandaladrawing.R;
import com.madala.mandaladrawing.model.DrawingEvent;
import com.madala.mandaladrawing.utils.Common;
public class CanvasView extends View {
private final Context context;
private Bitmap bitmap;
private Canvas bitmapCanvas;
private Paint bitmapPaint;
private Path path = new Path();
private Paint brushPaint;
private int numberOfMirror = 5;
private int cx, cy;
public CanvasView(Context context) {
super(context);
this.context = context;
init();
}
public CanvasView(Context context, AttributeSet attrs) {
super(context, attrs);
this.context = context;
init();
}
public CanvasView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
this.context = context;
init();
}
private void init() {
brushPaint = createPaint();
brushPaint.setColor(0xffffffff);
}
@Override
protected void onDraw(Canvas canvas) {
canvas.drawBitmap(bitmap, 0, 0, bitmapPaint);
canvas.drawPath(path, brushPaint);
for (int i = 1; i < numberOfMirror; i++) {
canvas.rotate(360f / numberOfMirror, cx, cy);
canvas.drawPath(path, brushPaint);
}
}
public void clearCanvas(){
bitmapCanvas.drawColor(0, PorterDuff.Mode.CLEAR);
invalidate();
}
@Override
protected void onSizeChanged(int w, int h, int oldw, int oldh) {
super.onSizeChanged(w, h, oldw, oldh);
setLayerType(View.LAYER_TYPE_HARDWARE, null);
