我试图学习如何在Java中使用FileReader读取文件,但是我一直遇到错误。我正在使用Eclipse,出现了红色的错误提示,显示"The constructor FileReader(File) is undefined"和"The constructor BufferedReader(FileReader) is undefined",但是我不知道这个错误来自哪里,因为我使用了正确的库和语句。
我得到了以下错误:
我得到了以下错误:
Exception in thread "main" java.lang.Error: Unresolved compilation problems:
The constructor FileReader(File) is undefined
The constructor BufferedReader(FileReader) is undefined
at FileReader.main(FileReader.java:17)
以下是我的代码:
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
public class FileReader {
public static void main(String[] args) {
File file = new File("example.txt");
BufferedReader br = null;
try {
FileReader fr = new FileReader(file);
br = new BufferedReader(fr);
String line;
while( (line = br.readLine()) != null ) {
System.out.println(line);
}
} catch (FileNotFoundException e) {
System.out.println("File not found: " + file.toString());
} catch (IOException e) {
System.out.println("Unable to read file: " + file.toString());
}
finally {
try {
br.close();
} catch (IOException e) {
System.out.println("Unable to close file: " + file.toString());
}
catch(NullPointerException ex) {
}
}
}
}
为了更好的理解(抱歉图片有些大,但我相信您可以缩放。您可以在行左侧看到红色错误的位置):
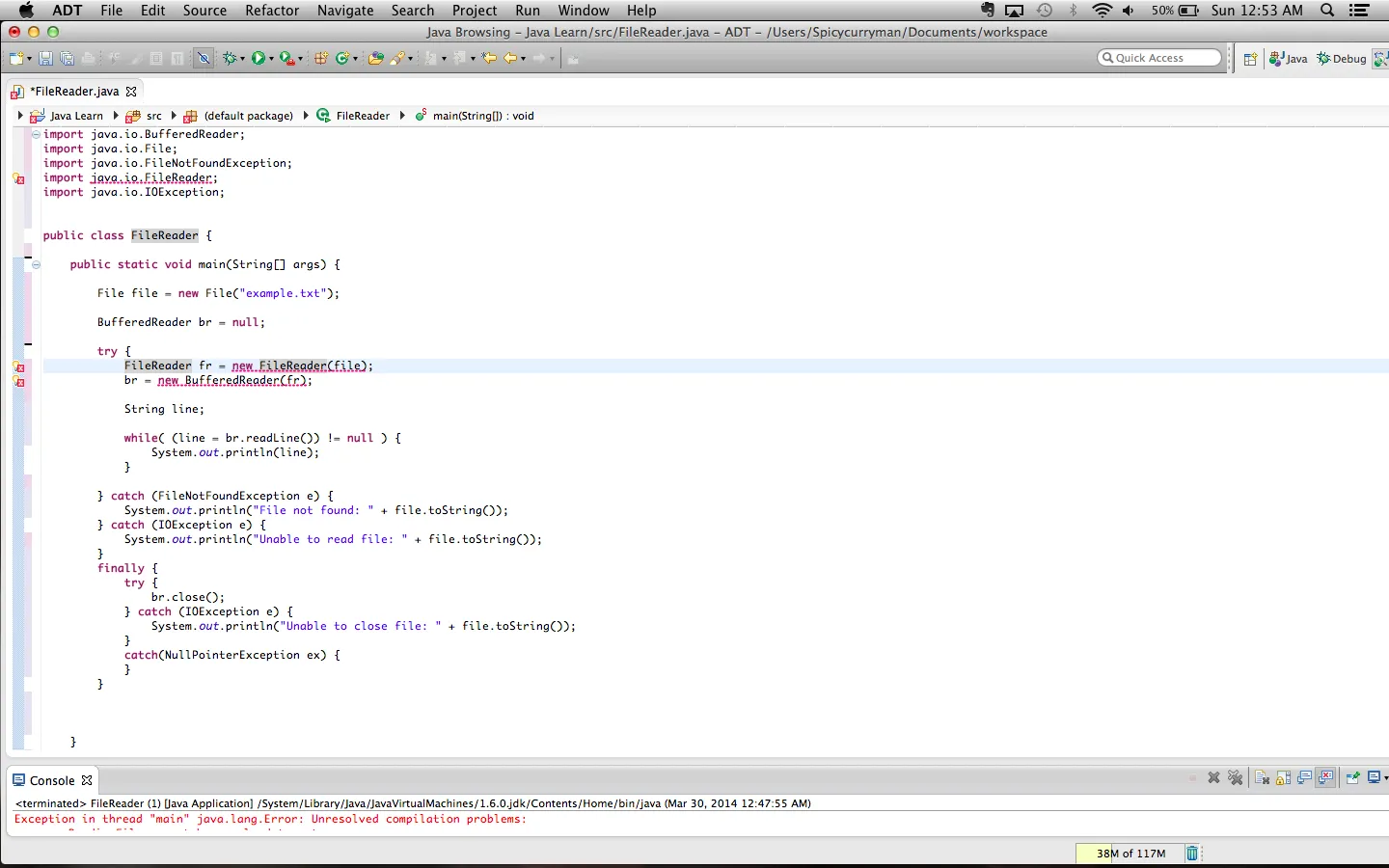