在$group阶段根据条件计算数据,我们可以利用"$accumulator"运算符,在MongoDb 5.0版本中已有所改变。
因此,根据您的需求,我们可以使用以下聚合阶段进行实现 -
db.products.aggregate([
{
$group: {
_id: "$item",
totalCounts: { $sum: 1 },
countsMeta: {
$accumulator: {
init: function () {
return { countSmaller: 0, countBigger: 0 };
},
accumulate: function (state, value) {
return value < 10
? { ...state, countSmaller: state.countSmaller + 1 }
: { ...state, countBigger: state.countBigger + 1 };
},
accumulateArgs: ["$value"],
merge: function (state1, state2) {
return {
countSmaller: state1.countSmaller + state2.countSmaller,
countBigger: state1.countBigger + state2.countBigger,
};
},
finalize: function (state) {
return state;
},
lang: "js",
},
},
},
},
]);
这个执行结果如下所示:
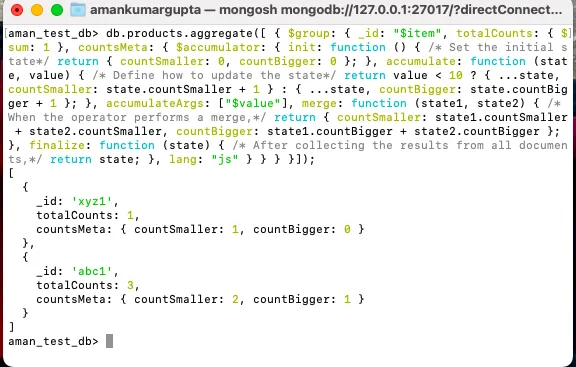
如果需要更多关于阶段和操作符的信息,请参考以下链接:
https://www.mongodb.com/docs/manual/reference/operator/aggregation/accumulator/
希望这能帮到你或其他人。谢谢!
祝编码愉快 :-)
value
字段是一个字符串,因此您可能希望将该键值转换为数字。 - chridamvalue
字段为数字的说明。 我会把这部分留给OP去练习 :) - Anand Jayabalan