我目前正在尝试使用新的Android Support Design库中的各种新组件。我已经在我的MainActivity.java
中实现了一个NavigationView
,使用FragmentManager在导航抽屉中的项目之间进行导航:
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.content, mTabLayoutFragment)
.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN)
.commit();
我在一个碎片中使用了 TabLayout
。下面是该碎片的布局:
<android.support.design.widget.AppBarLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.design.widget.TabLayout
android:id="@+id/tabLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:tabMode="fixed"
app:tabGravity="fill"
app:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"/>
<android.support.v4.view.ViewPager
android:id="@+id/viewpager"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@android:color/white"/>
</android.support.design.widget.AppBarLayout>
而其背后的 Java 代码为:
import android.os.Bundle;
import android.support.design.widget.*;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentStatePagerAdapter;
import android.support.v4.view.ViewPager;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class TabLayoutFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View inflatedView = inflater.inflate(R.layout.fragment_tablayout, container, false);
TabLayout tabLayout = (TabLayout) inflatedView.findViewById(R.id.tabLayout);
tabLayout.addTab(tabLayout.newTab().setText("Campusplan"));
tabLayout.addTab(tabLayout.newTab().setText("Raumplan"));
final ViewPager viewPager = (ViewPager) inflatedView.findViewById(R.id.viewpager);
viewPager.setAdapter(new PagerAdapter
(getFragmentManager(), tabLayout.getTabCount()));
viewPager.addOnPageChangeListener(new TabLayout.TabLayoutOnPageChangeListener(tabLayout));
tabLayout.setOnTabSelectedListener(new TabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabLayout.Tab tab) {
viewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {
}
@Override
public void onTabReselected(TabLayout.Tab tab) {
}
});
return inflatedView;
}
public class PagerAdapter extends FragmentStatePagerAdapter {
int mNumOfTabs;
public PagerAdapter(FragmentManager fm, int NumOfTabs) {
super(fm);
this.mNumOfTabs = NumOfTabs;
}
@Override
public Fragment getItem(int position) {
switch (position) {
case 0:
TabItem1 tab1 = new TabItem1();
return tab1;
case 1:
TabItem1 tab2 = new TabItem1();
return tab2;
default:
return null;
}
}
@Override
public int getCount() {
return mNumOfTabs;
}
}
}
请注意
TabItem1
和 TabItem1
,它们是由仅包含 TextView 的片段构成。这两个片段将显示在 TabLayout 中。现在,这段代码似乎在某种程度上是有效的。它看起来像这样:
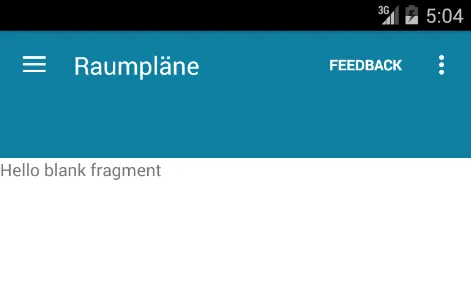
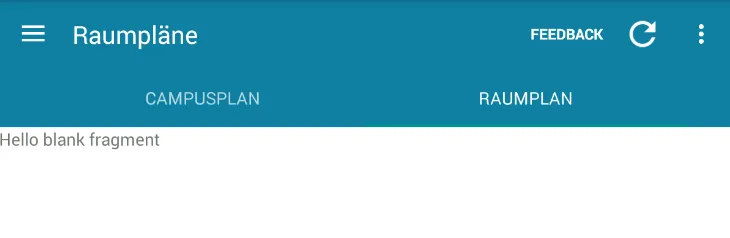
AndroidManifest
中有以下内容:android:configChanges="orientation|screenSize"