编辑:添加了“亚克力”模糊和色调渲染选项。不过当移动窗口时似乎有点慢。
这是你想要的吗?
运行函数之前的窗口:
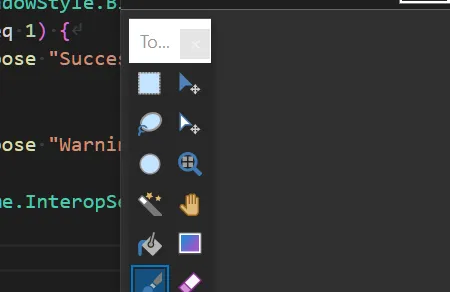
运行函数之后的窗口(Set-WindowBlur -MainWindowHandle 853952 -Enable
):
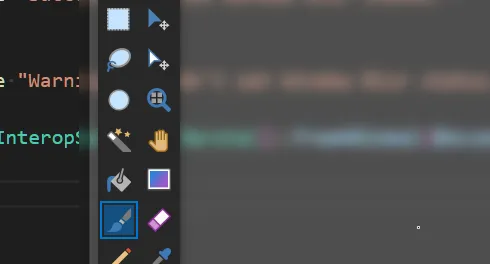
主要代码:
$SetWindowComposition = @'
[DllImport("user32.dll")]
public static extern int SetWindowCompositionAttribute(IntPtr hwnd, ref WindowCompositionAttributeData data);
[StructLayout(LayoutKind.Sequential)]
public struct WindowCompositionAttributeData {
public WindowCompositionAttribute Attribute;
public IntPtr Data;
public int SizeOfData;
}
public enum WindowCompositionAttribute {
WCA_ACCENT_POLICY = 19
}
public enum AccentState {
ACCENT_DISABLED = 0,
ACCENT_ENABLE_BLURBEHIND = 3,
ACCENT_ENABLE_ACRYLICBLURBEHIND = 4
}
[StructLayout(LayoutKind.Sequential)]
public struct AccentPolicy {
public AccentState AccentState;
public int AccentFlags;
public int GradientColor;
public int AnimationId;
}
'@
Add-Type -MemberDefinition $SetWindowComposition -Namespace 'WindowStyle' -Name 'Blur'
function Set-WindowBlur {
[CmdletBinding()]
param (
[Parameter(Mandatory)]
[int]
$MainWindowHandle,
[Parameter(ParameterSetName='Enable',Mandatory)]
[switch]
$Enable,
[Parameter(ParameterSetName='Acrylic',Mandatory)]
[switch]
$Acrylic,
[Parameter(ParameterSetName='Acrylic')]
[ValidateRange(0x000000, 0xFFFFFF)]
[int]
$Color= 0x000000,
[Parameter(ParameterSetName='Acrylic')]
[ValidateRange(0, 255)]
[int]
$Transparency = 80,
[Parameter(ParameterSetName='Disable',Mandatory)]
[switch]
$Disable
)
$Accent = [WindowStyle.Blur+AccentPolicy]::new()
switch ($PSCmdlet.ParameterSetName) {
'Enable' {
$Accent.AccentState = [WindowStyle.Blur+AccentState]::ACCENT_ENABLE_BLURBEHIND
}
'Acrylic' {
$Accent.AccentState = [WindowStyle.Blur+AccentState]::ACCENT_ENABLE_ACRYLICBLURBEHIND
$Accent.GradientColor = $Transparency -shl 24 -bor ($Color -band 0xFFFFFF)
}
'Disable' {
$Accent.AccentState = [WindowStyle.Blur+AccentState]::ACCENT_DISABLED
}
}
$AccentStructSize = [System.Runtime.InteropServices.Marshal]::SizeOf($Accent)
$AccentPtr = [System.Runtime.InteropServices.Marshal]::AllocHGlobal($AccentStructSize)
[System.Runtime.InteropServices.Marshal]::StructureToPtr($Accent,$AccentPtr,$false)
$Data = [WindowStyle.Blur+WindowCompositionAttributeData]::new()
$Data.Attribute = [WindowStyle.Blur+WindowCompositionAttribute]::WCA_ACCENT_POLICY
$Data.SizeOfData = $AccentStructSize
$Data.Data = $AccentPtr
$Result = [WindowStyle.Blur]::SetWindowCompositionAttribute($MainWindowHandle,[ref]$Data)
if ($Result -eq 1) {
Write-Verbose "Successfully set Window Blur status."
}
else {
Write-Verbose "Warning, couldn't set Window Blur status."
}
[System.Runtime.InteropServices.Marshal]::FreeHGlobal($AccentPtr)
}
使用时可用如下方式调用:
Set-WindowBlur -MainWindowHandle 1114716 -Acrylic -Color 0xFF0000 -Transparency 50
Set-WindowBlur -MainWindowHandle 1114716 -Disable
Set-WindowBlur -MainWindowHandle 1114716 -Enable
翻译自:https://gist.github.com/riverar/fd6525579d6bbafc6e48
和:
https://github.com/riverar/sample-win32-acrylicblur/blob/master/MainWindow.xaml.cs