好的,这个答案有点晚了,但我最近注意到我的原始问题有一些活动(并且没有提供有效的解决方案),所以我想给你最终对我有用的东西。
我将我的答案分为三个部分:
背景
(此部分对解决方案不重要)
我的原始问题是我有很多图片(即巨量),这些图片被单独存储在数据库中,以字节数组的形式存在。我想用所有这些图片制作一个视频序列。
我的设备设置大致如下图所示:
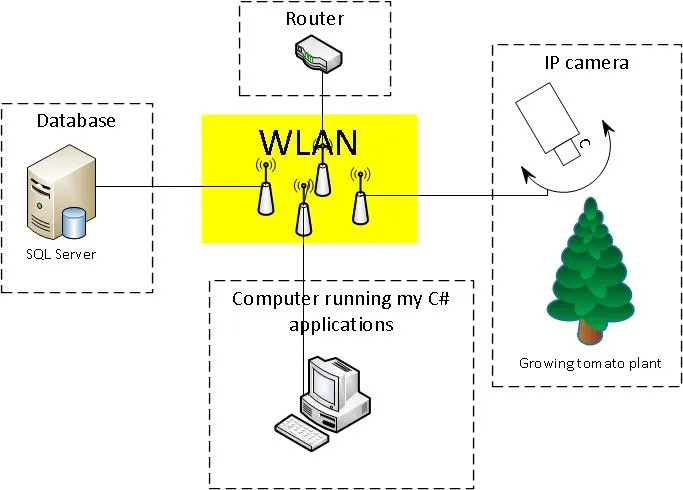
这些图片描绘了处于不同状态下的生长中的番茄植物。所有图片都是在白天每隔1分钟拍摄的。
while (true)
{
if (daylight)
{
}
}
我有一个非常简单的数据库来存储图像,里面只有一个表(ImageSet表):
问题
我已经阅读了许多关于ffmpeg的文章(请参见我的原始问题),但我找不到任何关于如何从一组图像转换为视频的文章。
解决方案
最终,我找到了一个可行的解决方案!其中主要部分来自于开源项目AForge.NET。简单来说,你可以说AForge.NET是一个用C#编写的计算机视觉和人工智能库。(如果你想要这个框架的副本,只需从http://www.aforgenet.com/获取即可)
在AForge.NET中,有一个名为VideoFileWriter类(使用ffmpeg编写视频文件的类)。这几乎完成了所有的工作。(这里还有一个非常好的例子here)
这是我用来从我的图像数据库中获取和转换图像数据为视频的最终类(精简版):
public class MovieMaker
{
public void Start()
{
var startDate = DateTime.Parse("12 Mar 2012");
var endDate = DateTime.Parse("13 Aug 2012");
CreateMovie(startDate, endDate);
}
public Bitmap ToBitmap(byte[] byteArrayIn)
{
var ms = new System.IO.MemoryStream(byteArrayIn);
var returnImage = System.Drawing.Image.FromStream(ms);
var bitmap = new System.Drawing.Bitmap(returnImage);
return bitmap;
}
public Bitmap ReduceBitmap(Bitmap original, int reducedWidth, int reducedHeight)
{
var reduced = new Bitmap(reducedWidth, reducedHeight);
using (var dc = Graphics.FromImage(reduced))
{
dc.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.HighQualityBicubic;
dc.DrawImage(original, new Rectangle(0, 0, reducedWidth, reducedHeight), new Rectangle(0, 0, original.Width, original.Height), GraphicsUnit.Pixel);
}
return reduced;
}
private void CreateMovie(DateTime startDate, DateTime endDate)
{
int width = 320;
int height = 240;
var framRate = 200;
using (var container = new ImageEntitiesContainer())
{
var query = from d in container.ImageSet
where d.Date >= startDate && d.Date <= endDate
select d;
using (var vFWriter = new VideoFileWriter())
{
vFWriter.Open("nameOfMyVideoFile.avi", width, height, framRate, VideoCodec.Raw);
var imageEntities = query.ToList();
foreach (var imageEntity in imageEntities)
{
var imageByteArray = imageEntity.Data;
var bmp = ToBitmap(imageByteArray);
var bmpReduced = ReduceBitmap(bmp, width, height);
vFWriter.WriteVideoFrame(bmpReduced);
}
vFWriter.Close();
}
}
}
}
更新于2013年11月29日(如何)(希望这是您所要求的,@Kiquenet?)
- 从下载页面下载AForge.NET Framework(下载完整的ZIP压缩包,您将在
AForge.NET Framework-2.2.5\Samples文件夹
中找到许多有趣的Visual Studio解决方案和项目,例如视频...)
- 命名空间:
AForge.Video.FFMPEG
(来自文档)
- 程序集:
AForge.Video.FFMPEG
(在AForge.Video.FFMPEG.dll
中)(来自文档)(您可以在AForge.NET Framework-2.2.5\Release
文件夹中找到此AForge.Video.FFMPEG.dll
)
如果你想创建自己的解决方案,请确保在项目中引用了AForge.Video.FFMPEG.dll
。然后,使用VideoFileWriter类应该很容易。如果您按照link到类的链接,您将找到一个非常好(而简单)的示例。在代码中,他们使用for
循环将Bitmap image
提供给VideoFileWriter。