你可以使用JsonConverter来实现。
例如,当你从第三方服务中获取一些数据时,它们经常更改属性名称,然后又返回到之前的属性名称,这时就很有用了。 :D
以下代码展示了如何从多个属性名称反序列化到同一个类属性,并使用[JsonProperty(PropertyName = "EnrollmentStatusEffectiveDateStr")]
属性进行修饰。
类MediCalFFSPhysician
也使用自定义的JsonConverter进行修饰:[JsonConverter(typeof(MediCalFFSPhysicianConverter))]
请注意,_propertyMappings
字典包含应映射到属性EnrollmentStatusEffectiveDateStr
的可能属性名称:
private readonly Dictionary<string, string> _propertyMappings = new()
{
{"Enrollment_Status_Effective_Dat", "EnrollmentStatusEffectiveDateStr"},
{"Enrollment_Status_Effective_Date", "EnrollmentStatusEffectiveDateStr"},
{"USER_Enrollment_Status_Effectiv", "EnrollmentStatusEffectiveDateStr"}
};
完整代码:
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
using Newtonsoft.Json.Serialization;
using System.Reflection;
using System.Text.Json;
internal class JSONDeserialization
{
private static void Main(string[] args)
{
var jsonPayload1 = $"{{\"Enrollment_Status_Effective_Dat\":\"2022/10/13 19:00:00+00\"}}";
var jsonPayload2 = $"{{\"Enrollment_Status_Effective_Date\":\"2022-10-13 20:00:00+00\"}}";
var jsonPayload3 = $"{{\"USER_Enrollment_Status_Effectiv\":\"2022-10-13 21:00:00+00\"}}";
var deserialized1 = JsonConvert.DeserializeObject<MediCalFFSPhysician>(jsonPayload1);
var deserialized2 = JsonConvert.DeserializeObject<MediCalFFSPhysician>(jsonPayload2);
var deserialized3 = JsonConvert.DeserializeObject<MediCalFFSPhysician>(jsonPayload3);
Console.WriteLine(deserialized1.Dump());
Console.WriteLine(deserialized2.Dump());
Console.WriteLine(deserialized3.Dump());
Console.ReadKey();
}
}
public class MediCalFFSPhysicianConverter : JsonConverter
{
private readonly Dictionary<string, string> _propertyMappings = new()
