使用 Swift 检测带属性文本上的轻拍
对于初学者来说,有时候设置东西可能有些困难(至少我是这样),所以这个示例会更详细一些。
在项目中添加一个 UITextView
。
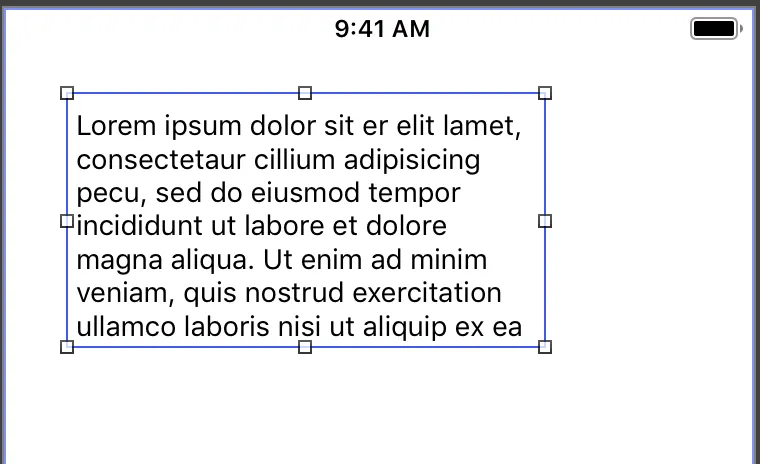
Outlet
使用一个名为 textView
的 outlet 将 UITextView
连接到 ViewController
。
自定义属性
我们将通过创建一个扩展来创建一个自定义属性。
注意:这个步骤技术上是可选的,但是如果你不这么做,下一部分的代码需要使用标准属性,例如 NSAttributedString.Key.foregroundColor
。使用自定义属性的好处在于你可以定义你想要存储在属性文本范围内的值。
使用文件 > 新建 > 文件... > iOS > 源代码 > Swift 文件添加一个新的 Swift 文件。你可以给它取任何你想要的名字。我要把我的命名为 NSAttributedStringKey+CustomAttribute.swift。
将以下代码粘贴到文件中:
import Foundation
extension NSAttributedString.Key {
static let myAttributeName = NSAttributedString.Key(rawValue: "MyCustomAttribute")
}
代码
将ViewController.swift中的代码替换为以下内容。注意 UIGestureRecognizerDelegate
。
import UIKit
class ViewController: UIViewController, UIGestureRecognizerDelegate {
@IBOutlet weak var textView: UITextView!
override func viewDidLoad() {
super.viewDidLoad()
let myString = NSMutableAttributedString(string: "Swift attributed text")
let myRange = NSRange(location: 0, length: 5)
let myCustomAttribute = [ NSAttributedString.Key.myAttributeName: "some value"]
myString.addAttributes(myCustomAttribute, range: myRange)
textView.attributedText = myString
let tap = UITapGestureRecognizer(target: self, action: #selector(myMethodToHandleTap(_:)))
tap.delegate = self
textView.addGestureRecognizer(tap)
}
@objc func myMethodToHandleTap(_ sender: UITapGestureRecognizer) {
let myTextView = sender.view as! UITextView
let layoutManager = myTextView.layoutManager
var location = sender.location(in: myTextView)
location.x -= myTextView.textContainerInset.left;
location.y -= myTextView.textContainerInset.top;
let characterIndex = layoutManager.characterIndex(for: location, in: myTextView.textContainer, fractionOfDistanceBetweenInsertionPoints: nil)
if characterIndex < myTextView.textStorage.length {
print("character index: \(characterIndex)")
let myRange = NSRange(location: characterIndex, length: 1)
let substring = (myTextView.attributedText.string as NSString).substring(with: myRange)
print("character at index: \(substring)")
let attributeName = NSAttributedString.Key.myAttributeName
let attributeValue = myTextView.attributedText?.attribute(attributeName, at: characterIndex, effectiveRange: nil)
if let value = attributeValue {
print("You tapped on \(attributeName.rawValue) and the value is: \(value)")
}
}
}
}
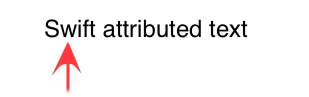
现在,如果您点击“Swift”中的“w”,您应该会得到以下结果:
character index: 1
character at index: w
You tapped on MyCustomAttribute and the value is: some value
笔记
- 在这里,我使用了自定义属性,但它也可以很容易地是颜色为
UIColor.green
的文本颜色NSAttributedString.Key.foregroundColor
。
- 以前,文本视图不能编辑或选择,但在我的Swift 4.2的更新答案中,无论是否选择这些选项,它似乎都能正常工作。
深入学习
这个答案基于这个问题的几个其他答案。除此之外,请参阅: