我正试图在JavaScript中实现深度优先搜索(DFS),但是我遇到了一些问题。下面是我的Algorithm类:
"use strict";
define([], function () {
return function () {
var that = this;
this.search = function (searchFor, node) {
if (searchFor === node.getValue()) {
return node;
}
var i, children = node.getChildren(), child, found;
for (i = 0; i < children.length; i += 1) {
child = children[i];
found = that.search(searchFor, child);
if (found) {
return found;
}
}
};
};
});
我是一个用来表示图中单个节点的Node类:
"use strict";
define([], function () {
return function (theValue) {
var value = theValue,
children = [];
this.addChild = function (theChild) {
children.push(theChild);
};
this.hasChildren = function () {
return children.length > 0;
};
this.getChildren = function () {
return children;
};
this.getValue = function () {
return value;
};
};
});
我创建了这样一棵树:
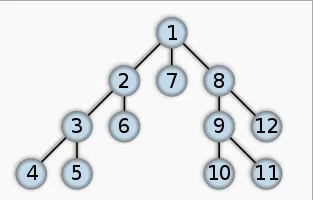
"use strict";
define(["DFS/Node", "DFS/Algorithm"], function (Node, Algorithm) {
return function () {
this.run = function () {
var node1 = new Node(1),
node2 = new Node(2),
node3 = new Node(3),
node4 = new Node(4),
node5 = new Node(5),
node6 = new Node(6),
node7 = new Node(7),
node8 = new Node(8),
node9 = new Node(9),
node10 = new Node(10),
node11 = new Node(11),
node12 = new Node(12),
dfs = new Algorithm();
node1.addChild(node2, node7, node8);
node2.addChild(node3, node6);
node3.addChild(node4, node5);
node8.addChild(node9, node12);
node9.addChild(node10, node11);
console.log(dfs.search(5, node1));
};
};
});
我在日志中看到 undefined。不确定为什么我的代码在4处停止而没有继续执行。
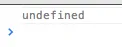
define()
函数来自哪里? - Ibrahim Najjar