我曾花费数周的时间来解决这个问题,直到我成功让我的iOS应用程序在任何状态下都能收到通知。
首先,您必须在Xcode中勾选“后台获取”和“后台处理”模式。这些可以在签名和功能下找到。
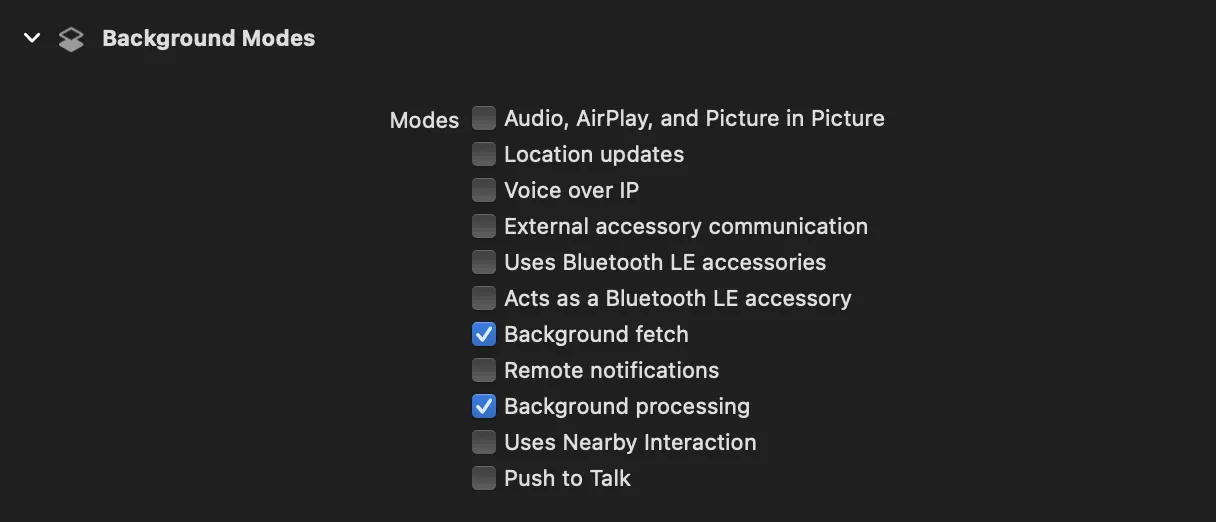
接下来,您必须在AppDelegate.swift中注册您的任务并为您的应用程序提供通知功能。
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
) -> Bool {
GeneratedPluginRegistrant.register(with: self)
UNUserNotificationCenter.current().delegate = self
WorkmanagerPlugin.setPluginRegistrantCallback { registry in
GeneratedPluginRegistrant.register(with: registry)
}
WorkmanagerPlugin.registerTask(withIdentifier: "your.task.identifier")
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
override func userNotificationCenter(
_ center: UNUserNotificationCenter,
willPresent notification: UNNotification,
withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
completionHandler(.alert)
}
}
您还需要在 info.plist 中注册您的任务,如下所示:
<key>BGTaskSchedulerPermittedIdentifiers</key>
<array>
<string>your.task.identifier/string>
</array>
<key>UIBackgroundModes</key>
<array>
<string>fetch</string>
<string>processing</string>
</array>
registerPeriodicTask在iOS上不起作用,因此您应该使用flutter_local_notifications的periodicallyShow函数。
为了参考,我提供了我的代码片段,展示了我如何使用workmanager和flutter_local_notifications;
void registerWorkmanagerTask() async {
try {
await Workmanager().registerOneOffTask(
AppConstants.notifyReviewTask,
AppConstants.notifyReviewTask,
initialDelay: Duration(hours: 4),
existingWorkPolicy: ExistingWorkPolicy.replace,
backoffPolicy: BackoffPolicy.linear,
);
} catch (e) {
print("exception caught $e");
}
}
@pragma('vm:entry-point')
void callbackDispatcher() {
Workmanager().executeTask((taskName, _) async {
try {
await NotificaitonService().setup();
if (taskName == AppConstants.notifyReviewTask)
await NotificaitonService().showNotification(
body: 'items are ready to be reviewed',
notificationId: AppConstants.recallNotificationId,
channel: AppConstants.recallChannel,
title: 'Time to review',
);
return Future.value(true);
} catch (e) {
print("exception caught $e");
}
});
}
请记住,iOS设备可以因多种原因(例如低电量)决定延迟运行后台工作管理器任务。此外,通知不会在iOS模拟器中显示。