我有一个包含多个片段的应用程序,当单击按钮时,我试图从一个片段转到另一个片段。
我遇到了麻烦的部分是startActivity(新的Intent(HomeFragment.this,FindPeopleFragment.class))
。
package info.androidhive.slidingmenu;
import info.androidhive.slidingmenu.HomeFragment;
import android.app.Fragment;
import android.content.Intent;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageButton;
import android.widget.Toast;
public class HomeFragment extends Fragment {
public HomeFragment() {
}
ImageButton bSearchByLocation, bSearchByNumber;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
super.onCreateView(inflater, container, savedInstanceState);
View InputFragmentView = inflater.inflate(R.layout.fragment_home,
container, false);
bSearchByNumber = ((ImageButton) InputFragmentView
.findViewById(R.id.bSearchByLocation));
bSearchByLocation = ((ImageButton) InputFragmentView
.findViewById(R.id.bSearchByNumber));
bSearchByLocation.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (v.getId() == R.id.bSearchByNumber) {
Toast.makeText(getActivity(), "1", Toast.LENGTH_SHORT)
.show();
startActivity(new Intent(HomeFragment.this, FindPeopleFragment.class));
}
}
});
bSearchByNumber.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (v.getId() == R.id.bSearchByLocation) {
Toast.makeText(getActivity(), "2", Toast.LENGTH_SHORT)
.show();
}
}
});
return InputFragmentView;
}
}
在我完成这个方案之后,代码看起来像这样:
package info.androidhive.slidingmenu;
import info.androidhive.slidingmenu.HomeFragment;
import android.app.Fragment;
import android.content.Intent;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Toast;
public class HomeFragment extends Fragment {
public HomeFragment() {
}
ImageButton bSearchByLocation, bSearchByNumber;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
super.onCreateView(inflater, container, savedInstanceState);
View InputFragmentView = inflater.inflate(R.layout.fragment_home,
container, false);
bSearchByNumber = ((ImageButton) InputFragmentView
.findViewById(R.id.bSearchByLocation));
bSearchByLocation = ((ImageButton) InputFragmentView
.findViewById(R.id.bSearchByNumber));
bSearchByLocation.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (v.getId() == R.id.bSearchByNumber) {
Toast.makeText(getActivity(), "1", Toast.LENGTH_SHORT)
.show();
startActivity(new Intent(getActivity(), FindPeopleFragment.class));
}
}
});
bSearchByNumber.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (v.getId() == R.id.bSearchByLocation) {
Toast.makeText(getActivity(), "2", Toast.LENGTH_SHORT)
.show();
}
}
});
return InputFragmentView;
}
}
但是当我运行它时,应用程序会崩溃并关闭。 这是我的AndroidManifest代码:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="info.androidhive.slidingmenu"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="11"
android:targetSdkVersion="17" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="info.androidhive.slidingmenu.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name="info.androidhive.slidingmenu.FindPeopleFragment"></activity>
</application>
</manifest>
这是我的聊天记录:
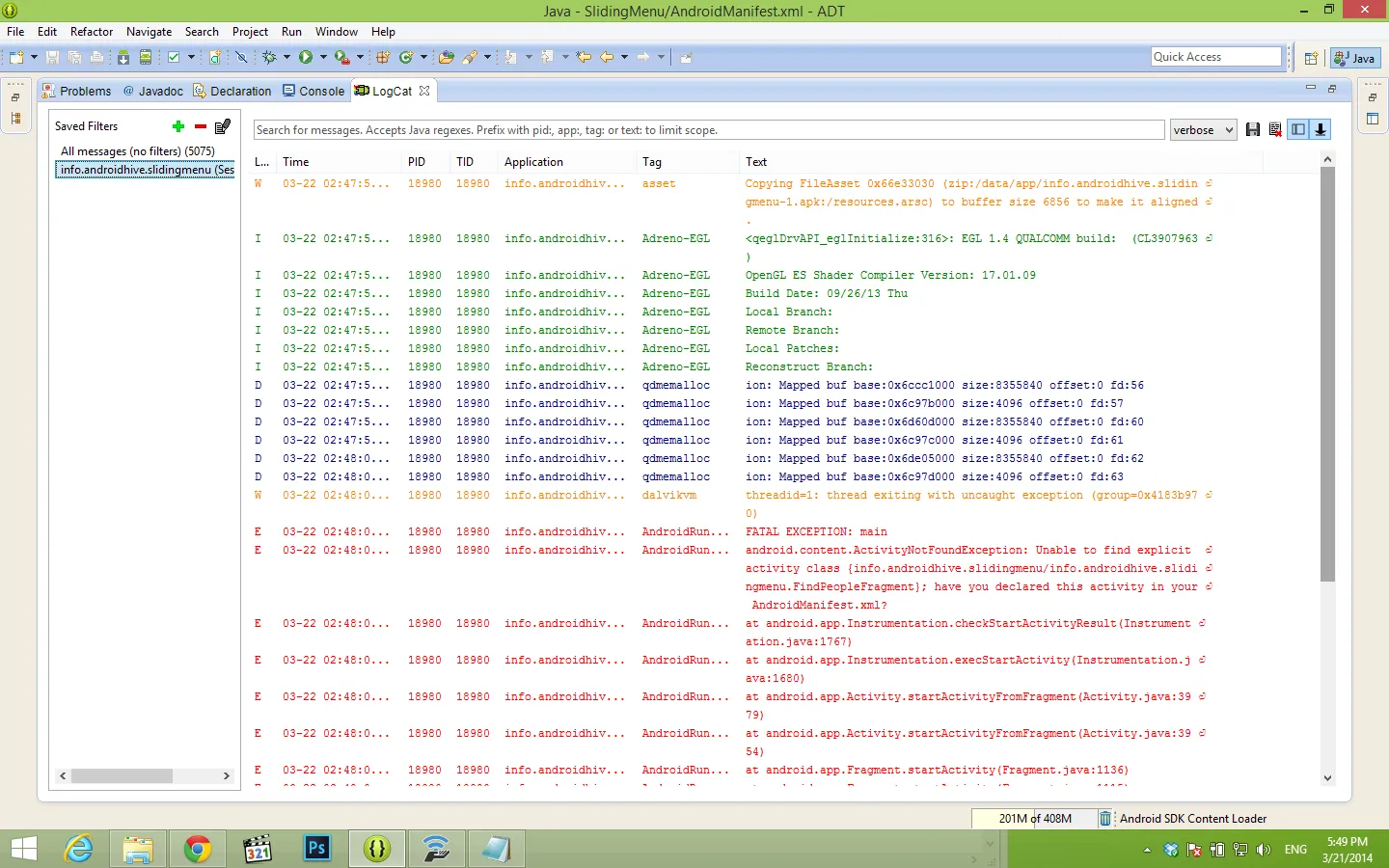
顺便说一下,我使用了这个源代码并对其进行了修改:
http://www.androidhive.info/2013/11/android-sliding-menu-using-navigation-drawer
Fragment
与其父Activity
通信的好方法。在你的“UPDATE”示例代码中,它依赖于Fragment
连接到父级MyFragmentActivity
- 这将防止Fragment
与任何其他类型的Activity
一起使用而不必重写代码。相反,Fragment
应该声明一个回调接口,使用该Fragment
的任何Activity
都应该实现该接口。通过这种方式,Fragment
永远不需要知道它连接到哪种类型的Activity
。 - Squonk