我可以帮您进行翻译。以下是需要翻译的内容:
基本上,一个垂直滚动视图(ScrollViewB)位于另一个垂直滚动视图(ScrollViewA)内部。ProfileOverviewViewController和ProfileDetailViewController都与设备屏幕大小相同,因此只有在ScrollViewA滚动到底部后,ScrollViewB才可见。
ScrollViewA启用了分页功能,因此会切换到占据整个屏幕视图的ProfileOverviewViewController或ProfileDetailViewController。
希望这张图能让布局更加清晰:
我的问题是:
如何在ScrollViewB滚动到顶部时将滚动传递给ScrollViewA?
谢谢。
我有一个接口,其结构如下,其中重要元素的名称用括号括起来:
- UIViewController
- UIScrollView (ScrollViewA)
- UIViewController (ProfileOverviewViewController)
- UIViewController (ProfileDetailViewController)
- UICollectionView (ScrollViewB)
基本上,一个垂直滚动视图(ScrollViewB)位于另一个垂直滚动视图(ScrollViewA)内部。ProfileOverviewViewController和ProfileDetailViewController都与设备屏幕大小相同,因此只有在ScrollViewA滚动到底部后,ScrollViewB才可见。
ScrollViewA启用了分页功能,因此会切换到占据整个屏幕视图的ProfileOverviewViewController或ProfileDetailViewController。
希望这张图能让布局更加清晰:
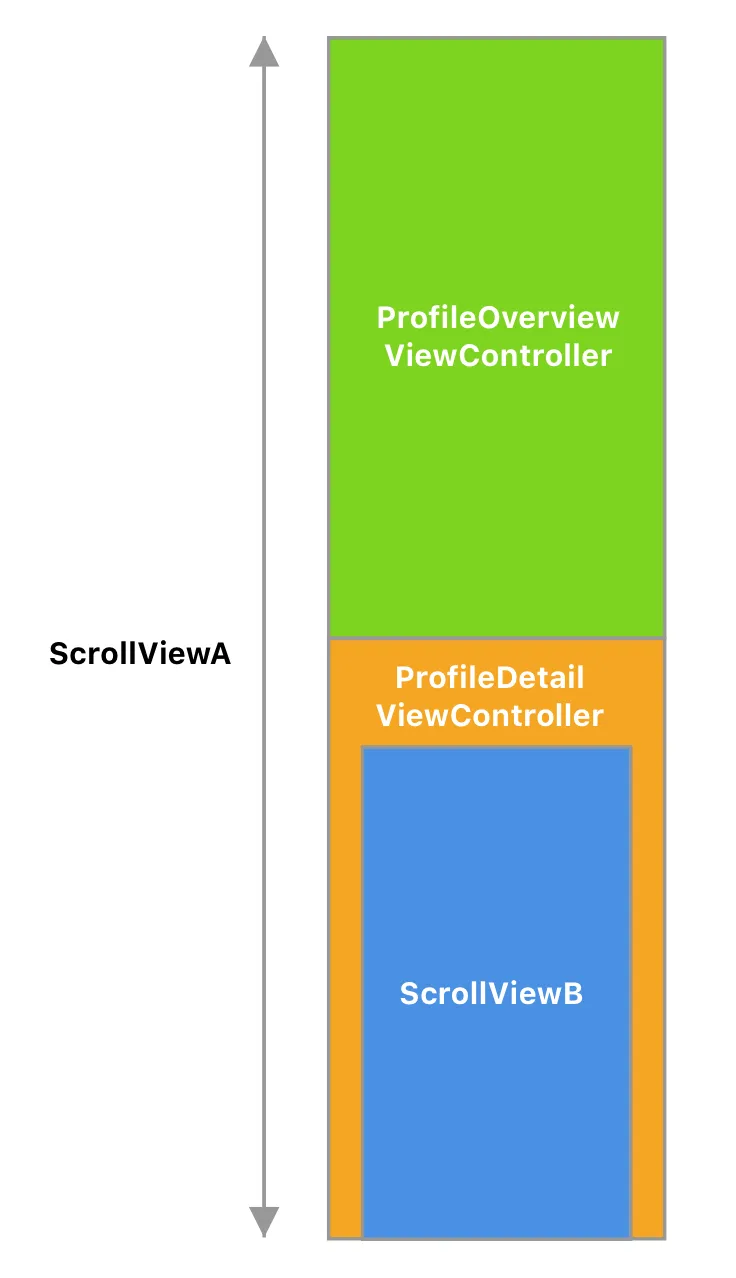
- 如果用户滚动到ScrollViewA的底部,使ProfileDetailViewController和ScrollViewB可见。
- 用户在ScrollViewB上向下滚动一点,然后释放手指。
- 然后用户在ScrollViewB上向上滚动。
- 当用户在ScrollViewB中的内容顶部仍然按住手指时,ScrollViewB应停止滚动,并且ScrollViewA应该开始向上滚动,直至到达ProfileOverviewViewController,所有这些都在同一个手势中进行。
如何在ScrollViewB滚动到顶部时将滚动传递给ScrollViewA?
谢谢。