使用 点到线段的最短距离 中的 dist
函数。
import math
def dist(p1, p2, c):
x1,y1 = p1
x2,y2 = p2
x3,y3 = c
px = x2-x1
py = y2-y1
something = px*px + py*py
u = ((x3 - x1) * px + (y3 - y1) * py) / float(something)
if u > 1:
u = 1
elif u < 0:
u = 0
x = x1 + u * px
y = y1 + u * py
dx = x - x3
dy = y - y3
dist = math.sqrt(dx*dx + dy*dy)
return dist
这里是一个测试:
(保留HTML,不进行解释)
rect = [[0. , 0. ],
[ 0.2, 1. ],
[ 2.2, 0.6],
[ 2. , -0.4]]
c = 0.5, 2.0
r = 1.0
distances = [dist(rect[i], rect[j], c) for i, j in zip([0, 1, 2, 3], [1, 2, 3, 0])]
print distances
print any(d < r for d in distances)
输出:
[1.044030650891055, 1.0394155162323753, 2.202271554554524, 2.0592194189509323]
False
这里是情节:
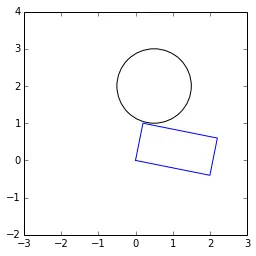