我正在尝试在父组件内的特定位置(基于某些计算)呈现多个子组件。给出垂直位置的计算看起来正确,但是组件未显示在应该显示的位置上。我已经尝试了绝对窗口位置和相对组件位置,但都没有成功。
父组件如下所示:
我能看到
我最初的想法是我某种方式弄乱了样式,我还尝试给每个元素赋予不同的
父组件如下所示:
const top = 170;
const bottom = 10;
const left = 10;
const right = 10;
const styles = StyleSheet.create({
grid: {
flex: 1,
position: 'absolute',
top: top,
height: Dimensions.get('window').height - top - bottom,
width: Dimensions.get('window').width - left - right,
borderLeftColor: 'black',
borderLeftWidth: 1,
borderBottomColor: 'black',
borderBottomWidth: 1
}
});
const DrawGrid: React.FC<IDrawGrid> = ({ maxDistance, elements }) => {
const [gridSize, setGridSize] = useState<LayoutRectangle>();
return (
<View style={styles.grid} onLayout={(event) => {
setGridSize(event.nativeEvent.layout);
}}>
{elements.map((element, index) => {
return (
<DrawElement element={element} maxDistance={maxDistance} gridSize={gridSize} index={index * 2} />
)
})}
</View>
);
};
渲染所有元素的子组件如下所示:
const top = 170;
const bottom = 20;
const left = 10;
const right = 10;
const styles = StyleSheet.create({
elementContainer: {
borderLeftColor: 'red',
borderLeftWidth: 1,
borderTopColor: 'red',
borderTopWidth: 1,
borderRightColor: 'red',
borderRightWidth: 1,
borderBottomColor: 'red',
borderBottomWidth: 1,
borderRadius: 5,
padding: 2,
position: 'relative',
alignSelf: 'flex-start'
}
});
const getVerticalPosition = (someDistance: number, maxDistance: number, height: number) => {
if (!someDistance || !maxDistance) return { top: 0 };
const topDistance = (1 - (someDistance / maxDistance)) * height;
return { top: topDistance };
};
const DrawElement: React.FC<IDrawElement> = ({ maxDistance, element, gridSize, index }) => {
const styleVertical = getVerticalPosition(someDistance, maxDistance, gridSize.height);
return (
<View key={key} style={[styles.elementContainer, styleVertical]}>
<Text>top: {styleVertical.top.toFixed(2)}</Text>
</View>
);
};
我能看到
getVerticalPosition
返回了正确的值,但元素从未出现在预期位置。在下面的快照中,我打印了每个元素的顶部值,我们可以看到它根本没有被尊重。(水平位置超出了问题的范围)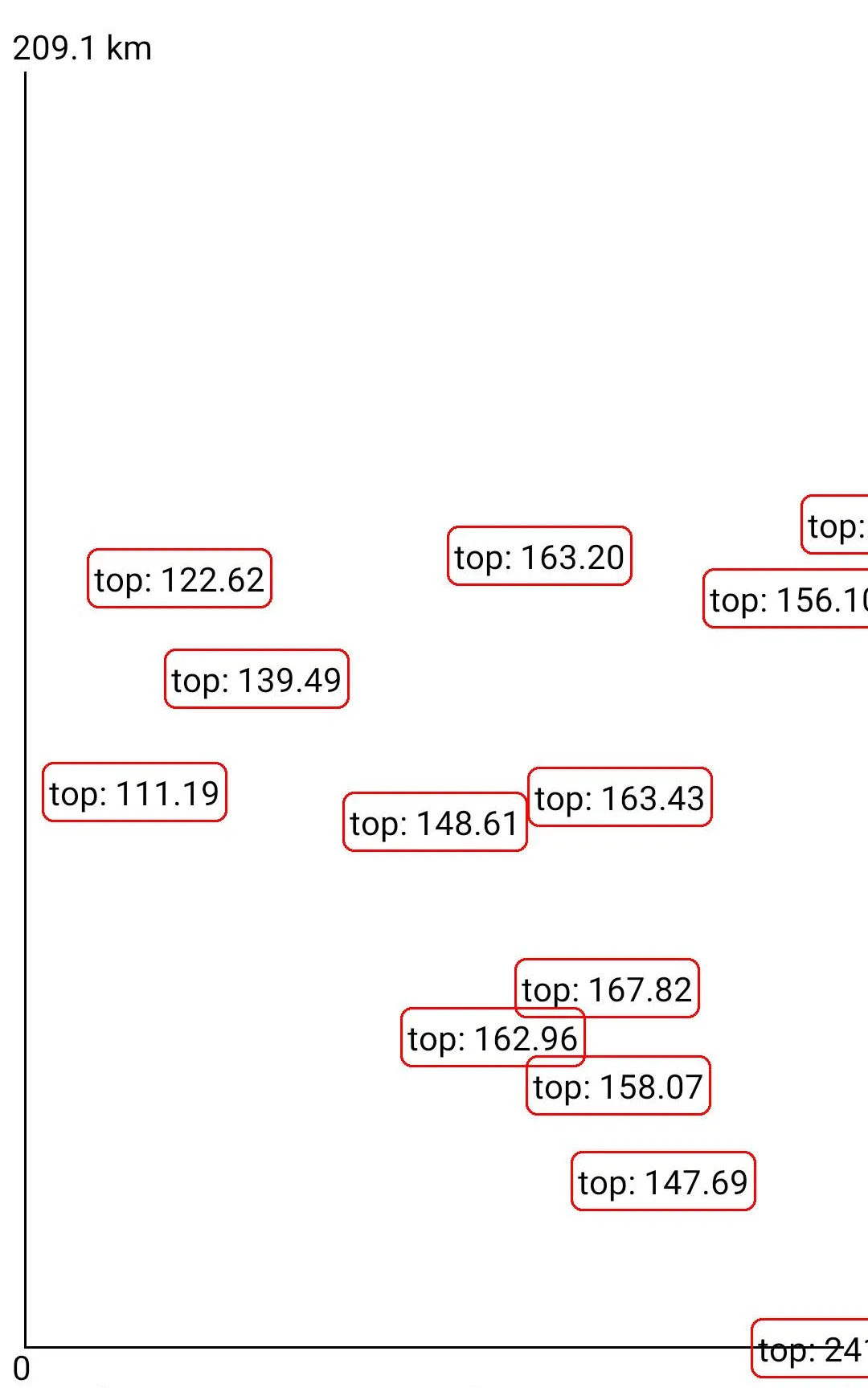
zindex
,但都没有成功。有什么想法吗?任何帮助将不胜感激,谢谢!