{
{"Enrollment_Status_Effective_Dat", "EnrollmentStatusEffectiveDateStr"},
{"Enrollment_Status_Effective_Date", "EnrollmentStatusEffectiveDateStr"},
{"USER_Enrollment_Status_Effectiv", "EnrollmentStatusEffectiveDateStr"}
};
public override bool CanWrite => false;
public override void WriteJson(JsonWriter writer, object value, Newtonsoft.Json.JsonSerializer serializer)
{
throw new NotImplementedException();
}
public override bool CanConvert(Type objectType)
{
return objectType.GetTypeInfo().IsClass;
}
public override object ReadJson(JsonReader reader, Type objectType, object existingValue, Newtonsoft.Json.JsonSerializer serializer)
{
object instance = Activator.CreateInstance(objectType);
var props = objectType.GetTypeInfo().DeclaredProperties.ToList();
JObject jo = JObject.Load(reader);
foreach (JProperty jp in jo.Properties())
{
if (!_propertyMappings.TryGetValue(jp.Name, out var name))
name = jp.Name;
PropertyInfo prop = props.FirstOrDefault(pi =>
pi.CanWrite && pi.GetCustomAttribute<JsonPropertyAttribute>().PropertyName == name);
prop?.SetValue(instance, jp.Value.ToObject(prop.PropertyType, serializer));
}
return instance;
}
}
[JsonConverter(typeof(MediCalFFSPhysicianConverter))]
public class MediCalFFSPhysician
{
[JsonProperty(PropertyName = "EnrollmentStatusEffectiveDateStr")]
public string EnrollmentStatusEffectiveDateStr { get; set; }
}
public static class ObjectExtensions
{
public static string Dump(this object obj)
{
try
{
return System.Text.Json.JsonSerializer.Serialize(obj, new JsonSerializerOptions { WriteIndented = true });
}
catch (Exception)
{
return string.Empty;
}
}
}
输出结果如下:
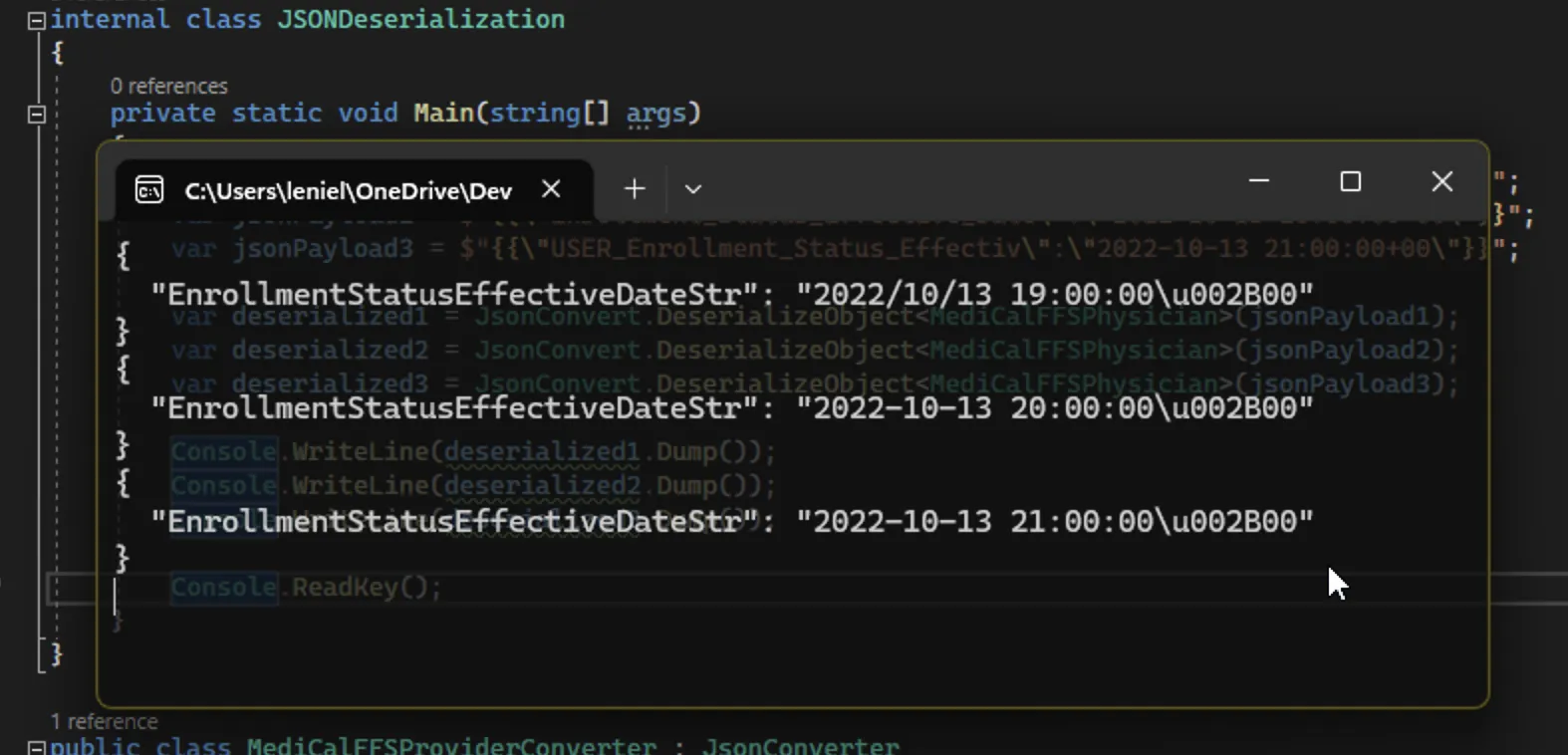
摘自:将不同的JSON结构反序列化为同一个C#类