我正在使用C# Winform中的Selenium Firefox WebDrivers,我有以下代码以获取单击“webtraffic_popup_start_button”时显示的弹出窗口的句柄,但是它应该获取弹出窗口的句柄,但弹出窗口的句柄与当前句柄相同。
string current = driver.CurrentWindowHandle;
driver.FindElement(By.XPath("//*[@id='webtraffic_popup_start_button']")).Click();
Thread.Sleep(Sleep_Seconds);
popup = driver.CurrentWindowHandle;
Thread.Sleep(3000);
driver.SwitchTo().Window(current);
Thread.Sleep(1000);
任何帮助都将不胜感激,谢谢。
这就是弹出窗口的样子。
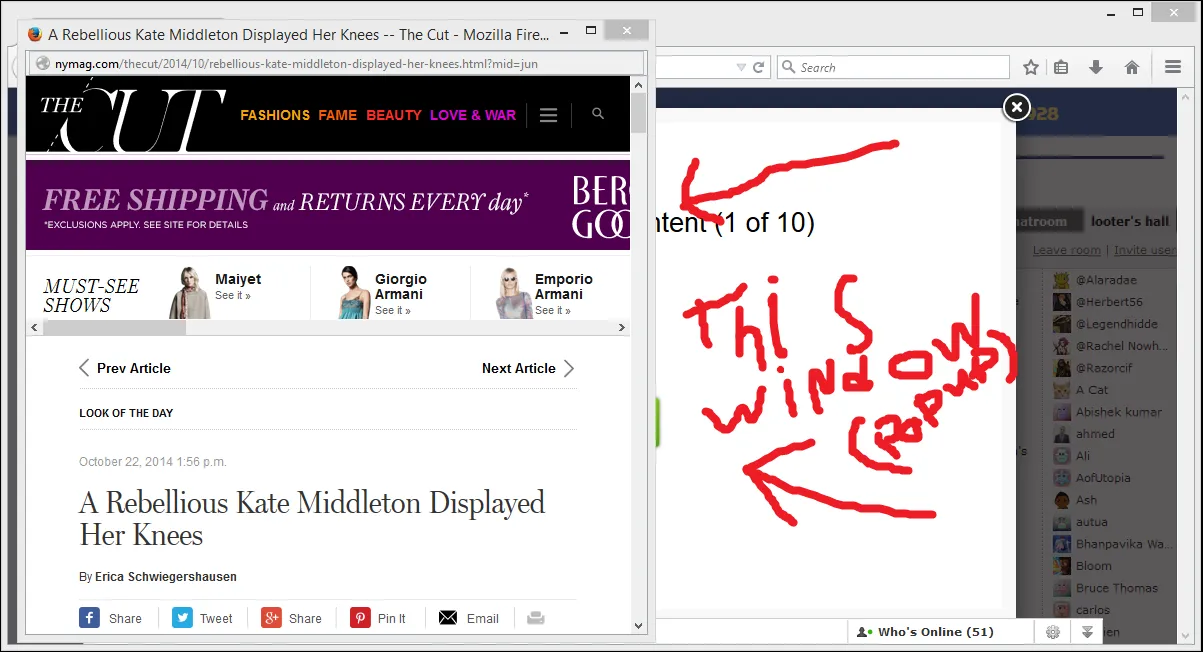