bitmap = Bitmap.createBitmap(w, h, Bitmap.Config.ARGB_8888);
bitmapCanvas = new Canvas(bitmap);
bitmapPaint = new Paint(Paint.DITHER_FLAG);
cx = w / 2;
cy = h / 2;
}
@Override
public boolean onTouchEvent(MotionEvent event) {
float x = event.getX();
float y = event.getY();
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
path.moveTo(x, y);
break;
case MotionEvent.ACTION_MOVE:
path.lineTo(x, y);
break;
case MotionEvent.ACTION_UP:
path.lineTo(x, y);
drawToCanvas(path, brushPaint);
path.reset();
break;
default:
return false;
}
invalidate();
return true;
}
private void drawToCanvas(Path path, Paint brushPaint) {
bitmapCanvas.drawPath(path, brushPaint);
for (int i = 1; i < numberOfMirror; i++) {
bitmapCanvas.rotate(360f / numberOfMirror, cx, cy);
bitmapCanvas.drawPath(path, brushPaint);
}
}
public int getCurrentBrushColor() {
return brushPaint.getColor();
}
public void setCurrentBrushColor(int color) {
brushPaint.setColor(color);
}
private Paint createPaint() {
Paint p = new Paint(Paint.ANTI_ALIAS_FLAG);
p.setStrokeWidth(8f);
p.setStyle(Paint.Style.STROKE);
p.setStrokeJoin(Paint.Join.ROUND);
p.setStrokeCap(Paint.Cap.ROUND);
return p;
}
}
我无法实现下图所示的功能:
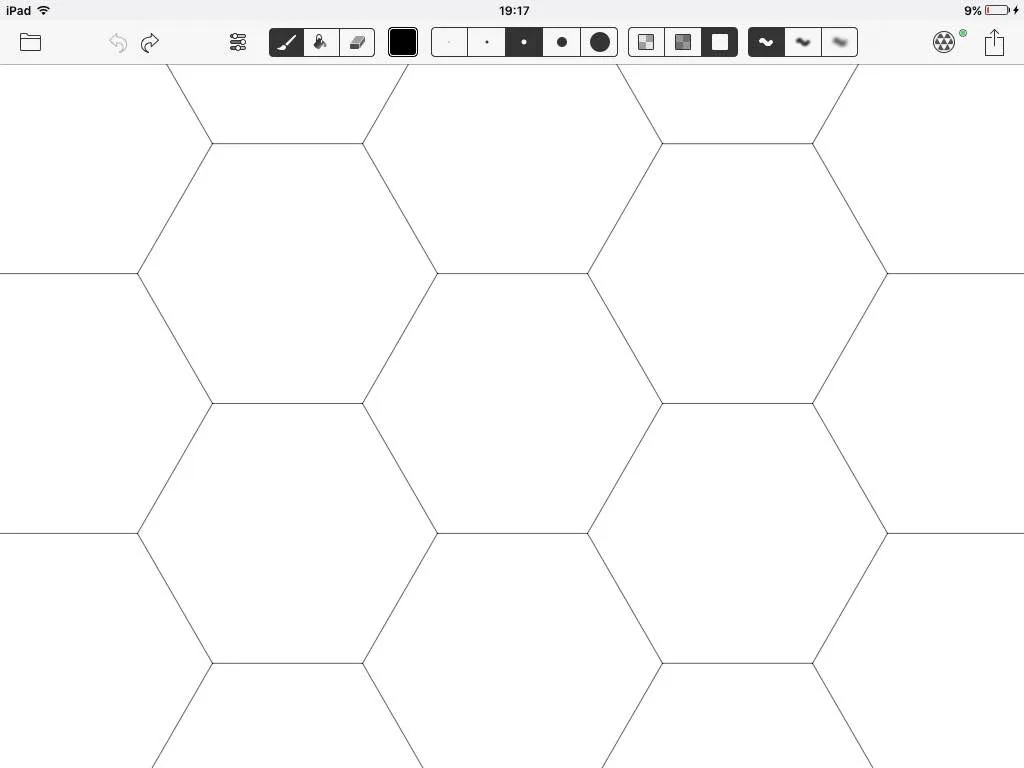
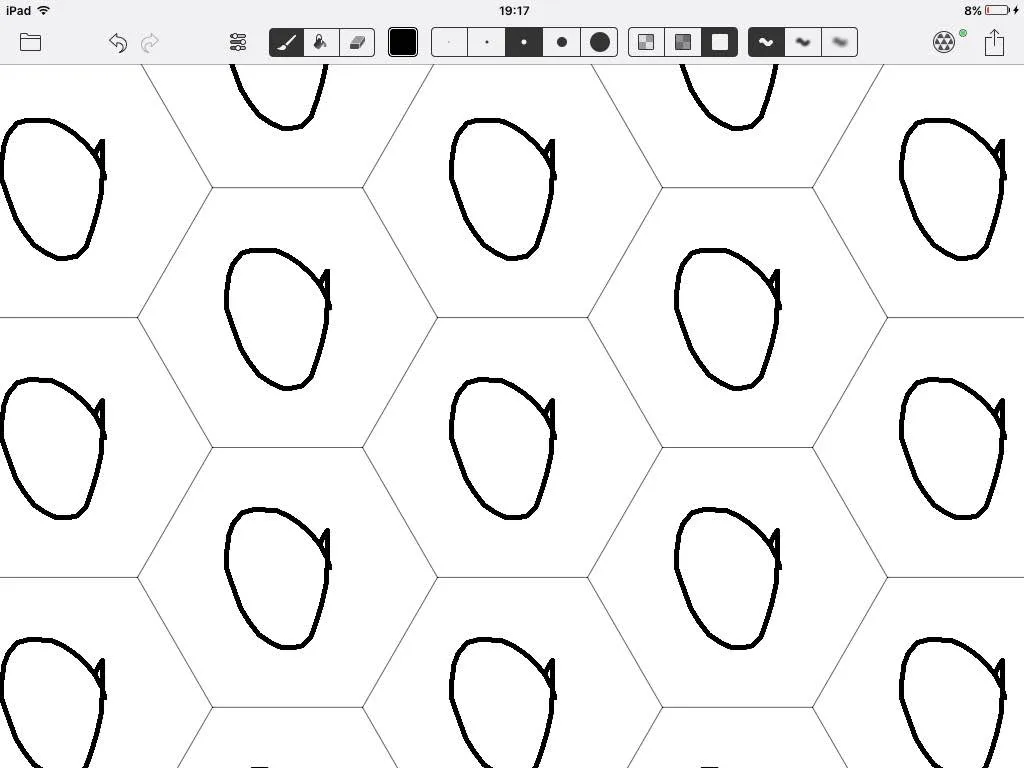
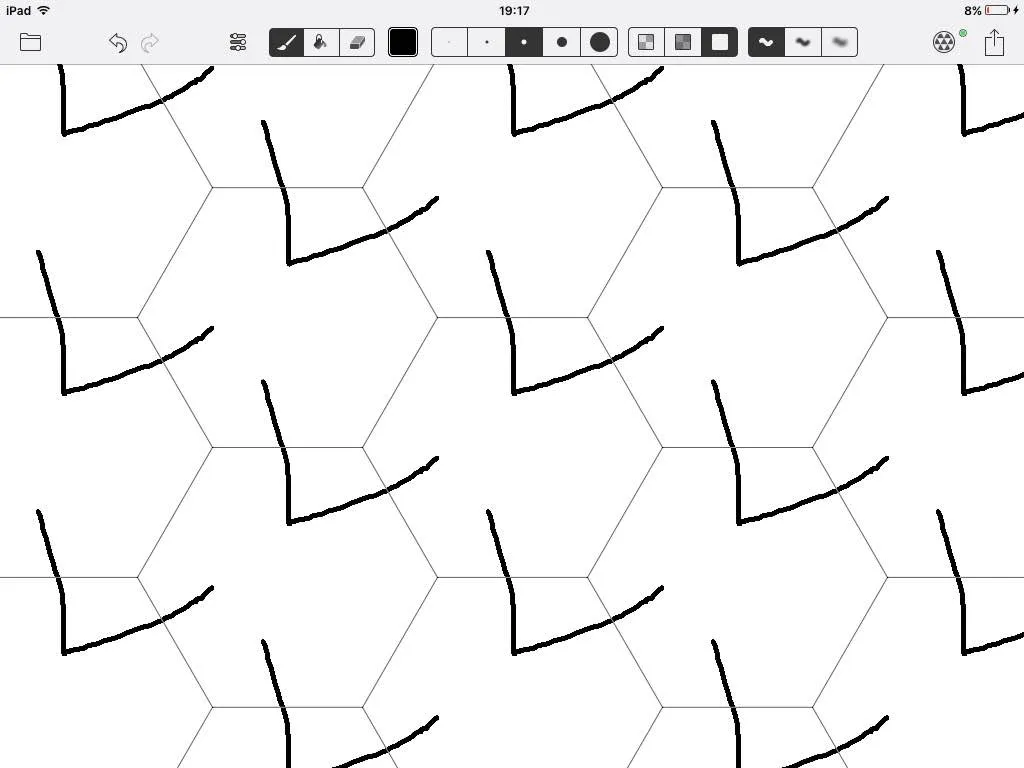