我目前正在尝试使用EF Core创建Web API,并且在连接表格时遇到了一些问题。我正在使用以下数据库图表进行操作:
目前从API获取的数据如下:
如果我使用SQL,我会将用户加入“评论”,但我不能这样做,所以我尝试了一个类似的解决方案,我认为这样可以解决问题。
然而,虽然这个查询确实执行了,但结果与我写的第一个查询相同,问题在于用户没有加入评论。
简而言之,我可以将用户连接到帖子和评论连接到帖子,但我无法将用户绑定到评论。
希望您能帮助我,提前感谢:)
编辑:这是我的模型。
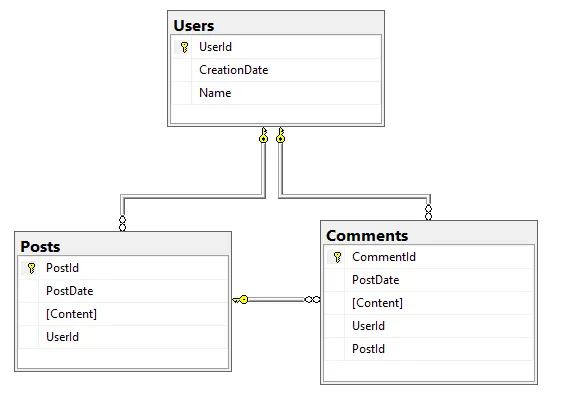
[
{
"postId":1,
"postDate":"2018-10-21T21:56:43.9838536",
"content":"First entry in posts!",
"user":{
"userId":1,
"creationDate":"2018-10-21T21:56:36.3539549",
"name":"Hansel"
},
"comments":[
{
"commentId":1,
"postDate":"0001-01-01T00:00:00",
"content":"Nice!",
"user":null
},
{
"commentId":2,
"postDate":"0001-01-01T00:00:00",
"content":"Cool, here's another comment",
"user":null
},
{
"commentId":3,
"postDate":"0001-01-01T00:00:00",
"content":"and the last one for the night",
"user":null
}
]
},
{
"postId":2,
"postDate":"2018-10-22T21:56:44.0650102",
"content":"Its good to see that its working!",
"user":{
"userId":2,
"creationDate":"2018-10-16T21:56:36.4585213",
"name":"Chris"
},
"comments":[
]
}
]
正如你所看到的,它几乎可以工作了,我的问题在于评论中的空值,我希望能够把用户也取出来,但是出现了某些问题,我不能做到。
我的当前查询语句如下(我正在使用DTO来清理生成的JSON):
var result = from post in context.Posts
join user in context.Users
on post.User.UserId equals user.UserId
join comment in context.Comments
on post.PostId equals comment.Post.PostId into comments
select new PostDTO
{
Content = post.Content,
PostDate = post.PostDate,
User = UserDTO.UserToDTO(user),
Comments = CommentDTO.CommentToDTO(comments.ToList()),
PostId = post.PostId
};
如果我使用SQL,我会将用户加入“评论”,但我不能这样做,所以我尝试了一个类似的解决方案,我认为这样可以解决问题。
var result = from post in context.Posts
join user in context.Users on post.User.UserId equals user.UserId
join comment in
(from u in context.Users
join c in context.Comments
on u.UserId equals c.User.UserId select c)
on post.PostId equals comment.Post.PostId into comments
select new PostDTO
{
Content = post.Content,
PostDate = post.PostDate,
User = UserDTO.UserToDTO(user),
Comments = CommentDTO.CommentToDTO(comments.ToList()),
PostId = post.PostId
};
然而,虽然这个查询确实执行了,但结果与我写的第一个查询相同,问题在于用户没有加入评论。
简而言之,我可以将用户连接到帖子和评论连接到帖子,但我无法将用户绑定到评论。
希望您能帮助我,提前感谢:)
编辑:这是我的模型。
public class Comment
{
public int CommentId { get; set; }
public DateTime PostDate { get; set; }
public string Content { get; set; }
public User User { get; set; }
public Post Post { get; set; }
}
public class User
{
public int UserId { get; set; }
public DateTime CreationDate { get; set; }
public string Name{ get; set; }
public ICollection<Post> Posts { get; set; }
public ICollection<Comment> Comments { get; set; }
}
public class Post
{
public int PostId { get; set; }
public DateTime PostDate { get; set; }
public string Content { get; set; }
public User User { get; set; }
public ICollection<Comment> Comments { get; set; }
}
join
,而是使用导航属性。并且使用 AutoMapper,这样你就可以在一行代码中将实体类投影到 DTO 类。当前的代码会触发客户端评估,因为CommentDTO.CommentToDTO()
无法被翻译成 SQL。 - Gert Arnolduser
变量join user in context.Users on post.User.UserId equals user.UserId
与post.User
相同。如果我们继续下去,post
应该有ICollecton<Comment> Comments
和Comment
应该有User User
,所以你应该只需要使用select
(投影)就可以得出结果。 - Ivan Stoev