我曾使用WPF开发了两个中等规模的应用程序,WPF的简洁性和功能给我留下了深刻的印象。当我向一位业务应用程序员同事解释WPF的各种优点时,他提出了一个让我完全不知所措的问题:
问题:
他用以下方式在约2分钟内编写了一个应用程序:
- 打开新的WinForms项目。
- 定义一个名为
Loan
的类。 - 构建项目。
- 使用
Loan
定义一个对象数据源。 - 在“数据源资源管理器”中将
Loan
数据源的视图类型更改为“详细信息”。 - 在设计器中将数据源拖放到窗体上。
- 使用包含一个对象的
Loan[]
来提供数据源。 - 构建并运行应用程序。
代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WinForms_DataBinding_Example
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
protected override void OnLoad(EventArgs e)
{
base.OnLoad(e);
loanBindingSource.DataSource = new Loan[] { new Loan() };
}
}
public class Loan
{
public decimal Amount { get; set; }
public decimal Rate { get; set; }
public decimal Total { get { return Amount * Rate; } }
}
}
设计师:
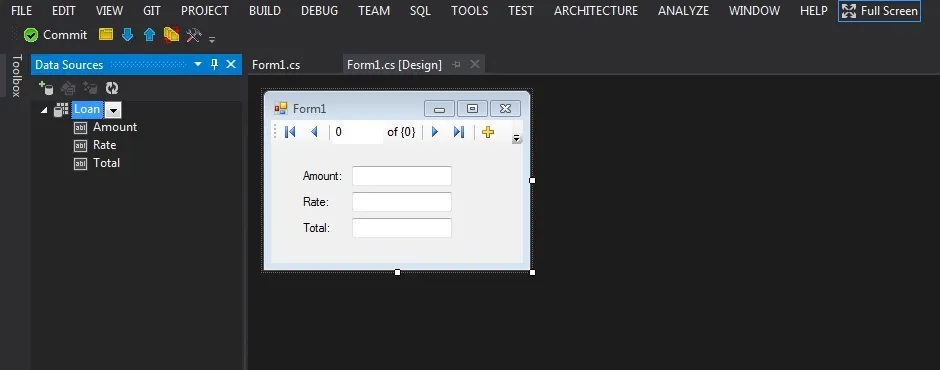
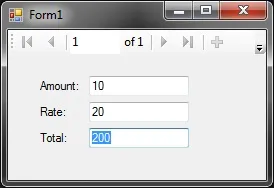
Amount
或Rate
的值时,Total
的值会相应地更改。设计师解释说,这是业务应用程序中非常有用的功能,在实体的一个属性发生任何更改时,立即更新视图,计算属性也会立即刷新,从而提高用户体验。考虑到典型的业务实体类具有许多属性,这样可以节省大量编码时间。然后他让我在WPF中做同样的事情。我首先向他解释了我不明白这里进行了什么黑魔法。
Total
文本框如何自动更新?这是我的第一个问题:Q1.
Loan
类没有实现INotifyPropertyChanged
或类似的东西。那么,当Amount
或Rate
文本框失去焦点时,Total
文本框如何更新?然后我告诉他,我不知道如何在WPF中轻松完成同样的事情。但是,我在UI中使用了3个
TextBlock
和3个TextBox
编写了相同的应用程序。我还需要让Loan
类实现INotifyPropertyChanged
。为Amount
和Rate
添加了备份字段。每当设置这些属性时,我都会为属性Total
引发一次属性更改通知。最后,我得到了一个控件排列不好但与WinForms应用程序完成相同任务的应用程序。然而,这比WinForms方法要难得多。我回家后想到了个好主意,将
Loan
数据源拖放到WPF窗口上(在将视图模式更改为详细信息后)。果然,我得到了与WinForms应用程序相同类型的UI,并在将数据源设置为与WinForms应用程序相同的Loan[]
后,看起来已经完成。我运行了应用程序,更改了Amount
和Rate
字段,希望看到Total
自动更改。但是,我很失望,Total
字段没有更改:
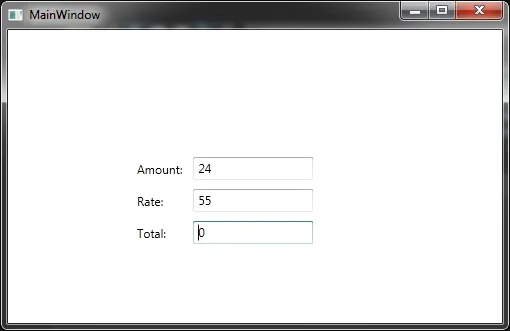
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using WinForms_DataBinding_Example;
namespace WPF_Grid_Example
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void Window_Loaded_1(object sender, RoutedEventArgs e)
{
System.Windows.Data.CollectionViewSource loanViewSource = ((System.Windows.Data.CollectionViewSource)(this.FindResource("loanViewSource")));
// Load data by setting the CollectionViewSource.Source property:
loanViewSource.Source = new List<Loan>() { new Loan() };
}
}
}
:
<Window
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:WinForms_DataBinding_Example="clr-namespace:WinForms_DataBinding_Example;assembly=WinForms_DataBinding_Example" mc:Ignorable="d" x:Class="WPF_Grid_Example.MainWindow"
Title="MainWindow" Height="350" Width="525" Loaded="Window_Loaded_1">
<Window.Resources>
<CollectionViewSource x:Key="loanViewSource" d:DesignSource="{d:DesignInstance {x:Type WinForms_DataBinding_Example:Loan}, CreateList=True}"/>
</Window.Resources>
<Grid>
<Grid x:Name="grid1" DataContext="{StaticResource loanViewSource}" HorizontalAlignment="Left" Margin="121,123,0,0" VerticalAlignment="Top">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto"/>
<ColumnDefinition Width="Auto"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Label Content="Amount:" Grid.Column="0" HorizontalAlignment="Left" Margin="3" Grid.Row="0" VerticalAlignment="Center"/>
<TextBox x:Name="amountTextBox" Grid.Column="1" HorizontalAlignment="Left" Height="23" Margin="3" Grid.Row="0" Text="{Binding Amount, Mode=TwoWay, NotifyOnValidationError=true, ValidatesOnExceptions=true}" VerticalAlignment="Center" Width="120"/>
<Label Content="Rate:" Grid.Column="0" HorizontalAlignment="Left" Margin="3" Grid.Row="1" VerticalAlignment="Center"/>
<TextBox x:Name="rateTextBox" Grid.Column="1" HorizontalAlignment="Left" Height="23" Margin="3" Grid.Row="1" Text="{Binding Rate, Mode=TwoWay, NotifyOnValidationError=true, ValidatesOnExceptions=true}" VerticalAlignment="Center" Width="120"/>
<Label Content="Total:" Grid.Column="0" HorizontalAlignment="Left" Margin="3" Grid.Row="2" VerticalAlignment="Center"/>
<TextBox x:Name="totalTextBox" Grid.Column="1" HorizontalAlignment="Left" Height="23" Margin="3" Grid.Row="2" Text="{Binding Total, Mode=OneWay}" VerticalAlignment="Center" Width="120"/>
</Grid>
</Grid>
</Window>
问题2:我之前曾被WinForms的黑魔法困扰过,现在同样的黑魔法在WPF中不起作用了,为什么?
问题3:如何使WPF版本自动更新Total
字段,就像WinForms示例中那样?
问题4:对于这种类型的企业应用程序开发,哪个平台更好/更快?如果我要为WPF进行更好的辩论,我应该关注什么?
我希望我对问题有清晰的表述。如果需要任何澄清,请告诉我。谢谢。