我正在使用 Spring Data JPA
中的 CrudRepository
检索数据。我想要从我的自定义查询中检索到的记录进行过滤。我尝试使用 .setMaxResults(20);
来选择前 20
行。但是出现了错误。我想要从我的表中筛选出前 20
行。
这是我的存储库
package lk.slsi.repository;
import java.util.Date;
import lk.slsi.domain.SLSNotification;
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.CrudRepository;
import org.springframework.data.repository.query.Param;
import java.util.List;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
/**
* Created by ignotus on 2/10/2017.
*/
public interface SLSNotificationRepository extends CrudRepository<SLSNotification, Integer> {
@Override
SLSNotification save(SLSNotification slsNotification);
@Override
SLSNotification findOne(Integer snumber);
@Override
long count();
@Override
void delete(Integer integer);
@Override
void delete(SLSNotification slsNotification);
@Override
void delete(Iterable<? extends SLSNotification> iterable);
@Override
List<SLSNotification> findAll();
@Query("select a from SLSNotification a where a.slsiUnit in :unitList order by snumber desc")
List<SLSNotification> getApplicationsByUnit(@Param("unitList") List<String> unitList);
@Query("select a from SLSNotification a where a.userId = :userId")
List<SLSNotification> getApplicationsByUserId(@Param("userId") String userId);
@Query("select a.snumber, a.date, a.slsNo, a.slsiUnit, a.productDesc, a.status from SLSNotification a where a.userId = :userId ORDER BY snumber desc")
List<SLSNotification> getApplicationsByUserIdforManage(@Param("userId") String userId);
@Query("select a from SLSNotification a where a.slsNo = :slsNo")
SLSNotification getApplicationBySLSNumber(@Param("slsNo") String slsNo);
}
我希望我的List<SLSNotification> getApplicationsByUserIdforManage(@Param("userId") String userId);
方法检索有限的数据集。我该如何调用实体管理器或其他任何东西来完成此操作?请帮我做到这一点。
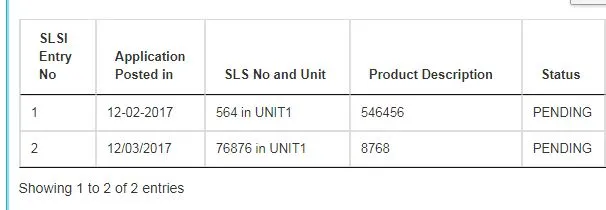
limit
关键字呢? - Shafin Mahmud